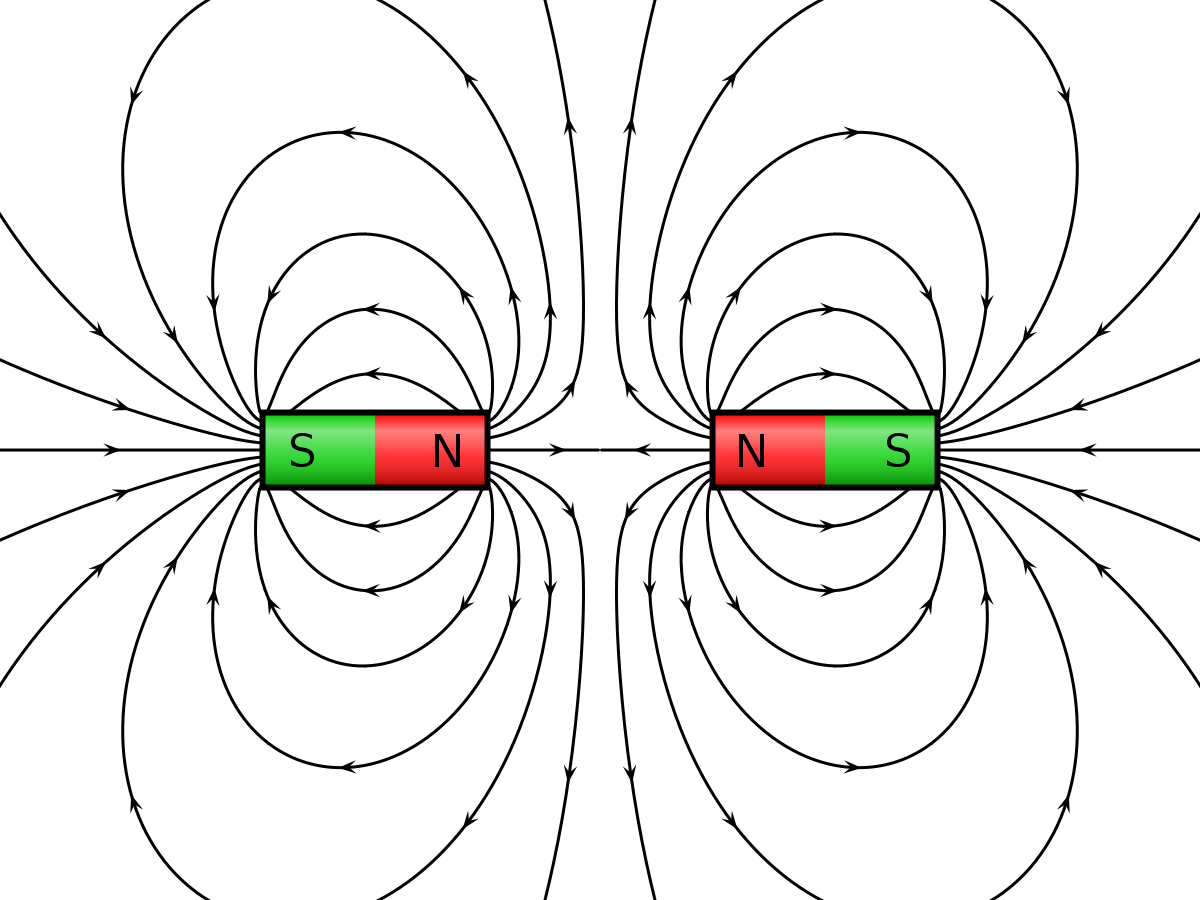
Python Parse Html File
html.parser — Simple HTML and XHTML parser — Python …
Source code: Lib/html/
This module defines a class HTMLParser which serves as the basis for
parsing text files formatted in HTML (HyperText Mark-up Language) and XHTML.
class (*, convert_charrefs=True)¶
Create a parser instance able to parse invalid markup.
