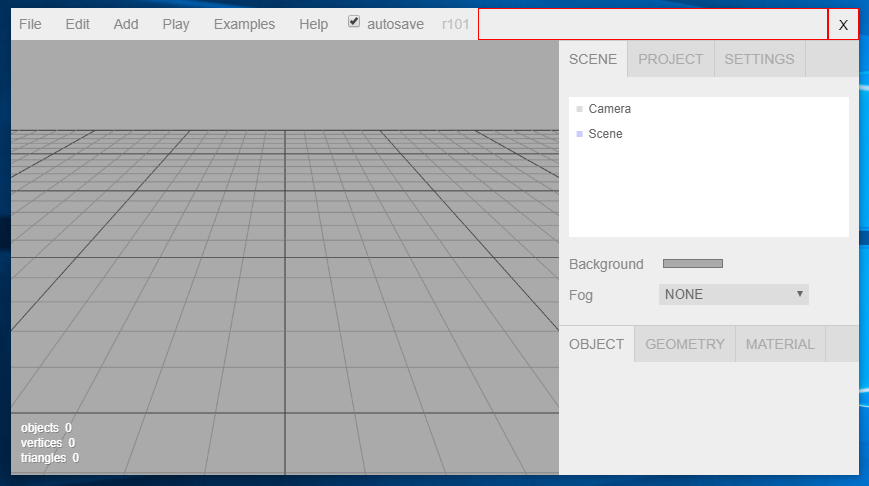
Node Js Parse Html
node-html-parser – npm
Fast HTML Parser is a very fast HTML parser. Which will generate a simplified
DOM tree, with element query support.
Per the design, it intends to parse massive HTML files in lowest price, thus the
performance is the top priority. For this reason, some malformatted HTML may not
be able to parse correctly, but most usual errors are covered (eg. HTML4 style
no closing
Install
npm install –save node-html-parser
Note: when using Fast HTML Parser in a Typescript project the minimum Typescript version supported is ^4. 1. 2.
Performance
Faster than htmlparser2!
htmlparser:26. 7111 ms/file ± 170. 066
cheerio:24. 2480 ms/file ± 17. 1711
parse5:13. 7239 ms/file ± 8. 68561
high5:7. 75466 ms/file ± 5. 33549
htmlparser2:5. 27376 ms/file ± 8. 68456
node-html-parser:2. 85768 ms/file ± 2. 87784
Tested with htmlparser-benchmark.
Usage
import { parse} from ‘node-html-parser’;
const root = parse(‘
- Hello World
‘);
(ructure);
// ul#list
// li
// #text
(root. querySelector(‘#list’));
// { tagName: ‘ul’,
// rawAttrs: ‘id=”list”‘,
// childNodes:
// [ { tagName: ‘li’,
// rawAttrs: ”,
// childNodes: [Object],
// classNames: []}],
// id: ‘list’,
// classNames: []}
(String());
//
- Hello World
t_content(‘
‘);
String(); //
var HTMLParser = require(‘node-html-parser’);
var root = (‘
- Hello World
‘);
Global Methods
parse(data[, options])
Parse given data, and return root of the generated DOM.
data, data to parse
options, parse options
{
lowerCaseTagName: false, // convert tag name to lower case (hurt performance heavily)
comment: false, // retrieve comments (hurt performance slightly)
blockTextElements: {
script: true, // keep text content when parsing
noscript: true, // keep text content when parsing
style: true, // keep text content when parsing
pre: true // keep text content when parsing}}
valid(data[, options])
Parse given data, return true if the givent data is valid, and return false if not.
HTMLElement Methods
HTMLElement#trimRight()
Trim element from right (in block) after seeing pattern in a TextNode.
HTMLElement#removeWhitespace()
Remove whitespaces in this sub tree.
HTMLElement#querySelectorAll(selector)
Query CSS selector to find matching nodes.
Note: Full css3 selector supported since v3. 0. 0.
HTMLElement#querySelector(selector)
Query CSS Selector to find matching node.
HTMLElement#getElementsByTagName(tagName)
Get all elements with the specified tagName.
Note: * for all elements.
HTMLElement#closest(selector)
Query closest element by css selector.
HTMLElement#appendChild(node)
Append a child node to childNodes
HTMLElement#insertAdjacentHTML(where, html)
parses the specified text as HTML and inserts the resulting nodes into the DOM tree at a specified position.
HTMLElement#setAttribute(key: string, value: string)
Set value to key attribute.
HTMLElement#setAttributes(attrs: Record
Set attributes of the element.
HTMLElement#removeAttribute(key: string)
Remove key attribute.
HTMLElement#getAttribute(key: string)
Get key attribute.
HTMLElement#exchangeChild(oldNode: Node, newNode: Node)
Exchanges given child with new child.
