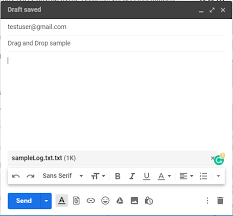
Selenium Js Api
The Selenium Browser Automation Project
Selenium is an umbrella project for a range of tools and libraries
that enable and support the automation of web provides extensions to emulate user interaction with browsers,
a distribution server for scaling browser allocation,
and the infrastructure for implementations of the
W3C WebDriver specification
that lets you write interchangeable code for all major web project is made possible by volunteer contributors
who have put in thousands of hours of their own time,
and made the source code
freely available
for anyone to use, enjoy, and lenium brings together browser vendors, engineers, and enthusiasts
to further an open discussion around automation of the web platform.
The project organises an annual conference
to teach and nurture the the core of Selenium is WebDriver,
an interface to write instruction sets that can be run interchangeably in many
browsers. Here is one of the simplest instructions you can make:JavaPythonCSharpRubyJavaScriptKotlinimport;
import;
import static;
public class HelloSelenium {
public static void main(String[] args) {
WebDriver driver = new FirefoxDriver();
WebDriverWait wait = new WebDriverWait(driver, Duration. ofSeconds(10));
try {
(“);
ndElement((“q”)). sendKeys(“cheese” +);
WebElement firstResult = (presenceOfElementLocated(By. cssSelector(“h3”)));
(tAttribute(“textContent”));} finally {
();}}}
from selenium import webdriver
from import By
from import Keys
from import WebDriverWait
from pport. expected_conditions import presence_of_element_located
#This example requires Selenium WebDriver 3. 13 or newer
with refox() as driver:
wait = WebDriverWait(driver, 10)
(“)
nd_element(, “q”). send_keys(“cheese” +)
first_result = (presence_of_element_located((By. CSS_SELECTOR, “h3”)))
print(t_attribute(“textContent”))
using System;
using lenium;
using refox;
using;
class HelloSelenium {
static void Main() {
using(IWebDriver driver = new FirefoxDriver()) {
WebDriverWait wait = new WebDriverWait(driver, omSeconds(10));
vigate(). GoToUrl(“);
ndElement((“q”)). SendKeys(“cheese” +);
(webDriver => ndElement(By. CssSelector(“h3”)). Displayed);
IWebElement firstResult = ndElement(By. CssSelector(“h3”));
Console. WriteLine(tAttribute(“textContent”));}}}
require ‘selenium-webdriver’
driver =:firefox
wait = (timeout: 10)
begin
”
nd_element(name: ‘q’). send_keys ‘cheese’, :return
first_result = { nd_element(css: ‘h3’)}
puts tribute(‘textContent’)
ensure
end
const {Builder, By, Key, until} = require(‘selenium-webdriver’);
(async function example() {
let driver = await new Builder(). forBrowser(‘firefox’)();
// Navigate to Url
await (”);
// Enter text “cheese” and perform keyboard action “Enter”
await ndElement((‘q’)). sendKeys(‘cheese’, );
let firstResult = await (until. elementLocated((‘h3’)), 10000);
(await tAttribute(‘textContent’));}
finally{
await ();}})();
import
fun main() {
val driver = FirefoxDriver()
val wait = WebDriverWait(driver, Duration. ofSeconds(10))
ndElement((“q”)). sendKeys(“cheese” +)
val firstResult = (presenceOfElementLocated(By. cssSelector(“h3”)))
println(tAttribute(“textContent”))} finally {
()}}
See the Overview to check the different project
components and decide if Selenium is the right tool for should continue on to Getting Started
to understand how you can install Selenium and successfully use it as a test
automation tool, and scaling simple tests like this to run in large, distributed
environments on multiple browsers, on several different operating systems. OverviewIs Selenium for you? See an overview of the different project tting StartedIf you are new to Selenium, we have a few resources that can help you get up to speed right away. WebDriverWebDriver drives a browser natively, learn more about idelines and recommendationsSome guidelines and recommendations on testing from the Selenium practicesThings to avoid when automating browsers with idWant to run tests in parallel across multiple machines? Then, Grid is for pport PackagesSet of packages and functionalities to simplify automation with Selenium. LegacyDocumentation related to the legacy components of Selenium. Meant to be kept purely for historical reasons and not as a incentive to use deprecated components.
Where is the documentation of Selenium Webdriver for NodeJS?
I don’t seem to find any, the only one I always find is this one:
But it is more a guide. Not a documentation of all functions. It lacks for example the documentation for, or something like getWindowHandles()
asked May 19 ’15 at 10:34
JustGoschaJustGoscha23. 7k14 gold badges47 silver badges61 bronze badges
2
I’m having the same problem and there is almost no proper examples out there as gar as I know. Please share, if you found something!
