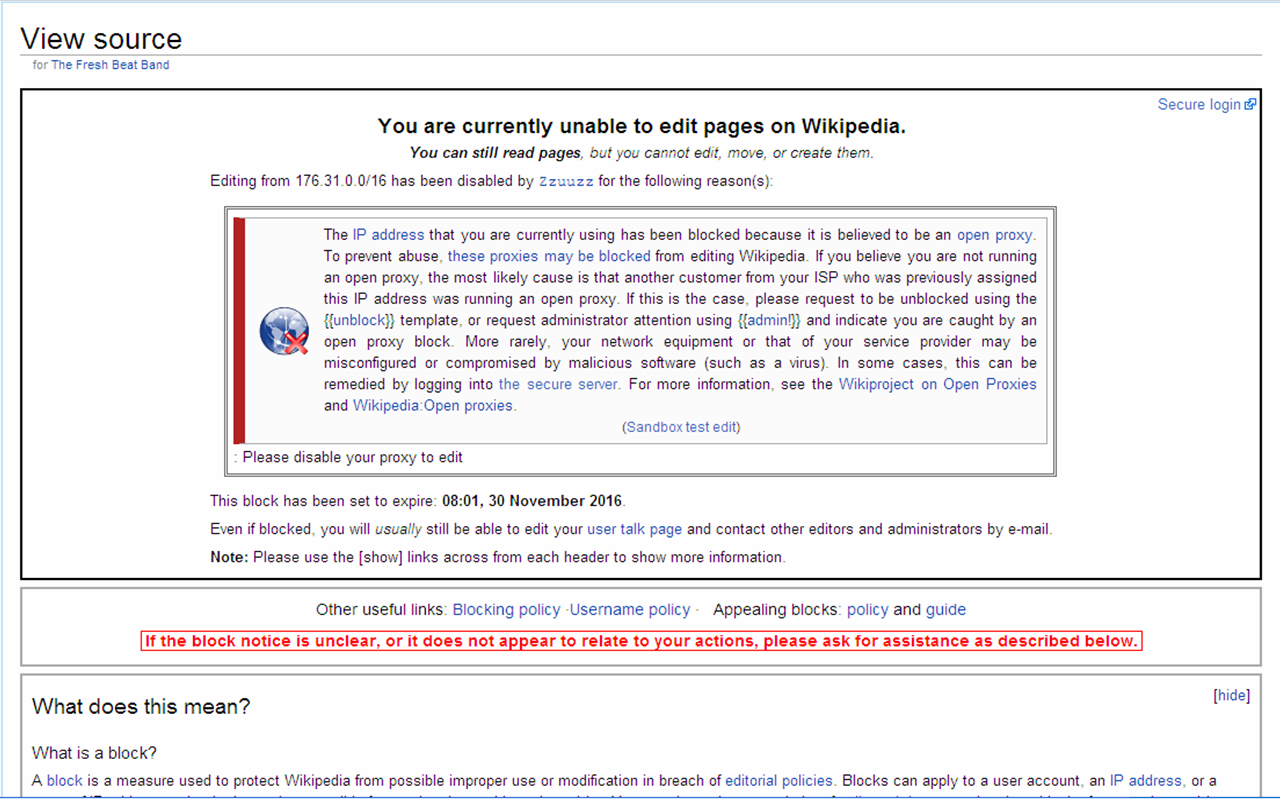
Proxy Php Url
How to proxy another page in PHP – Stack Overflow
I’m looking for the fastest and easiest way to proxy a page in PHP. I don’t want the user to be redirected, I just want my script to return the same content, response code and headers as another remote URL.
asked Jun 21 ’11 at 13:04
2
echo file_get_contents(‘proxypage’);
Would that work?
EDIT:
First answer was a bit short, and I don’t believe it will handle headers as you would like.
However you can also do this:
function get_proxy_site_page( $url)
{
$options = [
CURLOPT_RETURNTRANSFER => true, // return web page
CURLOPT_HEADER => true, // return headers
CURLOPT_FOLLOWLOCATION => true, // follow redirects
CURLOPT_ENCODING => “”, // handle all encodings
CURLOPT_AUTOREFERER => true, // set referer on redirect
CURLOPT_CONNECTTIMEOUT => 120, // timeout on connect
CURLOPT_TIMEOUT => 120, // timeout on response
CURLOPT_MAXREDIRS => 10, // stop after 10 redirects];
$ch = curl_init($url);
curl_setopt_array($ch, $options);
$remoteSite = curl_exec($ch);
$header = curl_getinfo($ch);
curl_close($ch);
$header[‘content’] = $remoteSite;
return $header;}
This will return you an array containing lots of information on the remote page. $header[‘content’] will have both the content of the website and the headers, $header[header_size] will contain the length of that header so you can use substr to split those up.
