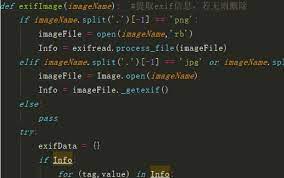
What Is Import Requests In Python
Using the Requests Library in Python – PythonForBeginners.com
First things first, let’s introduce you to is the Requests Resource? Requests is an Apache2 Licensed HTTP library, written in Python. It is designed to be used by humans to interact with the language. This means you don’t have to manually add query strings to URLs, or form-encode your POST data. Don’t worry if that made no sense to you. It will in due can Requests do? Requests will allow you to send HTTP/1. 1 requests using Python. With it, you can add content like headers, form data, multipart files, and parameters via simple Python libraries. It also allows you to access the response data of Python in the same programming, a library is a collection or pre-configured selection of routines, functions, and operations that a program can use. These elements are often referred to as modules, and stored in object braries are important, because you load a module and take advantage of everything it offers without explicitly linking to every program that relies on them. They are truly standalone, so you can build your own programs with them and yet they remain separate from other of modules as a sort of code reiterate, Requests is a Python to Install RequestsThe good news is that there are a few ways to install the Requests library. To see the full list of options at your disposal, you can view the official install documentation for Requests can make use of pip, easy_install, or you’d rather work with source code, you can get that on GitHub, as the purpose of this guide, we are going to use pip to install the your Python interpreter, type the following:pip install requests Importing the Requests ModuleTo work with the Requests library in Python, you must import the appropriate module. You can do this simply by adding the following code at the beginning of your script:import requests Of course, to do any of this – installing the library included – you need to download the necessary package first and have it accessible to the a RequestWhen you ping a website or portal for information this is called making a request. That is exactly what the Requests library has been designed to get a webpage you would do something like the following:r = (‘)Working with Response CodeBefore you can do anything with a website or URL in Python, it’s a good idea to check the current status code of said portal. You can do this with the dictionary look-up object. r = (”)
atus_code
>>200
atus_code ==
>>> True
[‘temporary_redirect’]
>>> 307
>>> 418
[‘o/’]
>>> 200Get the ContentAfter a web server returns a response, you can collect the content you need. This is also done using the get requests requests
r = (”)
print
# The Requests library also comes with a built-in JSON decoder,
# just in case you have to deal with JSON data
import requests
print r. jsonWorking with HeadersBy utilizing a Python dictionary, you can access and view a server’s response headers. Thanks to how Requests works, you can access the headers using any capitalization you’d you perform this function but a header doesn’t exist in the response, the value will default to None. r. headers
{
‘status’: ‘200 OK’,
‘content-encoding’: ‘gzip’,
‘transfer-encoding’: ‘chunked’,
‘connection’: ‘close’,
‘server’: ‘nginx/1. 0. 4’,
‘x-runtime’: ‘148ms’,
‘etag’: ‘”e1ca502697e5c9317743dc078f67693f”‘,
‘content-type’: ‘application/json; charset=utf-8’}
r. headers[‘Content-Type’]
>>>’application/json; charset=utf-8′
r. (‘content-type’)
r. headers[‘X-Random’]
>>>None
# Get the headers of a given URL
resp = (“)
print atus_code,, resp. headersEncodingRequests will automatically decade any content pulled from a server. But most Unicode character sets are seamlessly decoded you make a request to a server, the Requests library make an educated guess about the encoding for the response, and it does this based on the HTTP headers. The encoding that is guessed will be used when you access the rough this file, you can discern what encoding the Requests library is using, and change it if need be. This is possible thanks to the r. encoding property you’ll find in the and when you change the encoding value, Requests will use the new type so long as you call in your r. encoding
>> utf-8
