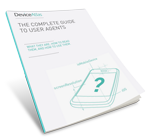
Parsing A File In Python
How to Read a Text file In Python Effectively
Summary: in this tutorial, you learn various ways to read text files in;DRThe following shows how to read all texts from the file into a string:with open(”) as f:
lines = adlines()Code language: JavaScript (javascript)Steps for reading a text file in PythonTo read a text file in Python, you follow these steps:First, open a text file for reading by using the open(), read text from the text file using the file read(), readline(), or readlines() method of the file, close the file using the file close() method. 1) open() functionThe open() function has many parameters but you’ll be focusing on the first (path_to_file, mode)The path_to_file parameter specifies the path to the text the file is in the same folder as the program, you just need to specify the name of the file. Otherwise, you need to specify the path to the specify the path to the file, you use the forward-slash (‘/’) even if you’re working in example, if the file is stored in the sample folder as the program, you need to specify the path to the file as c:/sample/ mode is an optional parameter. It’s a string that specifies the mode in which you want to open the following table shows available modes for opening a text file:ModeDescription’r’Open for text file for reading text’w’Open a text file for writing text’a’Open a text file for appending textFor example, to open a file whose name is stored in the same folder as the program, you use the following code: f = open(”, ‘r’)Code language: JavaScript (javascript)The open() function returns a file object which you will use to read text from a text file. 2) Reading text methodsThe file object provides you with three methods for reading text from a text file:read() – read all text from a file into a string. This method is useful if you have a small file and you want to manipulate the whole text of that adline() – read the text file line by line and return all the lines as adlines() – read all the lines of the text file and return them as a list of strings. 3) close() methodThe file that you open will remain open until you close it using the close() ’s important to close the file that is no longer in use. If you don’t close the file, the program may crash or the file would be following shows how to call the close() method to close the ()Code language: CSS (css)To close the file automatically without calling the close() method, you use the with statement like this:with open(path_to_file) as f:
contents = adlines()Code language: JavaScript (javascript)In practice, you’ll use the with statement to close the file ading a text file examplesWe’ll use file for the following example illustrates how to use the read() method to read all the contents of the file into a string:with open(”) as f:
contents = ()
print(contents)Code language: JavaScript (javascript)Output:Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex….
The following example uses the readlines() method to read the text file and returns the file contents as a list of strings:lines = []
with open(”) as f:
lines = adlines()
count = 0
for line in lines:
count += 1
print(f’line {count}: {line}’) Code language: JavaScript (javascript)Output:line 1: Beautiful is better than ugly.
line 2: Explicit is better than implicit.
line 3: Simple is better than complex….
The following example shows how to use the readline() to read the text file line by line:with open(”) as f:
line = adline()
while line:
print(line)Code language: JavaScript (javascript)Output:Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated….
A more concise way to read a text file line by lineThe open() function returns a file object which is an iterable object. Therefore, you can use a for loop to iterate over the lines of a text file as follows:with open(”) as f:
for line in f:
print(line)Code language: JavaScript (javascript)This is more concise way to read a text file line by UTF-8 text filesThe code in the previous examples works fine with ASCII text files. However, if you’re dealing with other languages such as Japanese, Chinese, and Korean, the text file is not a simple ASCII text file. And it’s likely a UTF-8 file that uses more than just the standard ASCII text open a UTF-8 text file, you need to pass the encoding=’utf-8′ to the open() function to instruct it to expect UTF-8 characters from the the demonstration, you’ll use the following file that contains some quotes in following shows how to loop through the file:with open(”, encoding=’utf8′) as f:
print(())
Code language: JavaScript (javascript)Output:SummaryUse the open() function with the ‘r’ mode to open a text file for the read(), readline(), or readlines() method to read a text close a file after completing reading it using the close() method or the with the encoding=’utf-8′ to read the UTF-8 text you find this tutorial helpful?
