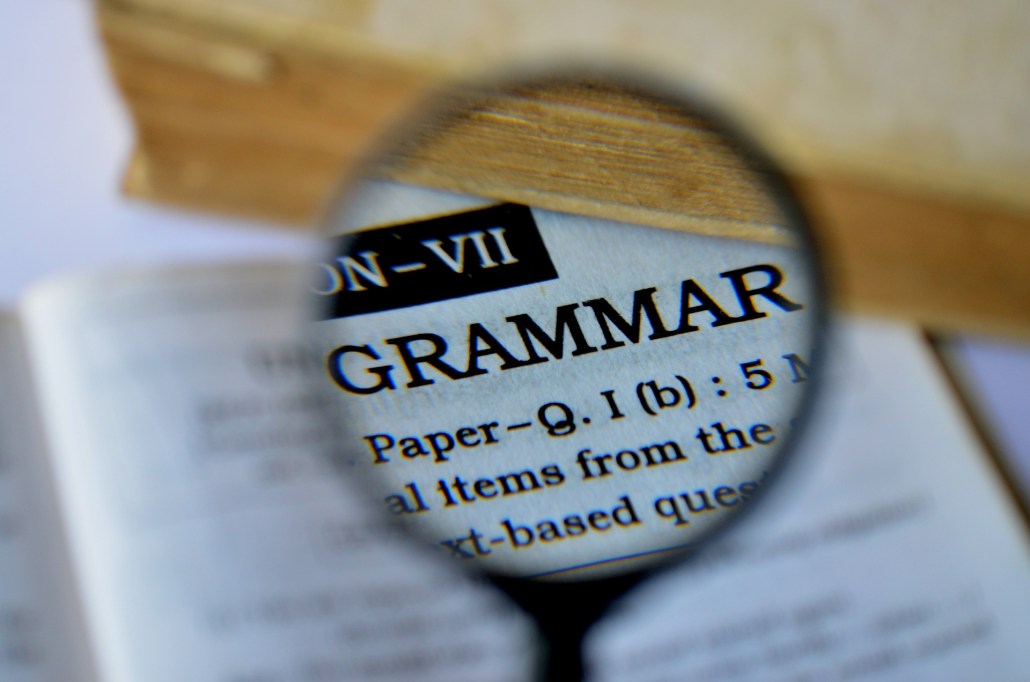
Parser Parse Python
parser — dateutil 2.8.2 documentation
dateutil
This module offers a generic date/time string parser which is able to parse
most known formats to represent a date and/or time.
This module attempts to be forgiving with regards to unlikely input formats,
returning a datetime object even for dates which are ambiguous. If an element
of a date/time stamp is omitted, the following rules are applied:
If AM or PM is left unspecified, a 24-hour clock is assumed, however, an hour
on a 12-hour clock (0 <= hour <= 12) must be specified if AM or PM is
specified.
If a time zone is omitted, a timezone-naive datetime is returned.
If any other elements are missing, they are taken from the
time object passed to the parameter default. If this
results in a day number exceeding the valid number of days per month, the
value falls back to the end of the month.
Additional resources about date/time string formats can be found below:
A summary of the international standard date and time notation
W3C Date and Time Formats
Time Formats (Planetary Rings Node)
CPAN ParseDate module
Java SimpleDateFormat Class
Functions¶
(parserinfo=None, **kwargs)[source]¶
Parse a string in one of the supported formats, using the
parserinfo parameters.
Parameters:
timestr – A string containing a date/time stamp.
parserinfo – A parserinfo object containing parameters for the parser.
If None, the default arguments to the parserinfo
constructor are used.
The **kwargs parameter takes the following keyword arguments:
default – The default datetime object, if this is a datetime object and not
None, elements specified in timestr replace elements in the
default object.
ignoretz – If set True, time zones in parsed strings are ignored and a naive
datetime object is returned.
tzinfos – Additional time zone names / aliases which may be present in the
string. This argument maps time zone names (and optionally offsets
from those time zones) to time zones. This parameter can be a
dictionary with timezone aliases mapping time zone names to time
zones or a function taking two parameters (tzname and
tzoffset) and returning a time zone.
The timezones to which the names are mapped can be an integer
offset from UTC in seconds or a tzinfo object.