>>> r. encoding = ‘ISO-8859-1’Custom HeadersIf you want to add custom HTTP headers to a request, you must pass them through a dictionary to the headers json
url = ”
payload = {‘some’: ‘data’}
headers = {‘content-type’: ‘application/json’}
r = (url, (payload), headers=headers)Redirection and HistoryRequests will automatically perform a location redirection when you use the GET and OPTIONS verbs in will redirect all HTTP requests to HTTPS automatically. This keeps things secure and can use the history method of the response object to track redirection status. r = (”)
>>> ”
>>> 200
r. history
>>> []Make an HTTP Post RequestYou can also handle post requests using the Requests library. r = ()But you can also rely on other HTTP requests too, like PUT, DELETE, HEAD, and OPTIONS. r = (“)
r = (“)
r = requests. options(“)You can use these methods to accomplish a great many things. For instance, using a Python script to create a GitHub requests, json
github_url = ”
data = ({‘name’:’test’, ‘description’:’some test repo’})
r = (github_url, data, auth=(‘user’, ‘*****’))
print r. jsonErrors and ExceptionsThere are a number of exceptions and error codes you need to be familiar with when using the Requests library in there is a network problem like a DNS failure, or refused connection the Requests library will raise a ConnectionError invalid HTTP responses, Requests will also raise an HTTPError exception, but these are a request times out, a Timeout exception will be and when a request exceeds the preconfigured number of maximum redirections, then a TooManyRedirects exception will be exceptions that Requests raises will be inherited from the questException can read more about the Requests library at the links below. Python TrainingCourse: Python 3 For BeginnersOver 15 hours of video content with guided instruction for beginners. Learn how to create real world applications and master the basics.
Python Requests Module – W3Schools
Example
Make a request to a web page, and print the response text:
import requestsx = (”)print()
Run Example »
Definition and Usage
The requests module allows you to send HTTP
requests using Python.
The HTTP request returns a Response Object with all the response data
(content, encoding, status, etc).
Download and Install the Requests Module
Navigate your command line to the location of PIP, and type the following:
C:\Users\Your Name\AppData\Local\Programs\Python\Python36-32\Scripts>pip install requests
Syntax
thodname(params)
Methods
Method
Description
delete(url, args)
Sends a DELETE request to the specified url
get(url, params, args)
Sends a GET request to the specified url
head(url, args)
Sends a HEAD request to the specified url
patch(url, data, args)
Sends a PATCH request to the specified url
post(url, data, json, args)
Sends a POST request to the specified url
put(url, data, args)
Sends a PUT request to the specified url
request(method, url, args)
Sends a request of the specified method to the specified url
How to PIP Install Requests Python Package – ActiveState
Try a faster and easier way to manage your Python dependencies. Use Python 3. 9 by ActiveState and build your own runtime with the packages and dependencies you need. Get started for free by creating an account on the ActiveState Platform or logging in with your GitHub account.
Requests is a popular open source HTTP library that simplifies working with HTTP requests.
The Requests library is available for both Python 2 and Python 3 from the Python Package Index (PyPI), and has the following features:
Allows you to send HTTP/1. 1 PUT, DELETE, HEAD, GET and OPTIONS requests with ease. For example:
import requests
req = quest(‘GET’, ”)
Req
# Output:
You can use it to send form-encoded data, similar to an HTML form.
PRovides a demystified, readable API for humans. For example, this is how you make an HTTP POST request:
r = (”, data = {‘key’:’value’})”
Prior to the availability of the Requests library, it was necessary to manually add query strings to URLs, and form-encode PUT & POST data. Now you can use Requests with the JSON method instead.
Requests Installation
Check if Requests is already installed and up-to-date by entering the following command:
python -m pip show requests
Output should be similar to:
Name: requests
Version: 2. 26. 0
Summary: Python HTTP for Humans.