Read a File Line-by-Line in Python – Stack Abuse
Introduction
A common task in programming is opening a file and parsing its contents. What do you do when the file you are trying to process is quite large, like several GB of data or larger? The answer to this problem is to read in chunks of a file at a time, process it, then free it from memory so you can process another chunk, until the whole massive file has been processed. While it’s up to you to determine a suitable size for the chunks of data you’re processing, for many applications it’s suitable to process a file one line at a time.
Throughout this article we’ll be covering a number of code examples that demonstrate how to read files line by line. In case you want to try out some of these examples by yourself, the code used in this article can be found at the following GitHub repo.
Basic File IO in Python
Read a File Line-by-Line in Python with readline()
Read a File Line-by-Line in Python with readlines()
Read a File Line-by-Line with a for Loop – Best Approach!
Applications of Reading Files Line-by-Line
Python is a great general purpose programming language, and it has a number of very useful file IO functionality in its standard library of built-in functions and modules.
The built-in open() function is what you use to open a file object for either reading or writing purposes. Here’s how you can use it to open a file:
fp = open(‘path/to/’, ‘r’)
As demonstrated above, the open() function takes in multiple arguments. We will be focusing on two arguments, with the first being a positional string parameter representing the path to the file you want to open. The second (optional) parameter is also a string, and it specifies the mode of interaction you intend to be used on the file object being returned by the function call. The most common modes are listed in the table below, with the default being ‘r’ for reading:
Mode
Description
r
Open for reading plain text
w
Open for writing plain text
a
Open an existing file for appending plain text
rb
Open for reading binary data
wb
Open for writing binary data
Once you have written or read all of the desired data in a file object, you need to close the file so that resources can be reallocated on the operating system that the code is running on.
()
Note: It’s always good practice to close a file object resource, but it’s a task that’s easy to forget.
While you can always remember to call close() on a file object, there’s an alternate and more elegant way to open a file object and ensure that the Python interpreter cleans up after its use:
with open(‘path/to/’) as fp:
# Do stuff with fp
By simply using the with keyword (introduced in Python 2. 5) to the code we use to open a file object, Python will do something similar to the following code. This ensures that no matter what the file object is closed after use:
try:
fp = open(‘path/to/’)
finally:
Either of these two methods are suitable, with the first example being more Pythonic.
The file object returned from the open() function has three common explicit methods (read(), readline(), and readlines()) to read in data. The read() method reads in all the data into a single string. This is useful for smaller files where you would like to do text manipulation on the entire file. Then there is readline(), which is a useful way to only read in individual lines, in incremental amounts at a time, and return them as strings. The last explicit method, readlines(), will read all the lines of a file and return them as a list of strings.
Note: For the remainder of this article we will be working with the text of the book The “Iliad of Homer”, which can be found at, as well as in the GitHub repo where the code is for this article.
Let’s start off with the the readline() method, which reads a single line, which will require us to use a counter and increment it:
filepath = ”
with open(filepath) as fp:
line = adline()
cnt = 1
while line:
print(“Line {}: {}”(cnt, ()))
cnt += 1
This code snippet opens a file object whose reference is stored in fp, then reads in a line one at a time by calling readline() on that file object iteratively in a while loop. It then simply prints the line to the console.
Running this code, you should see something like the following:…
Line 567: exceedingly trifling. We have no remaining inscription earlier than the
Line 568: fortieth Olympiad, and the early inscriptions are rude and unskilfully
Line 569: executed; nor can we even assure ourselves whether Archilochus, Simonides
Line 570: of Amorgus, Kallinus, Tyrtaeus, Xanthus, and the other early elegiac and
Line 571: lyric poets, committed their compositions to writing, or at what time the
Line 572: practice of doing so became familiar. The first positive ground which
Line 573: authorizes us to presume the existence of a manuscript of Homer, is in the
Line 574: famous ordinance of Solon, with regard to the rhapsodies at the
Line 575: Panathenaea: but for what length of time previously manuscripts had
Line 576: existed, we are unable to say….
