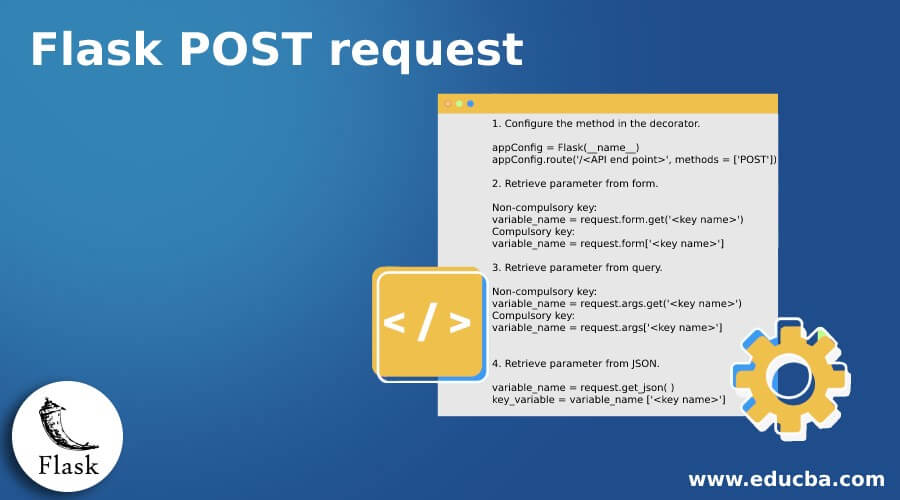
Python Post Request Example
Python Requests post Method – W3Schools
❮ Requests Module
Example
Make a POST request to a web page, and return the response text:
import requestsurl = ”
myobj = {‘somekey’: ‘somevalue’}x = (url, data = myobj)
print()
Run Example »
Definition and Usage
The post() method sends a POST request to the specified url.
The post() method is used when you want to
send some data to the server.
Syntax
(url, data={key: value}, json={key: value},
args)
args means zero or more of the named arguments in the parameter table below. Example:
(url, data = myobj, timeout=2. 50)
Parameter Values
Parameter
Description
url
Try it
Required. The url of the request
data
Optional. A dictionary, list of tuples, bytes or a file object to send to the specified url
json
Optional. A JSON object to send to the specified url
files
Optional. A dictionary of files to send to the specified url
allow_redirects
Optional. A Boolean to enable/disable fault
True (allowing redirects)
auth
Optional. A tuple to enable a certain HTTP fault
None
cert
Optional. A String or Tuple specifying a cert file or fault
cookies
Optional. A dictionary of cookies to send to the specified fault
headers
Optional. A dictionary of HTTP headers to send to the specified fault None
proxies
Optional. A dictionary of the protocol to the proxy fault
stream
Optional. A Boolean indication if the response should be immediately downloaded (False) or streamed (True). Default
False
timeout
Optional. A number, or a tuple, indicating how many seconds to wait for the client to make a connection and/or send a fault None which means the request will continue
until the connection is closed
verify
Optional. A Boolean or a String indication to verify the servers TLS certificate or fault True
Return Value
A sponse object.