>>> from import parse
>>> from import gettz
>>> tzinfos = {“BRST”: -7200, “CST”: gettz(“America/Chicago”)}
>>> parse(“2012-01-19 17:21:00 BRST”, tzinfos=tzinfos)
time(2012, 1, 19, 17, 21, tzinfo=tzoffset(u’BRST’, -7200))
>>> parse(“2012-01-19 17:21:00 CST”, tzinfos=tzinfos)
time(2012, 1, 19, 17, 21,
tzinfo=tzfile(‘/usr/share/zoneinfo/America/Chicago’))
This parameter is ignored if ignoretz is set.
dayfirst – Whether to interpret the first value in an ambiguous 3-integer date
(e. g. 01/05/09) as the day (True) or month (False). If
yearfirst is set to True, this distinguishes between YDM and
YMD. If set to None, this value is retrieved from the current
parserinfo object (which itself defaults to False).
yearfirst – Whether to interpret the first value in an ambiguous 3-integer date
(e. 01/05/09) as the year. If True, the first number is taken to
be the year, otherwise the last number is taken to be the year. If
this is set to None, the value is retrieved from the current
fuzzy – Whether to allow fuzzy parsing, allowing for string like “Today is
January 1, 2047 at 8:21:00AM”.
fuzzy_with_tokens – If True, fuzzy is automatically set to True, and the parser
will return a tuple where the first element is the parsed
time datetimestamp and the second element is
a tuple containing the portions of the string which were ignored:
>>> parse(“Today is January 1, 2047 at 8:21:00AM”, fuzzy_with_tokens=True)
(time(2047, 1, 1, 8, 21), (u’Today is ‘, u’ ‘, u’at ‘))
Returns:Returns a time object or, if the
fuzzy_with_tokens option is True, returns a tuple, the
first element being a time object, the second
a tuple containing the fuzzy tokens.
Raises:
ParserError – Raised for invalid or unknown string formats, if the provided
tzinfo is not in a valid format, or if an invalid date would
be created.
OverflowError – Raised if the parsed date exceeds the largest valid C integer on
your system.
classmethod oparse(dt_str)¶
Parse an ISO-8601 datetime string into a time.
An ISO-8601 datetime string consists of a date portion, followed
optionally by a time portion – the date and time portions are separated
by a single character separator, which is T in the official
standard. Incomplete date formats (such as YYYY-MM) may not be
combined with a time portion.
Supported date formats are:
Common:
YYYY
YYYY-MM or YYYYMM
YYYY-MM-DD or YYYYMMDD
Uncommon:
YYYY-Www or YYYYWww – ISO week (day defaults to 0)
YYYY-Www-D or YYYYWwwD – ISO week and day
The ISO week and day numbering follows the same logic as
().
Supported time formats are:
hh
hh:mm or hhmm
hh:mm:ss or hhmmss
(Up to 6 sub-second digits)
Midnight is a special case for hh, as the standard supports both
00:00 and 24:00 as a representation. The decimal separator can be
either a dot or a comma.
Caution
Support for fractional components other than seconds is part of the
ISO-8601 standard, but is not currently implemented in this parser.
Supported time zone offset formats are:
Z (UTC)
±HH:MM
±HHMM
±HH
Offsets will be represented as objects,
with the exception of UTC, which will be represented as
Time zone offsets equivalent to UTC (such
as +00:00) will also be represented as
Parameters:dt_str – A string or stream containing only an ISO-8601 datetime string
Returns:Returns a time representing the string.
Unspecified components default to their lowest value.
Warning
As of version 2. 7. 0, the strictness of the parser should not be
considered a stable part of the contract. Any valid ISO-8601 string
that parses correctly with the default settings will continue to
parse correctly in future versions, but invalid strings that
currently fail (e. 2017-01-01T00:00+00:00:00) are not
guaranteed to continue failing in future versions if they encode
a valid date.
New in version 2. 0.
Classes¶
class (dayfirst=False, yearfirst=False)[source]¶
Class which handles what inputs are accepted. Subclass this to customize
the language and acceptable values for each parameter.
yearfirst is set to True, this distinguishes between YDM
and YMD. Default is False.