If convert_charrefs is True (the default), all character
references (except the ones in script/style elements) are
automatically converted to the corresponding Unicode characters.
An HTMLParser instance is fed HTML data and calls handler methods
when start tags, end tags, text, comments, and other markup elements are
encountered. The user should subclass HTMLParser and override its
methods to implement the desired behavior.
This parser does not check that end tags match start tags or call the end-tag
handler for elements which are closed implicitly by closing an outer element.
Changed in version 3. 4: convert_charrefs keyword argument added.
Changed in version 3. 5: The default value for argument convert_charrefs is now True.
Example HTML Parser Application¶
As a basic example, below is a simple HTML parser that uses the
HTMLParser class to print out start tags, end tags, and data
as they are encountered:
from import HTMLParser
class MyHTMLParser(HTMLParser):
def handle_starttag(self, tag, attrs):
print(“Encountered a start tag:”, tag)
def handle_endtag(self, tag):
print(“Encountered an end tag:”, tag)
def handle_data(self, data):
print(“Encountered some data:”, data)
parser = MyHTMLParser()
(‘
‘
Parse me!
‘)
The output will then be:
Encountered a start tag: html
Encountered a start tag: head
Encountered a start tag: title
Encountered some data: Test
Encountered an end tag: title
Encountered an end tag: head
Encountered a start tag: body
Encountered a start tag: h1
Encountered some data: Parse me!
Encountered an end tag: h1
Encountered an end tag: body
Encountered an end tag: html
HTMLParser Methods¶
HTMLParser instances have the following methods:
(data)¶
Feed some text to the parser. It is processed insofar as it consists of
complete elements; incomplete data is buffered until more data is fed or
close() is called. data must be str.
()¶
Force processing of all buffered data as if it were followed by an end-of-file
mark. This method may be redefined by a derived class to define additional
processing at the end of the input, but the redefined version should always call
the HTMLParser base class method close().
Reset the instance. Loses all unprocessed data. This is called implicitly at
instantiation time.
Return current line number and offset.
t_starttag_text()¶
Return the text of the most recently opened start tag. This should not normally
be needed for structured processing, but may be useful in dealing with HTML “as
deployed” or for re-generating input with minimal changes (whitespace between
attributes can be preserved, etc. ).
The following methods are called when data or markup elements are encountered
and they are meant to be overridden in a subclass. The base class
implementations do nothing (except for handle_startendtag()):
HTMLParser. handle_starttag(tag, attrs)¶
This method is called to handle the start of a tag (e. g.
The tag argument is the name of the tag converted to lower case. The attrs
argument is a list of (name, value) pairs containing the attributes found
inside the tag’s <> brackets. The name will be translated to lower case,
and quotes in the value have been removed, and character and entity references
have been replaced.
For instance, for the tag ‘)
Decl: DOCTYPE HTML PUBLIC “-//W3C//DTD HTML 4. 01//EN” ”
Parsing an element with a few attributes and a title:
>>> (‘
Start tag: img
attr: (‘src’, ”)
attr: (‘alt’, ‘The Python logo’)
>>>
>>> (‘
Python
‘)
Start tag: h1
Data: Python
End tag: h1
The content of script and style elements is returned as is, without
further parsing:
>>> (‘
‘)
Start tag: style
attr: (‘type’, ‘text/css’)
Data: #python { color: green}
End tag: style
>>> (‘‘)
Start tag: script
attr: (‘type’, ‘text/javascript’)
Data: alert(“hello! “);
End tag: script
Parsing comments:
>>> (‘‘… ‘IE-specific content‘)
Comment: a comment
Comment: [if IE 9]>IE-specific content‘):
>>> (‘>>>’)
Named ent: >
Num ent: >
Feeding incomplete chunks to feed() works, but
handle_data() might be called more than once
(unless convert_charrefs is set to True):
>>> for chunk in [‘
Start tag: span
Data: buff
Data: ered
Data: text
End tag: span
Parsing invalid HTML (e. unquoted attributes) also works:
>>> (‘
Start tag: p
Start tag: a
attr: (‘class’, ‘link’)
attr: (‘href’, ‘#main’)
Data: tag soup
End tag: p
End tag: a
How to parse local HTML file in Python? – GeeksforGeeks
