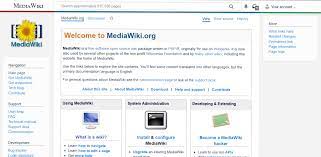
Php Proxy Tutorial
jenssegers/php-proxy – GitHub
This is a HTTP/HTTPS proxy script that forwards requests to a different server and returns the response. The Proxy class uses PSR7 request/response objects as input/output, and uses Guzzle to do the actual HTTP request.
Installation
Install using composer:
composer require jenssegers/proxy
Example
The following example creates a request object, based on the current browser request, and forwards it to The RemoveEncodingFilter removes the encoding headers from the original response so that the current webserver can set these correctly.
use Proxy\Proxy;
use Proxy\Adapter\Guzzle\GuzzleAdapter;
use Proxy\Filter\RemoveEncodingFilter;
use Laminas\Diactoros\ServerRequestFactory;
// Create a PSR7 request based on the current browser request.
$request = ServerRequestFactory::fromGlobals();
// Create a guzzle client
$guzzle = new GuzzleHttp\Client();
// Create the proxy instance
$proxy = new Proxy(new GuzzleAdapter($guzzle));
// Add a response filter that removes the encoding headers.
$proxy->filter(new RemoveEncodingFilter());
try {
// Forward the request and get the response.
$response = $proxy->forward($request)->to(”);
// Output response to the browser.
(new Laminas\HttpHandlerRunner\Emitter\SapiEmitter)->emit($response);} catch(\GuzzleHttp\Exception\BadResponseException $e) {
// Correct way to handle bad responses
(new Laminas\HttpHandlerRunner\Emitter\SapiEmitter)->emit($e->getResponse());}
Filters
You can apply filters to the requests and responses using the middleware strategy:
$response = $proxy
->forward($request)
->filter(function ($request, $response, $next) {
// Manipulate the request object.
$request = $request->withHeader(‘User-Agent’, ‘FishBot/1. 0’);
// Call the next item in the middleware.
$response = $next($request, $response);
// Manipulate the response object.
$response = $response->withHeader(‘X-Proxy-Foo’, ‘Bar’);
return $response;})
->to(”);
Building A Simple API Proxy Server with PHP – DZone Web Dev
Join the DZone community and get the full member experience.
Join For Free
these days i’m playing with backbone and using public api as a source. the web browser has one horrible feature: it don’t allow you to fetch any external resource to our host due to the cross-origin restriction. for example if we have a server at localhost we cannot perform one
ajax request to another host different than localhost. nowadays there is
a header to allow it:
access-control-allow-origin.
the problem is that the remote server must set up this header. for
example i was playing with github’s api and github doesn’t have this
header. if the server is my server, is pretty straightforward to put
this header but obviously i’m not the sysadmin of github, so i cannot do
it. what the solution? one possible solution is, for example, create a
proxy server at localhost with php. with php we can use any remote api
with curl (i wrote about it
here
and
for example). it’s not difficult, but i asked myself: can we create a
dummy proxy server with php to handle any request to localhost and
redirects to the real server, instead of create one proxy for each
request?. let’s start. problably there is one open source solution (tell
me if you know it) but i’m on holidays and i want to code a little bit
(i now, it looks insane but that’s me).
the idea is:…
$proxy->register(‘github’, ”);…
and when i type:
localhost/github/users/gonzalo123
and create a proxy to:
the request method is also important. if we create a post request to localhost we want a post request to github too.
this time we’re not going to reinvent the wheel, so we will use symfony componets so we will use composer to start our project:
we create a file with the dependencies:
{
“require”: {
“symfony/class-loader”:”dev-master”,
“symfony/-foundation”:”dev-master”}}
now
php install
and we can start coding. the script will look like this:
register(‘github’, ”);
$proxy->run();
foreach($proxy->getheaders() as $header) {
header($header);}
echo $proxy->getcontent();
as we can see we can register as many servers as we want. in this
example we only register github. the application only has two classes:
restproxy, who extracts the information from the request object and calls to the real server through
curlwrapper.
php
namespace restproxy;
class restproxy
private $request;
private $curl;
private $map;
private $content;
private $headers;
public function __construct(\symfony\component\foundation\request $request, curlwrapper $curl)
$this->request = $request;
$this->curl = $curl;}
public function register($name, $url)
$this->map[$name] = $url;}
public function run()
foreach ($this->map as $name => $mapurl) {
return $this->dispatch($name, $mapurl);}}
private function dispatch($name, $mapurl)
$url = $this->request->getpathinfo();
if (strpos($url, $name) == 1) {
$url = $mapurl. str_replace(“/{$name}”, null, $url);
$querystring = $this->request->getquerystring();
switch ($this->request->getmethod()) {
case ‘get’:
$this->content = $this->curl->doget($url, $querystring);
break;
case ‘post’:
$this->content = $this->curl->dopost($url, $querystring);
case ‘delete’:
$this->content = $this->curl->dodelete($url, $querystring);
case ‘put’:
$this->content = $this->curl->doput($url, $querystring);
break;}
$this->headers = $this->curl->getheaders();}}
public function getheaders()
return $this->headers;}
public function getcontent()
return $this->content;}}
the restproxy receive two instances in the constructor via dependency
injection (curlwrapper and request). this architecture helps a lot in
the
tests, because we can mock both instances. very helpfully when building restproxy.
the restproxy is registerd within
packaist
so we can install it using composer installer:
first install componser
curl -s | php
and create a new project:
php create-project gonzalo123/rest-proxy proxy
if we are using php5. 4 (if not, what are you waiting for? ) we can run the build-in server
cd proxy
php -s localhost:8888 -t www/
now we only need to open a web browser and type:
localhost:8888/github/users/gonzalo123
the library is very minimal (it’s enough for my experiment) and it does’t allow authorization.
of course full code is available in
github.