(e. If True, the first number is taken
to be the year, otherwise the last number is taken to be the year.
Default is False.
AMPM = [(‘am’, ‘a’), (‘pm’, ‘p’)]¶
HMS = [(‘h’, ‘hour’, ‘hours’), (‘m’, ‘minute’, ‘minutes’), (‘s’, ‘second’, ‘seconds’)]¶
JUMP = [‘ ‘, ‘. ‘, ‘, ‘, ‘;’, ‘-‘, ‘/’, “‘”, ‘at’, ‘on’, ‘and’, ‘ad’, ‘m’, ‘t’, ‘of’, ‘st’, ‘nd’, ‘rd’, ‘th’]¶
MONTHS = [(‘Jan’, ‘January’), (‘Feb’, ‘February’), (‘Mar’, ‘March’), (‘Apr’, ‘April’), (‘May’, ‘May’), (‘Jun’, ‘June’), (‘Jul’, ‘July’), (‘Aug’, ‘August’), (‘Sep’, ‘Sept’, ‘September’), (‘Oct’, ‘October’), (‘Nov’, ‘November’), (‘Dec’, ‘December’)]¶
PERTAIN = [‘of’]¶
TZOFFSET = {}¶
UTCZONE = [‘UTC’, ‘GMT’, ‘Z’, ‘z’]¶
WEEKDAYS = [(‘Mon’, ‘Monday’), (‘Tue’, ‘Tuesday’), (‘Wed’, ‘Wednesday’), (‘Thu’, ‘Thursday’), (‘Fri’, ‘Friday’), (‘Sat’, ‘Saturday’), (‘Sun’, ‘Sunday’)]¶
ampm(name)[source]¶
convertyear(year, century_specified=False)[source]¶
Converts two-digit years to year within [-50, 49]
range of self. _year (current local time)
hms(name)[source]¶
jump(name)[source]¶
month(name)[source]¶
pertain(name)[source]¶
tzoffset(name)[source]¶
utczone(name)[source]¶
validate(res)[source]¶
weekday(name)[source]¶
Warnings and Exceptions¶
class [source]¶
Exception subclass used for any failure to parse a datetime string.
This is a subclass of ValueError, and should be raised any time
earlier versions of dateutil would have raised ValueError.
New in version 2. 8. 1.
Raised when the parser finds a timezone it cannot parse into a tzinfo.
New in version 2. 0.
Python dateutil.parser.parse parses month first, not day – Stack …
You asked, ‘How can I tell the code that the format is ‘
Since you have already imported dateutil then most direct answer might be to specify the format of the date string but this is quite ugly code:
>>> (date_string, ‘%d. %m. %Y’)
time(2015, 1, 5, 0, 0)
We can see an obvious alternative embedded in the code. You could use that directly.
>>> from datetime import datetime
>>> rptime(date_string, ‘%d. %Y’)
There are also some newer alternative libraries that offer methods and properties aplenty.
Simplest to use in this case would be arrow:
>>> import arrow
>>> (date_string, ”)
Although I find the formatting for arrow easier to remember, pendulum uses Python’s old formatting system which might save you having to learn arrow’s.
>>> import pendulum
Python Parser | Working of Python Parse with different Examples
Introduction to Python Parser
In this article, parsing is defined as the processing of a piece of python program and converting these codes into machine language. In general, we can say parse is a command for dividing the given program code into a small piece of code for analyzing the correct syntax. In Python, there is a built-in module called parse which provides an interface between the Python internal parser and compiler, where this module allows the python program to edit the small fragments of code and create the executable program from this edited parse tree of python code. In Python, there is another module known as argparse to parse command-line options.
Working of Python Parse with Examples
In this article, Python parser is mainly used for converting data in the required format, this conversion process is known as parsing. As in many different applications data obtained can have different data formats and these formats might not be suitable to the particular application and here comes the use of parser that means parsing is necessary for such situations. Therefore, parsing is generally defined as the conversion of data with one format to some other format is known as parsing. In parser consists of two parts lexer and a parser and in some cases only parsers are used.