Though, this approach is crude and explicit. Most certainly not very Pythonic. We can utilize the readlines() method to make this code much more succinct.
Read a File Line-by-Line with readlines()
The readlines() method reads all the lines and stores them into a List. We can then iterate over that list and using enumerate(), make an index for each line for our convenience:
file = open(”, ‘r’)
lines = adlines()
for index, line in enumerate(lines):
print(“Line {}: {}”(index, ()))
This results in:
Check out our hands-on, practical guide to learning Git, with best-practices, industry-accepted standards, and included cheat sheet. Stop Googling Git commands and actually learn it!…
Line 160: INTRODUCTION.
Line 161:
Line 162:
Line 163: Scepticism is as much the result of knowledge, as knowledge is of
Line 164: scepticism. To be content with what we at present know, is, for the most
Line 165: part, to shut our ears against conviction; since, from the very gradual
Line 166: character of our education, we must continually forget, and emancipate
Line 167: ourselves from, knowledge previously acquired; we must set aside old
Line 168: notions and embrace fresh ones; and, as we learn, we must be daily
Line 169: unlearning something which it has cost us no small labour and anxiety to
Line 170: acquire….
Now, although much better, we don’t even need to call the readlines() method to achieve this same functionality. This is the traditional way of reading a file line-by-line, but there’s a more modern, shorter one.
Read a File Line-by-Line with a for Loop – Most Pythonic Approach
The returned File itself is an iterable. We don’t need to extract the lines via readlines() at all – we can iterate the returned object itself. This also makes it easy to enumerate() it so we can write the line number in each print() statement.
This is the shortest, most Pythonic approach to solving the problem, and the approach favored by most:
with open(”) as f:
for index, line in enumerate(f):
This results in:…
Line 277: Mentes, from Leucadia, the modern Santa Maura, who evinced a knowledge and
Line 278: intelligence rarely found in those times, persuaded Melesigenes to close
Line 279: his school, and accompany him on his travels. He promised not only to pay
Line 280: his expenses, but to furnish him with a further stipend, urging, that,
Line 281: “While he was yet young, it was fitting that he should see with his own
Line 282: eyes the countries and cities which might hereafter be the subjects of his
Line 283: discourses. ” Melesigenes consented, and set out with his patron,
Line 284: “examining all the curiosities of the countries they visited, and…
Here, we’re taking advantage of the built-in functionalities of Python that allow us to effortlessly iterate over an iterable object, simply using a for loop. If you’d like to read more about Python’s built-in functionalities on iterating objects, we’ve got you covered:
Python’s itertools – count(), cycle() and chain()
Python’s itertools: filter(), islice(), map() and zip()
How can you use this practically? Most NLP applications deal with large corpora of data. Most of the time, it won’t be wise to read the entire corpora into memory. While rudimentary, you can write a from-scratch solution to count the frequency of certain words, without using any external libraries. Let’s write a simple script that loads in a file, reads it line-by-line and counts the frequency of words, printing the 10 most frequent words and the number of their occurrences:
import sys
import os
def main():
filepath = [1]
if not (filepath):
print(“File path {} does not exist. Exiting… “(filepath))
bag_of_words = {}
for line in fp:
record_word_cnt(()(‘ ‘), bag_of_words)
sorted_words = order_bag_of_words(bag_of_words, desc=True)
print(“Most frequent 10 words {}”(sorted_words[:10]))
def order_bag_of_words(bag_of_words, desc=False):
words = [(word, cnt) for word, cnt in ()]
return sorted(words, key=lambda x: x[1], reverse=desc)
def record_word_cnt(words, bag_of_words):
for word in words:
if word! = ”:
if () in bag_of_words:
bag_of_words[()] += 1
else:
bag_of_words[()] = 1
if __name__ == ‘__main__’:
main()
The script uses the os module to make sure that the file we’re attempting to read actually exists. If so, its read line-by-line and each line is passed on into the record_word_cnt() function. It delimits the spaces between words and adds the word to the dictionary – bag_of_words. Once all the lines are recorded into the dictionary, we order it via order_bag_of_words() which returns a list of tuples in the (word, word_count) format, sorted by the word count.