Then it’s just a matter of using echoand header to proxy the page.
answered Jun 21 ’11 at 13:10
klennepetteklennepette3, 07619 silver badges23 bronze badges
1
You can use the PHP cURL functions to achieve this functionality:
// create a new cURL resource
$ch = curl_init();
// set URL and other appropriate options
curl_setopt($ch, CURLOPT_URL, ”);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// grab URL and pass it to the browser
$urlContent = curl_exec($ch);
From this point, you would grab the response header information using. (There are several values you can grab, all listed in the documentation).
// Check if any error occured
if(! curl_errno($ch))
$info = curl_getinfo($ch);
header(‘Content-Type: ‘. $info[‘content_type’]);
echo $urlContent;}
Make sure to close out the cURL handle.
// close cURL resource, and free up system resources
answered Jun 21 ’11 at 13:21
forgueamforgueam1331 silver badge6 bronze badges
You can get the html of the next page with curl, and then echo the response.
answered Jun 21 ’11 at 13:11
Question MarkQuestion Mark3, 5471 gold badge23 silver badges30 bronze badges
Not the answer you’re looking for? Browse other questions tagged php proxy or ask your own question.
proxy.php · GitHub
Instantly share code, notes, and snippets.
php
/**
* proxy for getting json data via CURL
*/
header('Cache-Control: no-cache, must-revalidate');
header('Expires: Mon, 26 Jul 1997 05:00:00 GMT');
header('Content-type: application/json');
$url = $_REQUEST['url'];
// clean the url here and make sure it's valid and escaped... then:
// $url = cleanURL($url);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HEADER, 0);
$response = curl_exec($ch);
curl_close($ch);
echo $response;? >
then simply do a local
({
url: ‘/’,
onComplete: function(data) {
(data);}})();
to make it safer, you can just hardwire the url into the proxy and possibly append escaped search terms or whatever so these will become your only argument.
This comment has been minimized.
your php script is not retruning any data at the moment. you still need an echo/print;)
It was not intended to be fully functional but fair enough:)
Simple PHP Proxy – Jon LaBelle
With Simple PHP Proxy, your JavaScript can access content in remote webpages, without cross-domain security limitations, even if it’s not available in JSONP format. Of course, you’ll need to install this PHP script on your server, but that’s a small price to have to pay for this much awesomeness.
PHP
Raw
php
// Script: Simple PHP Proxy: Get external HTML, JSON and more!
//
// *Version: 1. 6, Last updated: 1/24/2009*
// Project Home - // GitHub - // Source - //
// About: License
// Copyright (c) 2010 "Cowboy" Ben Alman,
// Dual licensed under the MIT and GPL licenses.
// //
// About: Examples
// This working example, complete with fully commented code, illustrates one way
// in which this PHP script can be used.
// Simple - //
// About: Release History
// 1. 6 - (1/24/2009) Now defaults to JSON mode, which can now be changed to
// native mode by specifying? mode=native. Native and JSONP modes are
// disabled by default because of possible XSS vulnerability issues, but
// are configurable in the PHP script along with a url validation regex.
// 1. 5 - (12/27/2009) Initial release
// Topic: GET Parameters
// Certain GET (query string) parameters may be passed into
// to control its behavior, this is a list of these parameters.
// url - The remote URL resource to fetch. Any GET parameters to be passed
// through to the remote URL resource must be urlencoded in this parameter.
// mode - If mode=native, the response will be sent using the same content
// type and headers that the remote URL resource returned. If omitted, the
// response will be JSON (or JSONP).
// are disabled by default, see
// callback – If specified, the response JSON will be wrapped in this named
// function call. This parameter and
// default, see
// user_agent – This value will be sent to the remote URL request as the
// `User-Agent:` HTTP request header. If omitted, the browser user agent
// will be passed through.
// send_cookies – If send_cookies=1, all cookies will be forwarded through to
// the remote URL request.
// send_session – If send_session=1 and send_cookies=1, the SID cookie will be
// forwarded through to the remote URL request.
// full_headers – If a JSON request and full_headers=1, the JSON response will
// contain detailed header information.
// full_status – If a JSON request and full_status=1, the JSON response will
// contain detailed cURL status information, otherwise it will just contain
// the `_code` property.
// Topic: POST Parameters
// All POST parameters are automatically passed through to the remote URL
// request.
// Topic: JSON requests
// This request will return the contents of the specified url in JSON format.