Prerequisites: BeautifulsoupParsing means dividing a file or input into pieces of information/data that can be stored for our personal use in the future. Sometimes, we need data from an existing file stored on our computers, parsing technique can be used in such cases. The parsing includes multiple techniques used to extract data from a file. The following includes Modifying the file, Removing something from the file, Printing data, using the recursive child generator method to traverse data from the file, finding the children of tags, web scraping from a link to extract useful information, etc. Attention geek! Strengthen your foundations with the Python Programming Foundation Course and learn the basics. To begin with, your interview preparations Enhance your Data Structures concepts with the Python DS Course. And to begin with your Machine Learning Journey, join the Machine Learning – Basic Level CourseModifying the fileUsing the prettify method to modify the HTML code from-, look better. Prettify makes the code look in the standard form like the one used in VS Code. Example:Output:Removing a tagA tag can be removed by using the decompose method and the select_one method with the CSS selectors to select and then remove the second element from the li tag and then using the prettify method to modify the HTML code from the file. Example:File Used:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()S = BeautifulSoup(index, ‘lxml’)Tag = lect_one(‘li:nth-of-type(2)’)compose()print(())Output:Finding tagsTags can be found normally and printed normally using print(). Example:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()Parse = BeautifulSoup(index, ‘lxml’)print()print(Parse. h1)print(Parse. h2)print(Parse. h3)print()Output:Traversing tagsThe recursiveChildGenerator method is used to traverse tags, which recursively finds all the tags within tags from the file. Example:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()S = BeautifulSoup(index, ‘lxml’)for TraverseTags in cursiveChildGenerator(): if print()Output:Parsing name and text attributes of tags Using the name attribute of the tag to print its name and the text attribute to print its text along with the code of the tag- ul from the file. Example:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()S = BeautifulSoup(index, ‘lxml’)print(f’HTML: {}, name: {}, text: {}’)Output:Finding Children of a tag The Children attribute is used to get the children of a tag. The Children attribute returns ‘tags with spaces’ between them, we’re adding a condition- e. name is not None to print only names of the tags from the file. Example:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()S = BeautifulSoup(index, ‘lxml’)Attr = mlAttr_Tag = [ for e in ildren if is not None]print(Attr_Tag)Output:Finding Children at all levels of a tag:The Descendants attribute is used to get all the descendants (Children at all levels) of a tag from the file. Example:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()S = BeautifulSoup(index, ‘lxml’)Des = dyAttr_Tag = [ for e in scendants if is not None]print(Attr_Tag)Output:Finding all elements of tags Using find_all():The find_all method is used to find all the elements (name and text) inside the p tag from the file. Example:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()S = BeautifulSoup(index, ‘lxml’)for tag in nd_all(‘p’): print(f'{}: {}’)Output:CSS selectors to find elements: Using the select method to use the CSS selectors to find the second element from the li tag from the file. Example:Python3from bs4 import BeautifulSoupHTMLFile = open(“”, “r”)index = ()S = BeautifulSoup(index, ‘lxml’)print((‘li:nth-of-type(2)’))Output: Attention geek! Strengthen your foundations with the Python Programming Foundation Course and learn the basics.
Parsing HTML using Python – Stack Overflow
I’m looking for an HTML Parser module for Python that can help me get the tags in the form of Python lists/dictionaries/objects.
If I have a document of the form:
then it should give me a way to access the nested tags via the name or id of the HTML tag so that I can basically ask it to get me the content/text in the div tag with class=’container’ contained within the body tag, or something similar.
If you’ve used Firefox’s “Inspect element” feature (view HTML) you would know that it gives you all the tags in a nice nested manner like a tree.
I’d prefer a built-in module but that might be asking a little too much.