Finally, we print the top ten most common words.
Typically, for this, you’d create a Bag of Words Model, using libraries like NLTK, though, this implementation will suffice. Let’s run the script and provide our to it:
$ python
Most frequent 10 words [(‘the’, 15633), (‘and’, 6959), (‘of’, 5237), (‘to’, 4449), (‘his’, 3440), (‘in’, 3158), (‘with’, 2445), (‘a’, 2297), (‘he’, 1635), (‘from’, 1418)]
If you’d like to read more about NLP, we’ve got a series of guides on various tasks: Natural Language Processing in Python.
Conclusion
In this article, we’ve explored multiple ways to read a file line-by-line in Python, as well as created a rudimentary Bag of Words model to calculate the frequency of words in a given file.
Parsing text with Python – vipinajayakumar
I hate parsing files, but it is something that I have had to do at the start of nearly every project. Parsing is not easy, and it can be a stumbling block for beginners. However, once you become comfortable with parsing files, you never have to worry about that part of the problem. That is why I recommend that beginners get comfortable with parsing files early on in their programming education. This article is aimed at Python beginners who are interested in learning to parse text files.
In this article, I will introduce you to my system for parsing files. I will briefly touch on parsing files in standard formats, but what I want to focus on is the parsing of complex text files. What do I mean by complex? Well, we will get to that, young padawan.
For reference, the slide deck that I use to present on this topic is available here. All of the code and the sample text that I use is available in my Github repo here.
Why parse files?
The big picture
Parsing text in standard format
Parsing text using string methods
Parsing text in complex format using regular expressions
Step 1: Understand the input format
Step 2: Import the required packages
Step 3: Define regular expressions
Step 4: Write a line parser
Step 5: Write a file parser
Step 6: Test the parser
Is this the best solution?
Conclusion
First, let us understand what the problem is. Why do we even need to parse files? In an imaginary world where all data existed in the same format, one could expect all programs to input and output that data. There would be no need to parse files. However, we live in a world where there is a wide variety of data formats. Some data formats are better suited to different applications. An individual program can only be expected to cater for a selection of these data formats. So, inevitably there is a need to convert data from one format to another for consumption by different programs. Sometimes data is not even in a standard format which makes things a little harder.
So, what is parsing?
Parse
Analyse (a string or text) into logical syntactic components.
I don’t like the above Oxford dictionary definition. So, here is my alternate definition.
Convert data in a certain format into a more usable format.
With that definition in mind, we can imagine that our input may be in any format. So, the first step, when faced with any parsing problem, is to understand the input data format. If you are lucky, there will be documentation that describes the data format. If not, you may have to decipher the data format for yourselves. That is always fun.
Once you understand the input data, the next step is to determine what would be a more usable format. Well, this depends entirely on how you plan on using the data. If the program that you want to feed the data into expects a CSV format, then that’s your end product. For further data analysis, I highly recommend reading the data into a pandas DataFrame.
If you a Python data analyst then you are most likely familiar with pandas. It is a Python package that provides the DataFrame class and other functions to do insanely powerful data analysis with minimal effort. It is an abstraction on top of Numpy which provides multi-dimensional arrays, similar to Matlab. The DataFrame is a 2D array, but it can have multiple row and column indices, which pandas calls MultiIndex, that essentially allows it to store multi-dimensional data. SQL or database style operations can be easily performed with pandas (Comparison with SQL). Pandas also comes with a suite of IO tools which includes functions to deal with CSV, MS Excel, JSON, HDF5 and other data formats.