Home-page:…
If not installed, you can install Requests on Linux, MacOS, and the Windows operating systems by running:
pip install requests
or
python -m pip install requests
To upgrade requests to the latest version, enter:
pip install –upgrade requests
To install a specific version of requests, eg. version 2. 6. 6, enter:
pip install requests==2. 0
To uninstall Requests, enter:
pip uninstall Requests
Alternate Methods for installing Requests
Install Requests from Source Code
The easiest way to install Requests from source code is to use the ActiveState Platform, which will automatically build and package it for you. Run the following command to create a new project in a virtual environment/ virtual directory:
For Linux, run the following in your terminal:
sh <(curl -q) --activate-default ActiveState-Labs/Python-3. 9Beta
For Windows, run the following in a CMD window:
powershell -Command "& $([scriptblock]::Create((New-Object Net. WebClient). DownloadString(''))) -activate-default ActiveState-Labs/Python-3. 9Beta"
Then run:
state install requests
Install Requests on Ubuntu and Debian Linux
Run the following command:
apt-get install python-requests
Install Requests on Fedora, Redhat and CentOS Linux
yum install python-requests
Install Requests with Git
If you have Git installed, you can use it in conjunction with pip to install Requests by running the following command:
pip install
Pip install Requests into a Virtual Directory
You should always work in a virtual environment to prevent conflicts. You can use pip to install a specific version of the Requests module into a Virtualenv environment for Python 2 or Venv for Python 3 projects.
Syntax:
Assuming that you are working in Python 3, you can set up a virtual directory for a project with the following command:
python3 -m venv
Activate
Linux:
source
Windows:. \env\Scripts\activate
You can pip install Requests into your virtual environment with the following command:
Pip Install Requests as a Dependency
Pip will allow you to declare a specific Requests version as a dependency in a file, along with other dependencies in a virtual environment. For example:
requests==
To install Requests as a dependency along with other dependencies in a file:
python3 -m pip install -r
A modern solution to Python package and dependency management – Try ActiveState’s Platform
Dependency resolution is at the core of the ActiveState Platform. When you create a project and start adding requirements, the Platforms tell you what dependencies those requirements have.
The ActiveState Platform is a cloud-based build tool for Python. It provides build automation and vulnerability remediation for:
Python language cores, including Python 2. 7 and Python 3. 5+
Python packages and their dependencies, including:
Transitive dependencies (ie., dependencies of dependencies)
Linked C and Fortran libraries, so you can build data science packages
Operating system-level dependencies for Windows, Linux, and macOS
Shared dependencies (ie., OpenSSL)
Find, fix and automatically rebuild a secure version of Python packages like Django and environments in minutes
The ActiveState Platform aims to handle every dependency for every language. That means handling libraries down to the C/C++ level, external tools, and all the conditional dependencies that exist. To take things even further, our ultimate goal is to support multi-language projects. That means that you can create a project using both Python and Perl packages, and we’ll make sure that both languages are using the same (up to date) OpenSSL version.
Python Dependency Management In Action
Get a hands-on appreciation for how the ActiveState Platform can help you manage your dependencies for Python environments. Just run the following command to install Python 3. 9 and our package manager, the State Tool:
Windows
Linux
Now you can run state install
Let us know your experience in the ActiveState Community forum.
Watch this video to learn how to use the ActiveState Platform to create a Python 3. 9 environment, and then use the Platform’s CLI (State Tool) to install and manage it.
Frequently Asked Questions about what is import requests in python
What is the requests package in Python?
The requests module allows you to send HTTP requests using Python. The HTTP request returns a Response Object with all the response data (content, encoding, status, etc).
What is PIP install requests in Python?
Pip install Requests into a Virtual Directory You can use pip to install a specific version of the Requests module into a Virtualenv environment for Python 2 or Venv for Python 3 projects. venv will create a virtual Python installation in the <env_name> folder.
What is the use of requests module in Python?
The requests module in Python allows you to exchange requests on the web. It is a very useful library that has many essential methods and features to send HTTP requests. As mentioned earlier, HTTP works as a request-response system between a server and a client.Mar 26, 2020