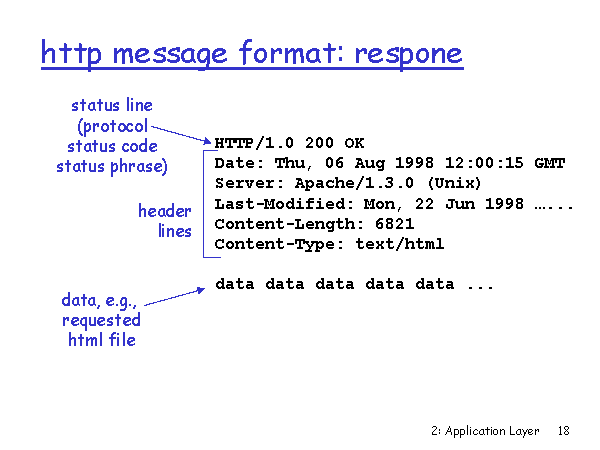
Selenium Run Javascript On Page
Execute javascript within webpage with selenium WebDriver
I am trying to execute a javascript method on a webpage with the selenium WebDriver. I am able to execute my own script on the page, but not call the method from the page’s HTML. Here is the HTML
function Search(){
if(check([0], “searchResults”)){
var fieldCode = “”;
var fieldText = “”;
if(([0]. elements[‘rNumber’] ==””) && (! isPRRNoSelected()))
{
alert(‘PRR No. must be a selected field. ‘);
unSelectAllOptions([0]. availableFieldsListArray);
unSelectAllOptions([0]. selectedFieldsList);}
else
[0]. = “false”;
[0] = “”;
[0]();}}}
If i attempt to submit the form manually it does not load properly. I can execute my own javascript using the code below. How can I execute the “Search” function from the page?
JavascriptExecutor js = (JavascriptExecutor)driver;
js. executeScript(“tElementById(‘fromIssueDate’). removeAttribute(‘readOnly’)”);
Vicky2, 8912 gold badges18 silver badges33 bronze badges
asked Jun 18 ’15 at 16:29
First of all you shouldn’t execute javascript using WebDriver unless you really have no other option. Why doesn’t the submit work? what did you try? In my experience sometimes click doesn’t trigger form submission, so instead you use submit on the form element. Note that if you submit on a non-form element its a no-op. So something like below should work,
WebElement email = ndElement((“email”));
WebElement password = ndElement((“password”));
WebElement submit = ndElement((“submit”));
ndKeys(“John”);
ssword(“foo”);
();
Going back to your original question, is Search() a global function? Then you could do something like below, however like I said, WebDriver doesn’t recommend you invoke javascript since that’s not how real users would interact with your application
js. executeScript(“();”);
answered Jun 18 ’15 at 19:59
nileshnilesh13. 5k6 gold badges60 silver badges76 bronze badges
1
Not the answer you’re looking for? Browse other questions tagged java javascript html selenium webdriver or ask your own question.
Selenium WebDriver and Execute JavaScript – Python Tutorial
You can execute Javascript with the Selenium WebDriver. In this tutorial you will learn how you can run js directly from your Python code.
You an use selenium to do automated testing of web apps or websites, or just automate the web browser. It can automate both the desktop browser and the mobile lenium webdriver can execute Javascript. After loading a page, you can execute any javascript you want. A webdriver must be installed for selenium to work.
All it takes to execute Javascript is calling the method execute_script(js) where js is your javascript code.
Related course:
Selenium Web Automation Course & Examples
javascriptWhat is JavaScript? JavaScript is a scripting languages that was made to run on top of websites (client side). It used to be to only make a webpage interactive, but these days there are complete frameworks that let you build the front-end of apps.
How to Execute Javascript? Before you can use selenium, make sure it is installed and that you also have the right web driver. You can initialize selenium the way you always do.
If you load a website with Python selenium, you can manually inject JavaScript onto that page. If you named your webdriver object driver, then you can execute it like so:
1driver. execute_script(“some javascript code here”);
The program below runs a one line javascript command after loading the page. This will show the alert box in the webpage.
1234567from selenium import refox()plicitly_wait(3)(“)js = ‘alert(“Hello World”)’driver. execute_script(js)
This means you can also use Javascript inside selenium to click on items, like on a button.
1234from selenium import webdriverdriver = refox()(“) driver. execute_script(“tElementsByClassName(‘comment-user’)[0]()”)
To scroll the browser window, you can use Javascript too:
1driver. execute_script(“rollTo(0, );”
But of course, you can also do this the Pythonic way by using the selenium module instead.
If you are new to selenium, then I highly recommend this book.
Download examples
How to Process JavaScript via Selenium – Digital.ai
Last Updated Jan 01, 2020 — Continuous Testing ExpertRead our JavaScript Selenium blog post and discover how the possibilities of JavaScript in your Selenium tests are, indeed, testing in the dark: How full visibility and codeless fill your enterprise testing gapsLearn how to gain visibility in to your organization’s testing process, while creating ease of use for non-technical a copyContinuous TestingIntroductionJavaScript, or, simply, JS is a high-level programming language and one of the core technologies of the World Wide Web. Most web-pages and web-applications heavily utilize JS. And it’s possible to interact with an application on a very complex level by using JavaScript scripts. It’s really, a very powerful tool. A way to turn a good tool into a great one, however, is to use it to enhance automated testing with rtunately, Selenium already has really deep connections with JavaScript to perform some interactions with web applications. And it allows a user to execute any JS script on a current page by invoking executeScript or executeAsyncScript methods of its JavaScriptExecutor execution methodsSo, as stated above, there are two methods that can execute your JS script: executeScript and executeAsyncScript. All of them have a common syntax:((JavascriptExecutor) driver). executeScript(Script, Arguments);Where the driver is your Selenium WebDriver, Script is your JavaScript as String, and Arguments are arguments, you want to pass to the script, accessible via arguments magic variable. Arguments for the script must be a number, a boolean, a String, a WebElement, or a List of any combination of the ‘s take a closer look at each of them. executeScriptExecutes JavaScript in the context of the currently selected frame or window via anonymous Selenium function. To get access to a current page DOM, you can use a document your script returns any value you can assign it to a variable, using types as follows:WebElement – if your script returns an HTML elementDouble – if it’s a decimal numberLong for non-decimal numbersBoolean for a boolean valueString – all the other casesYour script can also return List
Frequently Asked Questions about selenium run javascript on page
Can selenium execute JavaScript?
Fortunately, Selenium already has really deep connections with JavaScript to perform some interactions with web applications. And it allows a user to execute any JS script on a current page by invoking executeScript or executeAsyncScript methods of its JavaScriptExecutor interface.Jan 1, 2020
How can JavaScript be directly executed on WebDriver?
JavascriptExecutor consists of two methods that handle all essential interactions using JavaScript in Selenium. executeScript method – This method executes the test script in the context of the currently selected window or frame. The script in the method runs as an anonymous function.May 24, 2021
Which selenium code can execute JavaScript directly?
JavaScriptExecutor is an interface provided by Selenium Webdriver, which presents a way to execute JavaScript from Webdriver. This interface provides methods to run JavaScript on the selected window or the current page.May 3, 2021