// Request:
// >
// Response:
// > { “contents”: “… “, “headers”: {… }, “status”: {… }}
// JSON object properties:
// contents – (String) The contents of the remote URL resource.
// headers – (Object) A hash of HTTP headers returned by the remote URL
// resource.
// status – (Object) A hash of status codes returned by cURL.
// Topic: JSONP requests
// This request will return the contents of the specified url in JSONP format
// (but only if $enable_jsonp is enabled in the PHP script).
// > foo({ “contents”: “… }})
// Topic: Native requests
// This request will return the contents of the specified url in the format it
// was received in, including the same content-type and other headers (but only
// if $enable_native is enabled in the PHP script).
// > …
// Topic: Notes
// * Assumes magic_quotes_gpc = Off in
// Topic: Configuration Options
// These variables can be manually edited in the PHP file if necessary.
// $enable_jsonp – Only enable
// install this script on the same server as the page you’re calling it
// from, plain JSON will work. Defaults to false.
// $enable_native – You can enable
// this if you also whitelist specific URLs using $valid_url_regex, to avoid
// possible XSS vulnerabilities. Defaults to false.
// $valid_url_regex – This regex is matched against the url parameter to
// ensure that it is valid. This setting only needs to be used if either
// $enable_jsonp or $enable_native are enabled. Defaults to ‘/. */’ which
// validates all URLs.
// ############################################################################
// Change these configuration options if needed, see above descriptions for info.
$enable_jsonp = false;
$enable_native = false;
$valid_url_regex = ‘/. */’;
$url = $_GET[‘url’];
if (! $url) {
// Passed url not specified.
$contents = ‘ERROR: url not specified’;
$status = array(‘_code’ => ‘ERROR’);} elseif (! preg_match($valid_url_regex, $url)) {
// Passed url doesn’t match $valid_url_regex.
$contents = ‘ERROR: invalid url’;
$status = array(‘_code’ => ‘ERROR’);} else {
$ch = curl_init($url);
if (strtolower($_SERVER[‘REQUEST_METHOD’]) == ‘post’) {
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $_POST);}
if ($_GET[‘send_cookies’]) {
$cookie = array();
foreach ($_COOKIE as $key => $value) {
$cookie[] = $key. ‘=’. $value;}
if ($_GET[‘send_session’]) {
$cookie[] = SID;}
$cookie = implode(‘; ‘, $cookie);
curl_setopt($ch, CURLOPT_COOKIE, $cookie);}
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($ch, CURLOPT_HEADER, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERAGENT, $_GET[‘user_agent’]? $_GET[‘user_agent’]: $_SERVER[‘HTTP_USER_AGENT’]);
list($header, $contents) = preg_split(‘/([\r\n][\r\n])\\1/’, curl_exec($ch), 2);
$status = curl_getinfo($ch);
curl_close($ch);}
// Split header text into an array.
$header_text = preg_split(‘/[\r\n]+/’, $header);
if ($_GET[‘mode’] == ‘native’) {
if (! $enable_native) {
$contents = ‘ERROR: invalid mode’;
$status = array(‘_code’ => ‘ERROR’);}
// Propagate headers to response.
foreach ($header_text as $header) {
if (preg_match(‘/^(? :Content-Type|Content-Language|Set-Cookie):/i’, $header)) {
header($header);}}
print $contents;} else {
// $data will be serialized into JSON data.
$data = array();
// Propagate all HTTP headers into the JSON data object.
if ($_GET[‘full_headers’]) {
$data[‘headers’] = array();
preg_match(‘/^(. +? ):\s+(. *)$/’, $header, $matches);
if ($matches) {
$data[‘headers’][ $matches[1]] = $matches[2];}}}
// Propagate all cURL request / response info to the JSON data object.
if ($_GET[‘full_status’]) {
$data[‘status’] = $status;} else {
$data[‘status’] = array();
$data[‘status’][‘_code’] = $status[‘_code’];}
// Set the JSON data object contents, decoding it from JSON if possible.
$decoded_json = json_decode($contents);
$data[‘contents’] = $decoded_json? $decoded_json: $contents;
// Generate appropriate content-type header.
$is_xhr = strtolower($_SERVER[‘HTTP_X_REQUESTED_WITH’]) == ‘xmlrequest’;
header(‘Content-type: application/’. ($is_xhr? ‘json’: ‘x-javascript’));
// Get JSONP callback.
$jsonp_callback = $enable_jsonp && isset($_GET[‘callback’])? $_GET[‘callback’]: null;
// Generate JSON/JSONP string
$json = json_encode($data);
print $jsonp_callback? “$jsonp_callback($json)”: $json;}