I went through a lot of questions on Stack Overflow and a few blogs on the internet and most of them suggest BeautifulSoup or lxml or HTMLParser but few of these detail the functionality and simply end as a debate over which one is faster/more efficent.
the Tin Man152k39 gold badges201 silver badges282 bronze badges
asked Jul 29 ’12 at 12:00
1
So that I can ask it to get me the content/text in the div tag with class=’container’ contained within the body tag, Or something similar.
try:
from BeautifulSoup import BeautifulSoup
except ImportError:
from bs4 import BeautifulSoup
html = #the HTML code you’ve written above
parsed_html = BeautifulSoup(html)
print((‘div’, attrs={‘class’:’container’}))
You don’t need performance descriptions I guess – just read how BeautifulSoup works. Look at its official documentation.
Edward6252 gold badges10 silver badges29 bronze badges
answered Jul 29 ’12 at 12:12
AadaamAadaam3, 1591 gold badge12 silver badges9 bronze badges
9
I guess what you’re looking for is pyquery:
pyquery: a jquery-like library for python.
An example of what you want may be like:
from pyquery import PyQuery
html = # Your HTML CODE
pq = PyQuery(html)
tag = pq(‘div#id’) # or tag = pq(”)
print ()
And it uses the same selectors as Firefox’s or Chrome’s inspect element. For example:
The inspected element selector is ‘print’. So in pyquery, you just need to pass this selector:
pq(‘print’)
chris Frisina17. 9k20 gold badges75 silver badges157 bronze badges
answered Jul 29 ’12 at 12:47
YusuMishiYusuMishi2, 15716 silver badges7 bronze badges
Here you can read more about different HTML parsers in Python and their performance. Even though the article is a bit dated it still gives you a good overview.
Python HTML parser performance
I’d recommend BeautifulSoup even though it isn’t built in. Just because it’s so easy to work with for those kinds of tasks. Eg:
import urllib2
page = urllib2. urlopen(”)
soup = BeautifulSoup(page)
x = (‘div’, attrs={‘class’: ‘container’})
Matt Ellen9, 9834 gold badges62 silver badges81 bronze badges
answered Jul 29 ’12 at 12:07
QiauQiau5, 1283 gold badges27 silver badges40 bronze badges
3
I recommend lxml for parsing HTML. See “Parsing HTML” (on the lxml site).
In my experience Beautiful Soup messes up on some complex HTML. I believe that is because Beautiful Soup is not a parser, rather a very good string analyzer.
answered Oct 25 ’14 at 18:50
2
I recommend using justext library:
Usage:
Python2:
import requests
import justext
response = (“)
paragraphs = justext. justext(ntent, t_stoplist(“English”))
for paragraph in paragraphs:
print
Python3:
answered Jul 15 ’16 at 15:51
Wesam NaWesam Na1, 75922 silver badges20 bronze badges
I would use EHP
Here it is:
from ehp import *
doc = ”’
”’
html = Html()
dom = (doc)
for ind in (‘div’, (‘class’, ‘container’)):
Output:
Something here
Something else
answered Mar 20 ’16 at 9:44
Not the answer you’re looking for? Browse other questions tagged python xml-parsing html-parsing or ask your own question.
Frequently Asked Questions about python parse html file
How do I parse HTML in Python?
Examplefrom html. parser import HTMLParser.class Parser(HTMLParser):# method to append the start tag to the list start_tags.def handle_starttag(self, tag, attrs):global start_tags.start_tags. append(tag)# method to append the end tag to the list end_tags.def handle_endtag(self, tag):More items…
How do you scrape an HTML file in Python?
To extract data using web scraping with python, you need to follow these basic steps:Find the URL that you want to scrape.Inspecting the Page.Find the data you want to extract.Write the code.Run the code and extract the data.Store the data in the required format.Sep 24, 2021
Can Python read HTML file?
Opening an HTML file in Python allows the program to interact with the file. Once opened, the contents of the HTML file may be read or written to.