Feb 26 ’16 at 7:26
This question has a large view count and serves a purpose – this piece of documentation is rather hidden
Feb 25 ’19 at 17:39
WebDriverJs · SeleniumHQ/selenium Wiki – GitHub
Introduction
The WebDriverJS library uses a promise manager to ease the pain of working with a purely asynchronous API. Rather than writing a long chain of promises, the promise manager allows you to write code as if WebDriverJS had a synchronous, blocking API (like all of the other Selenium language bindings). For instance, instead of
const {Builder, By, until} = require(‘selenium-webdriver’);
new Builder(). forBrowser(‘firefox’)
()
(driver => {
return (”)
(_ => ndElement((‘q’)). sendKeys(‘webdriver’))
(_ => ndElement((‘btnK’))())
(_ => (until. titleIs(‘webdriver – Google Search’), 1000))
(_ => ());});
you can write
let driver = new Builder(). forBrowser(‘firefox’)
();
(”);
ndElement((‘q’)). sendKeys(‘webdriver’);
ndElement((‘btnK’))();
(until. titleIs(‘webdriver – Google Search’), 1000);
Unforutnately, there is no such thing as a free lunch. With WebDriverJS, using the promise manager comes at the cost of increased complexity (for the Selenium maintainers–the promise module is a 3300 line beast) and reduced debuggability. For debugging, suppose you inserted a debugger statement:
debugger;
When is this script going to pause – after WebDriver loads, or after it schedules the command to load Since the promise manager abstracts away the async nature of the API, it hides that you need to expicitly use a callback to break after a command executes:
(”)(() => debugger);
JavaScript has evolved in many ways since WebDriverJS was originally created. Not only did the community standardize the behavior and API for promises, but promises were added to the language itself. The next version of JavaScript, ES2017, adds support for async functions, greatly simplyfing the process of writing and maintaining asynchronous code. At this point, the benefits of the promise manager no longer outweigh its costs, so it will soon be deprecated and removed from WebDriverJS. The remainder of this guide will explain how users can migrate off the promise manager and effectively use the async constructs available today.
Moving to async/await
Step 1: Disabling the Promise Manager
As outlined in issue 2969, WebDriverJS’ promise manager will be deprecated and disabled by default with the first Node LTS release that includes native support for async functions. This feature is currently available in Node 7. x, hidden behind the –harmony_async_await flag.
Instead of waiting for the LTS, you can disable the promise manager today either by setting an environment variable, SELENIUM_PROMISE_MANAGER=0, or through the promise module’s API (E_PROMISE_MANAGER = false).
When the promise manager is disabled, any attempt to create a managed promise will generate an error, so expect your scripts to fail the first time you try running with the promise manager.
Step 2: Migrate Direct Usages of Managed Promises
Search your code for every instance where you create a omise object, either using the constructor, or the resolve/reject factories. Replace these calls with the equivalent listed in the table below. These functions will automatically switch from managed to native promises when the promise manager is disabled.
Original
Replacement
new omise()
eatePromise()
solve()
promise. fulfilled()
jected()
Step 3: Migrate Off of the Promise Manager
At this point, you should be ready to migrate off of the promise manager. Unfortunately, there’s no automated way to update your code; you basically have to disable the promise manager, see what fails, update some code, and try again. To understand how your code will, fail, consider a test for our Google Search example:
const test = require(‘selenium-webdriver/testing’);
describe(‘Google Search’, function() {
let driver;
(function() {
driver = new Builder(). forBrowser(‘firefox’)();});
(‘example’, function theTestFunction() {
(”); // (1)
ndElement((‘q’)). sendKeys(‘webdriver’); // (2)
ndElement((‘btnK’))(); // (3)
(until. titleIs(‘webdriver – Google Search’), 1000); // (4)});
();});});
Inside theTestFunction, when the promise manager is enabled, it will capture every WebDriver command and block its execution until those before it have completed. Thus, even though ndElement() on line (2) is immediately scheduled, it will not start execution until the command on line (1) completes.
When you disable the promise manager, every command will start executing as soon as its scheduled. The exact behavior depends on the specific browser, but essentially, the command to find an element on line (2) will start executing before the page requested on line (1) has loaded.
You will need to update your code to explicitly link each action so it does not start until previous commands have finished. Presented below are three options for how to update your code.