Although, we would want to read the data into a feature-rich data structure like a pandas DataFrame, it would be very inefficient to create an empty DataFrame and directly write data to it. A DataFrame is a complex data structure, and writing something to a DataFrame item by item is computationally expensive. It’s a lot faster to read the data into a primitive data type like a list or a dict. Once the list or dict is created, pandas allows us to easily convert it to a DataFrame as you will see later on. The image below shows the standard process when it comes to parsing any file.
If your data is in a standard format or close enough, then there is probably an existing package that you can use to read your data with minimal effort.
For example, let’s say we have a CSV file,
a, b, c
1, 2, 3
4, 5, 6
7, 8, 9
You can handle this easily with pandas.
123
import pandas as pd
df = ad_csv(”)
df
a b c
0 1 2 3
1 4 5 6
2 7 8 9
Python is incredible when it comes to dealing with strings. It is worth internalising all the common string operations. We can use these methods to extract data from a string as you can see in the simple example below.
1 2 3 4 5 6 7 8 9101112131415161718192021
my_string = ‘Names: Romeo, Juliet’
# split the string at ‘:’
step_0 = (‘:’)
# get the first slice of the list
step_1 = step_0[1]
# split the string at ‘, ‘
step_2 = (‘, ‘)
# strip leading and trailing edge spaces of each item of the list
step_3 = [() for name in step_2]
# do all the above operations in one go
one_go = [() for name in (‘:’)[1](‘, ‘)]
for idx, item in enumerate([step_0, step_1, step_2, step_3]):
print(“Step {}: {}”(idx, item))
print(“Final result in one go: {}”(one_go))
Step 0: [‘Names’, ‘ Romeo, Juliet’]
Step 1: Romeo, Juliet
Step 2: [‘ Romeo’, ‘ Juliet’]
Step 3: [‘Romeo’, ‘Juliet’]
Final result in one go: [‘Romeo’, ‘Juliet’]
As you saw in the previous two sections, if the parsing problem is simple we might get away with just using an existing parser or some string methods. However, life ain’t always that easy. How do we go about parsing a complex text file?
with open(”) as file:
file_contents = ()
print(file_contents)
Sample text
A selection of students from Riverdale High and Hogwarts took part in a quiz.
Below is a record of their scores.
School = Riverdale High
Grade = 1
Student number, Name
0, Phoebe
1, Rachel
Student number, Score
0, 3
1, 7
Grade = 2
0, Angela
1, Tristan
2, Aurora
0, 6
1, 3
2, 9
School = Hogwarts
0, Ginny
1, Luna
0, 8
0, Harry
1, Hermione
0, 5
1, 10
Grade = 3
0, Fred
1, George
0, 0
1, 0
That’s a pretty complex input file! Phew! The data it contains is pretty simple though as you can see below:
Name Score
School Grade Student number
Hogwarts 1 0 Ginny 8
1 Luna 7
2 0 Harry 5
1 Hermione 10
3 0 Fred 0
1 George 0
Riverdale High 1 0 Phoebe 3
1 Rachel 7
2 0 Angela 6
1 Tristan 3
2 Aurora 9
The sample text looks similar to a CSV in that it uses commas to separate out some information. There is a title and some metadata at the top of the file. There are five variables: School, Grade, Student number, Name and Score. School, Grade and Student number are keys. Name and Score are fields. For a given School, Grade, Student number there is a Name and a Score. In other words, School, Grade, and Student Number together form a compound key.
The data is given in a hierarchical format. First, a School is declared, then a Grade. This is followed by two tables providing Name and Score for each Student number. Then Grade is incremented. This is followed by another set of tables. Then the pattern repeats for another School. Note that the number of students in a Grade or the number of classes in a school are not constant, which adds a bit of complexity to the file. This is just a small dataset. You can easily imagine this being a massive file with lots of schools, grades and students.