❮ Requests Module
GET and POST requests using Python – GeeksforGeeks
This post discusses two HTTP (Hypertext Transfer Protocol) request methods GET and POST requests in Python and their implementation in is HTTP? HTTP is a set of protocols designed to enable communication between clients and servers. It works as a request-response protocol between a client and server. A web browser may be the client, and an application on a computer that hosts a web site may be the, to request a response from the server, there are mainly two methods:GET: to request data from the: to submit data to be processed to the is a simple diagram which explains the basic concept of GET and POST, to make HTTP requests in python, we can use several HTTP libraries like:liburllibrequestsThe most elegant and simplest of above listed libraries is Requests. We will be using requests library in this article. To download and install Requests library, use following command:pip install requestsOR, download it from here and install a Get requestimport requestslocation = “delhi technological university”PARAMS = {‘address’:location}r = (url = URL, params = PARAMS)data = ()latitude = data[‘results’][0][‘geometry’][‘location’][‘lat’]longitude = data[‘results’][0][‘geometry’][‘location’][‘lng’]formatted_address = data[‘results’][0][‘formatted_address’]print(“Latitude:%s\nLongitude:%s\nFormatted Address:%s”%(latitude, longitude, formatted_address))Output:The above example finds latitude, longitude and formatted address of a given location by sending a GET request to the Google Maps API. An API (Application Programming Interface) enables you to access the internal features of a program in a limited fashion. And in most cases, the data provided is in JSON(JavaScript Object Notation) format (which is implemented as dictionary objects in Python! ). Important points to infer:PARAMS = {‘address’:location}The URL for a GET request generally carries some parameters with it. For requests library, parameters can be defined as a dictionary. These parameters are later parsed down and added to the base url or the understand the parameters role, try to print after the response object is created. You will see something like this: is the actual URL on which GET request is mader = (url = URL, params = PARAMS)Here we create a response object ‘r’ which will store the request-response. We use () method since we are sending a GET request. The two arguments we pass are url and the parameters = ()Now, in order to retrieve the data from the response object, we need to convert the raw response content into a JSON type data structure. This is achieved by using json() method. Finally, we extract the required information by parsing down the JSON type a POST requestimport requestsAPI_KEY = “XXXXXXXXXXXXXXXXX”source_code = data = {‘api_dev_key’:API_KEY, ‘api_option’:’paste’, ‘api_paste_code’:source_code, ‘api_paste_format’:’python’}r = (url = API_ENDPOINT, data = data)pastebin_url = r. textprint(“The pastebin URL is:%s”%pastebin_url)This example explains how to paste your source_code to by sending POST request to the PASTEBIN of all, you will need to generate an API key by signing up here and then access your API key portant features of this code:data = {‘api_dev_key’:API_KEY,
‘api_option’:’paste’,
‘api_paste_code’:source_code,
‘api_paste_format’:’python’}Here again, we will need to pass some data to API server. We store this data as a dictionary. r = (url = API_ENDPOINT, data = data)Here we create a response object ‘r’ which will store the request-response. We use () method since we are sending a POST request. The two arguments we pass are url and the data stebin_url = response, the server processes the data sent to it and sends the pastebin URL of your source_code which can be simply accessed by. method could be used for many other tasks as well like filling and submitting the web forms, posting on your FB timeline using the Facebook Graph API, are some important points to ponder upon:When the method is GET, all form data is encoded into the URL, appended to the action URL as query string parameters. With POST, form data appears within the message body of the HTTP GET method, the parameter data is limited to what we can stuff into the request line (URL). Safest to use less than 2K of parameters, some servers handle up to such problem in POST method since we send data in message body of the HTTP request, not the ASCII characters are allowed for data to be sent in GET is no such restriction in POST is less secure compared to POST because data sent is part of the URL. So, GET method should not be used when sending passwords or other sensitive blog is contributed by Nikhil Kumar. If you like GeeksforGeeks and would like to contribute, you can also write an article using or mail your article to See your article appearing on the GeeksforGeeks main page and help other write comments if you find anything incorrect, or you want to share more information about the topic discussed above.
How to send and receive HTTP POST requests in Python – Stack Overflow
For the client side, you can do all sorts of requests using this python library: requests. It is quite intuitive and easy to use/install.
For the server side, I’ll recommend you to use a small web framework like Flask, Bottle or Tornado. These ones are quite easy to use, and lightweight.
For example, a small client-side code to send the post variable foo using requests would look like this:
import requests
r = (“yoururl/post”, data={‘foo’: ‘bar’})
# And done.
print() # displays the result body.
And a server-side code to receive and use the POST request using flask would look like this:
from flask import Flask, request
app = Flask(__name__)
(‘/’, methods=[‘POST’])
def result():
print([‘foo’]) # should display ‘bar’
return ‘Received! ‘ # response to your request.
This is the simplest & quickest way to send/receive a POST request using python.
Frequently Asked Questions about python post request example
How do you write a POST request in python?
Use requests. post() to make a POST request Call requests. post(url, data) to make a POST request to the source url with data attached. This returns a Response object containing the server’s response to the request.
How does Python handle POST request?
route(‘/’, methods=[‘POST’]) def result(): print(request. form[‘foo’]) # should display ‘bar’ return ‘Received ! ‘ # response to your request. This is the simplest & quickest way to send/receive a POST request using python.Jun 28, 2016
How do you send form data in POST request in python?
POST is the most common request method used to send data mostly through ‘form’ to the server for creating/updating in the server. You can use ‘post’ a form data to the route where the ‘requests. post’ and make a dictionary called ‘pload’ where it is sent as an argument to the post to ‘data=pload’.Sep 19, 2019