Opinions expressed by DZone contributors are their own.
Setup your own proxy website using PHP-Proxy – Hyperbyte
(Last Updated On: September 8, 2021)Setting up your own web proxy website can keep you floating even when most of the internet unblocking services will not work. Access to a web proxy appears to your Internet service provider as a normal web traffic. To setup it, the first thing to decide is whether you want to setup your web proxy on a shared hosting plan or do you plan to establish your website on a dedicated server or a virtual private server. If you want to setup it over a shared hosting plan it will only serve you personally and not many people can use it simultaneously. Shared hosting is usually very limited in terms of system resources but they are best for beginners. Hosting providers have great tutorials and also offer extensive support that are mostly included with the packages.
Prerequisites
You need a domain name, you will need to purchase one from a domain registrar like namecheap. If you are looking to just play and test things out, you can get free domains from Freenom. You will need a shared hosting plan from a web hosting provider like namecheap or a VPS or a dedicated Server if you plan to cater more people.
Install Proxy Website on Shared Hosting
This method works fine for shared hosting services and also when you cannot connect or have no console access to the server your sites are being hosted on. This is pretty easy and straight forward.
Download the pre-compiled files of web proxy zip file and upload them to your public directory of web server.
Upload & Extract in the root of your public directory or move the web proxy files in your desired folder inside your public directory. Ask for help from your hosting service provider if you are not sure. Essentially you are looking for something like a File Manager. It may look different and ways to access it may be different for different web hosting providers. Do not freak out if it does not look the same at your end.
Look for File manager in your hosting provider
After Upload & Extract, the files will look something like the following
Now we need to do one more thing before we can start using our proxy site. Edit the file ‘’. The file has the following content.
php // all possible options will be stored $config = array(); // a unique key that identifies this application - DO NOT LEAVE THIS EMPTY! $config['app_key'] = ''; // a secret key to be used during encryption $config['encryption_key'] = ''; /*......
We need to add a random value for $config['app_key']= '';
php
// all possible options will be stored
$config = array();
// a unique key that identifies this application - DO NOT LEAVE THIS EMPTY!
$config['app_key'] = 'SomethingHere';
// a secret key to be used during encryption
$config['encryption_key'] = '';
/*......
That’s it. Save the file and close it.
Now you can use your web proxy by visiting your domain name ‘’.
Install PHP-Proxy on VPS/Dedicated Server Ubuntu 20. 04
For VPS and Dedicated Servers, We need to setup web server first then we will setup PHP-Proxy over it. There are two commonly used web server applications, Apache and Nginx. There is a slight difference in setting up each. If this does not concern you I will advise you to go with Nginx. In my opinion, it has better memory management than Apache.
Setting up Nginx Web Server
SSH into your server and execute the following commands as root in sequence.
sudo apt update
sudo apt install nginx
sudo apt install php7. 4-fpm php7. 4-curl php7. 4-mbstring php7. 4-xml php7. 4-zip
Now we need to install composer then we can install PHP-Proxy with it in our root directory.
sudo apt install composer
Remove any default files in the root folder
sudo rm /var/www/html/*
Now installing PHP-Proxy with Composer.
sudo composer create-project athlon1600/php-proxy-app:dev-master /var/www/html
Change the permission settings and assign web server user all access to website directory.
sudo chown www-data:www-data /var/www/html/ -R
After it is done we need to configure Nginx Web Server.
NGINX Conifguration
Before we proceed to use our PHP-proxy installation we need to configure our web server so that it knows what files point to what domain name and how to execute any PHP scripts.
This is important. Change to the name of your domain.
sudo nano /etc/nginx/sites-available/
Copy and paste the below contents and edit accordingly.
server {
listen 80;
root /var/www/html;
index;
server_name location / {
try_files $uri $uri/ =404;}
location ~ \$ {
include snippets/;
fastcgi_pass unix:/var/run/php/;}
add_header X-Content-Type-Options nosniff;
add_header X-Frame-Options "SAMEORIGIN";
add_header X-XSS-Protection "1; mode=block";
location ~ /\ {
deny all;}}
Link this file to sites-enabled.
sudo ln -s /etc/nginx/sites-available/ /etc/nginx/sites-enabled/sudo unlink /etc/nginx/sites-enabled/default
Restart the web server service so that it can load with new configuration.
sudo systemctl reload nginx
Now if you followed every step carefully and accurately you will be able to access the web proxy by just visiting
Installing SSL Encryption (Let’s Encrypt) [Optional]
If you want to use our web proxy securely then you must install SSL encryption that is HTTPS. It is free and provided by Let’s Encrypt. Execute the following commands to set HTTPS over
sudo apt install certbot python3-certbot-nginx
This is important. Change & to the name of your domain.
sudo certbot --nginx -d -d
You will be asked to enter an email address and then must agree to the terms of service. If certbot assigns a SSL certificate it will ask you about redirecting all traffic to secure HTTPS or you want to keep insecure and ssl access enabled.
Please choose whether or not to redirect HTTP traffic to HTTPS, removing HTTP access. 1: No redirect – Make no further changes to the webserver configuration. 2: Redirect – Make all requests redirect to secure HTTPS access.
You can select option 2 here as we want a secure web proxy connection. And after you press [ENTER] certbot will make necessary changes to redirect all traffic to HTTPS only.
You work here is done. You can check your site will be working with. Comment if you run into any issues or want to add something.