It goes without saying that the data format is exceptionally poor. I have done this on purpose. If you understand how to handle this, then it will be a lot easier for you to master simpler formats. It’s not unusual to come across files like this if have to deal with a lot of legacy systems. In the past when those systems were being designed, it may not have been a requirement for the data output to be machine readable. However, nowadays everything needs to be machine-readable!
We will need the Regular expressions module and the pandas package. So, let’s go ahead and import those.
12
import re
In the last step, we imported re, the regular expressions module. What is it though?
Well, earlier on we saw how to use the string methods to extract data from text. However, when parsing complex files, we can end up with a lot of stripping, splitting, slicing and whatnot and the code can end up looking pretty unreadable. That is where regular expressions come in. It is essentially a tiny language embedded inside Python that allows you to say what string pattern you are looking for. It is not unique to Python by the way (treehouse).
You do not need to become a master at regular expressions. However, some basic knowledge of regexes can be very handy in your programming career. I will only teach you the very basics in this article, but I encourage you to do some further study. I also recommend regexper for visualising regular expressions. regex101 is another excellent resource for testing your regular expression.
We are going to need three regexes. The first one, as shown below, will help us to identify the school. Its regular expression is School = (. *)\n. What do the symbols mean?. : Any character
*: 0 or more of the preceding expression
(. *): Placing part of a regular expression inside parentheses allows you to group that part of the expression. So, in this case, the grouped part is the name of the school.
\n: The newline character at the end of the line
We then need a regular expression for the grade. Its regular expression is Grade = (\d+)\n. This is very similar to the previous expression. The new symbols are:
\d: Short for [0-9]
+: 1 or more of the preceding expression
Finally, we need a regular expression to identify whether the table that follows the expression in the text file is a table of names or scores. Its regular expression is (Name|Score). The new symbol is:
|: Logical or statement, so in this case, it means ‘Name’ or ‘Score. ’
We also need to understand a few regular expression functions:
mpile(pattern): Compile a regular expression pattern into a RegexObject.
A RegexObject has the following methods:
match(string): If the beginning of string matches the regular expression, return a corresponding MatchObject instance. Otherwise, return None.
search(string): Scan through string looking for a location where this regular expression produced a match, and return a corresponding MatchObject instance. Return None if there are no matches.
A MatchObject always has a boolean value of True. Thus, we can just use an if statement to identify positive matches. It has the following method:
group(): Returns one or more subgroups of the match. Groups can be referred to by their index. group(0) returns the entire match. group(1) returns the first parenthesized subgroup and so on. The regular expressions we used only have a single group. Easy! However, what if there were multiple groups? It would get hard to remember which number a group belongs to. A Python specific extension allows us to name the groups and refer to them by their name instead. We can specify a name within a parenthesized group (… ) like so: (? P
Let us first define all the regular expressions. Be sure to use raw strings for regex, i. e., use the subscript r before each pattern.
1234567
# set up regular expressions
# use to visualise these if required
rx_dict = {
‘school’: mpile(r’School = (? P
‘grade’: mpile(r’Grade = (? P
‘name_score’: mpile(r'(? P
Then, we can define a function that checks for regex matches.
1 2 3 4 5 6 7 8 910111213
def _parse_line(line):
“””
Do a regex search against all defined regexes and
return the key and match result of the first matching regex
for key, rx in ():
match = (line)
if match:
return key, match
# if there are no matches
return None, None
Finally, for the main event, we have the file parser function. It is quite big, but the comments in the code should hopefully help you understand the logic.