Python parsing is done using various ways such as the use of parser module, parsing using regular expressions, parsing using some string methods such as split() and strip(), parsing using pandas such as reading CSV file to text by using, etc. There is also a concept of argument parsing which means in Python, we have a module named argparse which is used for parsing data with one or more arguments from the terminal or command-line. There are other different modules when working with argument parsings such as getopt, sys, and argparse modules. Now let us below the demonstration for Python parser. In Python, the parser can also be created using few tools such as parser generators and there is a library known as parser combinators that are used for creating parsers.
Now let us see in the below example of how the parser module is used for parsing the given expressions.
Example #1
Code:
import parser
print(“Program to demonstrate parser module in Python”)
print(“\n”)
exp = “5 + 8”
print(“The given expression for parsing is as follows:”)
print(exp)
print(“Parsing of given expression results as: “)
st = (exp)
print(st)
print(“The parsed object is converted to the code object”)
code = mpile()
print(code)
print(“The evaluated result of the given expression is as follows:”)
res = eval(code)
print(res)
Output:
In the above program, we first need to import the parser module, and then we have declared expression to calculate, and to parse this expression we have to use a () function. Then we can evaluate the given expression using eval() function.
In Python, sometimes we get data that consists of date-time format which would be in CSV format or text format. So to parse such formats in proper date-time formats Python provides parse_dates() function. Suppose we have a CSV file that contains data and the data time details are separated with a comma which makes it difficult for reading therefore for such cases we use parse_dates() but before that, we have to import pandas as this function is provided by pandas.
In Python, we can also parse command-line options and arguments using an argparse module which is very user friendly for the command-line interface. Suppose we have Unix commands to execute through python command-line interface such as ls which list all the directories in the current drive and it will take many different arguments also therefore to create such command-line interface we use an argparse module in Python. Therefore, to create a command-line interface in Python we need to do the following; firstly, we have to import an argparse module, then we create an object for holding arguments using ArgumentParser() through the argparse module, later we can add arguments the ArgumentParser() object that will be created and we can run any commands in Python command line. Note as running any commands is not free other than the help command. So here is a small piece of code for how to write the python code to create a command line interface using an argparse module.
import argparse
Now we have created an object using ArgumentParser() and then we can parse the arguments using rse_args() function.
parser = gumentParser()
rse_args()
To add the arguments we can use add_argument() along with passing the argument to this function such as d_argument(“ ls ”). So let us see a small example below.
Example #2
d_argument(“ls”)
args = rse_args()
print()
So in the above program, we can see the screenshot of the output as we cannot use any other commands so it will give an error but when we have an argparse module then we can run the commands in python shell as follows:
$ python –help
usage: [-h] echo
Positional Arguments:
echo
Optional Arguments:
-h, –helpshow this help message and exit
$ python Educba
Educba
Conclusion
In this article, we conclude that Python provides a parsing concept. In this article, we saw that the parsing process is very simple which in general is the process of parting the large string of one type of format for converting this format to another required format is known as parsing. This is done in many different ways in Python using python string methods such as split() or strip(), using python pandas for converting CSV files to text format. In this, we saw that we can even use a parser module for using it as a command-line interface where we can run the commands easily using the argparse module in Python. In the above, we saw how to use argparse and how can we run the commands in Python terminal.
Recommended Articles
This is a guide to Python Parser. Here we also discuss the introduction and working of python parser along with different examples and its code implementation. You may also have a look at the following articles to learn more –
Python Timezone
Python NameError
Python OS Module
Python Event Loop
Frequently Asked Questions about parser parse python
What does parser parse do in Python?
Introduction to Python Parser. In this article, parsing is defined as the processing of a piece of python program and converting these codes into machine language. In general, we can say parse is a command for dividing the given program code into a small piece of code for analyzing the correct syntax.
What is Python parser?
The parser module provides an interface to Python’s internal parser and byte-code compiler. The primary purpose for this interface is to allow Python code to edit the parse tree of a Python expression and create executable code from this. … The parser module is deprecated and will be removed in future versions of Python.
How do you parse in Python?
Parsing text in complex format using regular expressionsStep 1: Understand the input format. 123. … Step 2: Import the required packages. We will need the Regular expressions module and the pandas package. … Step 3: Define regular expressions. … Step 4: Write a line parser. … Step 5: Write a file parser. … Step 6: Test the parser.Jan 7, 2018