Option 1: Use classic promise chaining
Your first option is to adopt classic promise chaining (yes, the very thing the promise manager was created to avoid). This will make your code far more verbose, but it will work with and without the promise manager and you won’t need to use the selenium-webdriver/testing module for mocha-based tests.
before(function() {
return new Builder(). forBrowser(‘firefox’)()(d => {
driver = d;});});
it(‘example’, function theTestFunction() {
(_ => ndElement((‘q’)))
(q => ndKeys(‘webdriver’))
(_ => ndElement((‘btnK’)))
(b => ())
(_ => (until. titleIs(‘webdriver – Google Search’), 1000));});
after(function() {
return ();});});
Option 2: Migrate to Generators
Your second option is to update your code to use asynchronouse generator functions. The selenium-webdriver/testing module already handles these out of the box. You can also use third-party libraries like for the same effect. Basically, change each of your test functions to a generator, and yield on a promise. The generator wrapper will transparently wait for the promise to resolve before resuming the function. It’s important to note, however, asynchronous generators are not currently supported natively in JavaScript, so you will have to use selenium-webdriver or another library for this to work.
(function*() {
driver = yield new Builder(). forBrowser(‘firefox’)();});
(‘example’, function* theTestFunction() {
yield (”); // (1)
yield ndElement((‘q’)). sendKeys(‘webdriver’); // (2)
yield ndElement((‘btnK’))(); // (3)
yield (until. titleIs(‘webdriver – Google Search’), 1000); // (4)});
yield ();});});
The advantage to using generators with selenium-webdriver/testing is your code will work with and without the promise manager, so you can convert one test at a time. Another advantage to this approach is your code will work today with Node 6 & 7. When async/await support is added to Node (it’s currently hidden behind a flag in Node 7), you can migrate from generators with find-and-replace, converting function*() to async function() and yield to await.
The selenium-webdriver/example directory contains an example of our Google Search test written with and without generators so you can compare the two side-by-side.
Option 3: Migrate to async/await
Your final option is to switch to async/await. As mentioned above, these language features are currently hidden behind a flag in Node 7, so you will have to run with –harmony_async_await or you will have to transpile your code with Babel (setting up Babel is left as an exercise for the reader).
Compared to generators, there is one more catch to using async/await: they do not play well with the promise manager, so you must ensure the promise manager is disabled. There is a complete working example of a test written using async/await provided in the selenium-webdriver/example directory:
E_PROMISE_MANAGER = false;
beforeEach(async function() {
driver = await new Builder(). forBrowser(‘firefox’)();});
afterEach(async function() {
await ();});
it(‘example’, async function() {
await (”);
await ndElement((‘q’)). sendKeys(‘webdriver’);
await ndElement((‘btnK’))();
await (until. titleIs(‘webdriver – Google Search’), 1000);});});
This example disables the promise manager globally. In order to migrate tests bit-by-bit, you can selectively disable the promise manager in before/after blocks:
function legacySuite(name, fn) {
describe(name, function() {
before(() => E_PROMISE_MANAGER = true);
after(() => E_PROMISE_MANAGER = false);
fn();});}
describe(‘Example’, function() {
legacySuite(‘legacy tests’, function() {
(‘test 1’, function() {
//… });});
it(‘test 2’, async function() {
Miscellaneous Tips
Use Logging To Find Unmigrated Code
While the promise manager can be easily toggled through an enviornment variable, constantly running your code in the two modes can be tedious. Selenium’s promise module provides logging to report every instance of unsynchronized code running through the promise manager (which, in code, is actually called the ControlFlow). You can enable this logging, then work through the messages to update each instance of commands that have not be properly chained (depending on the option you chose above).
Enabling logging is only two extra lines:
const {Builder, By, logging, until} = require(‘selenium-webdriver’);
stallConsoleHandler();
tLogger(‘ntrolFlow’). setLevel();
With logging, the example above will produce messages like:
[2017-03-06T02:18:14Z] [WARNING] [ntrolFlow] Detected scheduling of an unchained task.
When the promise manager is disabled, unchained tasks will not wait for
previously scheduled tasks to finish before starting to execute.
New task: Task: vigate()()
at hedule (/Users/jleyba/Development/test/node_modules/selenium-webdriver/lib/)
at (/Users/jleyba/Development/test/node_modules/selenium-webdriver/lib/)
at Object.
at Module. _compile ()
at ()
at tryModuleLoad ()
at nMain ()
Previous task: Task: eateSession()
at eateSession (/Users/jleyba/Development/test/node_modules/selenium-webdriver/lib/)
at eateSession (/Users/jleyba/Development/test/node_modules/selenium-webdriver/firefox/)
at createDriver (/Users/jleyba/Development/test/node_modules/selenium-webdriver/)
at (/Users/jleyba/Development/test/node_modules/selenium-webdriver/)
at ()