1 2 3 4 5 6 7 8 91011121314151617181920212223242526272829303132333435363738394041424344454647484950515253545556575859606162636465
def parse_file(filepath):
Parse text at given filepath
Parameters
———-
filepath: str
Filepath for file_object to be parsed
Returns
——-
data: Frame
Parsed data
data = [] # create an empty list to collect the data
# open the file and read through it line by line
with open(filepath, ‘r’) as file_object:
line = adline()
while line:
# at each line check for a match with a regex
key, match = _parse_line(line)
# extract school name
if key == ‘school’:
school = (‘school’)
# extract grade
if key == ‘grade’:
grade = (‘grade’)
grade = int(grade)
# identify a table header
if key == ‘name_score’:
# extract type of table, i. e., Name or Score
value_type = (‘name_score’)
# read each line of the table until a blank line
while ():
# extract number and value
number, value = ()(‘, ‘)
value = ()
# create a dictionary containing this row of data
row = {
‘School’: school,
‘Grade’: grade,
‘Student number’: number,
value_type: value}
# append the dictionary to the data list
(row)
# create a pandas DataFrame from the list of dicts
data = Frame(data)
# set the School, Grade, and Student number as the index
t_index([‘School’, ‘Grade’, ‘Student number’], inplace=True)
# consolidate df to remove nans
data = oupby()()
# upgrade Score from float to integer
data = (_numeric, errors=’ignore’)
return data
We can use our parser on our sample text like so:
1234
if __name__ == ‘__main__’:
filepath = ”
data = parse(filepath)
print(data)
This is all well and good, and you can see by comparing the input and output by eye that the parser is working correctly. However, the best practice is to always write unittests to make sure your code is doing what you intended it to do. Whenever you write a parser, please ensure that it’s well tested. I have gotten into trouble with my colleagues for using parsers without testing before. Eeek! It’s also worth noting that this does not necessarily need to be the last step. Indeed, lots of programmers preach about Test Driven Development. I have not included a test suite here as I wanted to keep this tutorial concise.
I have been parsing text files for a year and perfected my method over time. Even so, I did some additional research to find out if there was a better solution. Indeed, I owe thanks to various community members who advised me on optimising my code. The community also offered some different ways of parsing the text file. Some of them were clever and exciting. My personal favourite was this one. I presented my sample problem and solution at the forums below:
Reddit post
Stackoverflow post
Code review post
If your problem is even more complex and regular expressions don’t cut it, then the next step would be to consider parsing libraries. Here are a couple of places to start with:
Parsing Horrible Things with Python:
A PyCon lecture by Erik Rose looking at the pros and cons of various parsing libraries.
Parsing in Python: Tools and Libraries:
Tools and libraries that allow you to create parsers when regular expressions are not enough.
Now that you understand how difficult and annoying it can be to parse text files, if you ever find yourselves in the privileged position of choosing a file format, choose it with care. Here are Stanford’s best practices for file formats.
I’d be lying if I said I was delighted with my parsing method, but I’m not aware of another way, of quickly parsing a text file, that is as beginner friendly as what I’ve presented above. If you know of a better solution, I’m all ears! I have hopefully given you a good starting point for parsing a file in Python! I spent a couple of months trying lots of different methods and writing some insanely unreadable code before I finally figured it out and now I don’t think twice about parsing a file. So, I hope I have been able to save you some time. Have fun parsing text with python!
Frequently Asked Questions about parsing a file in python
How do you parse a file in Python?
Parsing text in complex format using regular expressionsStep 1: Understand the input format. 123. … Step 2: Import the required packages. We will need the Regular expressions module and the pandas package. … Step 3: Define regular expressions. … Step 4: Write a line parser. … Step 5: Write a file parser. … Step 6: Test the parser.Jan 7, 2018
What is file parsing?
1) File Parsing. Definition: Parse essentially means to ”resolve (a sentence) into its component parts and describe their syntactic roles”. … [Google Dictionary]File parsing in computer language means to give a meaning to the characters of a text file as per the formal grammar.Jan 9, 2019
What does it mean to parse a file in Python?
So “parsing” or “parsed” means to make something understandable. For programming this is converting information into a format that’s easier to work with. So the phrase “parsed as a string” means we are taking the data from the csv file and turning it into strings.Jan 23, 2020