HTMLElement#removeChild(node: Node)
Remove child node.
HTMLElement#toString()
Same as outerHTML
HTMLElement#set_content(content: string | Node | Node[])
Set content. Notice: Do not set content of the root node.
HTMLElement#remove()
Remove current element.
HTMLElement#replaceWith(.. (string | Node)[])
Replace current element with other node(s).
HTMLElement#classList
Add class name.
place(old: string, new: string)
Replace class name with another one.
()
Remove class name.
(className: string):void
Toggle class.
ntains(className: string): boolean
Get if contains
get class names
HTMLElement Properties
HTMLElement#text
Get unescaped text value of current node and its children. Like innerText.
(slow for the first time)
HTMLElement#rawText
Get escaped (as-it) text value of current node and its children. May have
& in it. (fast)
HTMLElement#tagName
Get tag name of HTMLElement. Notice: the returned value would be an uppercase string.
HTMLElement#structuredText
Get structured Text
HTMLElement#structure
Get DOM structure
HTMLElement#firstChild
Get first child node
HTMLElement#lastChild
Get last child node
HTMLElement#innerHTML
Set or Get innerHTML.
HTMLElement#outerHTML
Get outerHTML.
HTMLElement#nextSibling
Returns a reference to the next child node of the current element’s parent.
HTMLElement#nextElementSibling
Returns a reference to the next child element of the current element’s parent.
HTMLElement#textContent
Get or Set textContent of current element, more efficient than set_content.
HTMLElement#attributes
Get all attributes of current element. Notice: do not try to change the returned value.
HTMLElement#range
Corresponding source code start and end indexes (ie [ 0, 40])
Web Scraping and Parsing HTML with Node.js and Cheerio
The internet has a wide variety of information for human consumption. But this data is often difficult to access programmatically if it doesn’t come in the form of a dedicated REST API. With tools like Cheerio, you can scrape and parse this data directly from web pages to use for your projects and applications.
Let’s use the example of scraping MIDI data to train a neural network that can generate classic Nintendo-sounding music. In order to do this, we’ll need a set of music from old Nintendo games. Using Cheerio we can scrape this data from the Video Game Music Archive.
Getting started and setting up dependencies
Before moving on, you will need to make sure you have an up to date version of and npm installed.
Navigate to the directory where you want this code to live and run the following command in your terminal to create a package for this project:
The –yes argument runs through all of the prompts that you would otherwise have to fill out or skip. Now we have a for our app.
For making HTTP requests to get data from the web page we will use the Got library, and for parsing through the HTML we’ll use Cheerio.
Run the following command in your terminal to install these libraries:
npm install got@10. 4. 0 cheerio@1. 0. 0-rc. 3
Cheerio implements a subset of core jQuery, making it a familiar tool to use for lots of JavaScript developers. Let’s dive into how to use it.
Using Got to retrieve data to use with Cheerio
First let’s write some code to grab the HTML from the web page, and look at how we can start parsing through it. The following code will send a GET request to the web page we want, and will create a Cheerio object with the HTML from that page. We’ll name it $ following the infamous jQuery convention:
const fs = require(‘fs’);
const cheerio = require(‘cheerio’);
const got = require(‘got’);
const vgmUrl= ”;
got(vgmUrl)(response => {
const $ = ();
($(‘title’)[0]);})(err => {
(err);});
With this $ object, you can navigate through the HTML and retrieve DOM elements for the data you want, in the same way that you can with jQuery. For example, $(‘title’) will get you an array of objects corresponding to every
Getting familiar with Cheerio
When you have an object corresponding to an element in the HTML you’re parsing through, you can do things like navigate through its children, parent and sibling elements. The child of this
If you want to get more specific in your query, there are a variety of selectors you can use to parse through the HTML. Two of the most common ones are to search for elements by class or ID. If you wanted to get a div with the ID of “menu” you would run $(‘#menu’) and if you wanted all of the columns in the table of VGM MIDIs with the “header” class, you’d do $(”)
What we want on this page are the hyperlinks to all of the MIDI files we need to download. We can start by getting every link on the page using $(‘a’). Add the following to your code in
$(‘a’)((i, link) => {
const href =;
(href);});})(err => {
This code logs the URL of every link on the page. Notice that we’re able to look through all elements from a given selector using the () function. Iterating through every link on the page is great, but we’re going to need to get a little more specific than that if we want to download all of the MIDI files.
Filtering through HTML elements with Cheerio
Before writing more code to parse the content that we want, let’s first take a look at the HTML that’s rendered by the browser. Every web page is different, and sometimes getting the right data out of them requires a bit of creativity, pattern recognition, and experimentation.
Our goal is to download a bunch of MIDI files, but there are a lot of duplicate tracks on this webpage, as well as remixes of songs. We only want one of each song, and because our ultimate goal is to use this data to train a neural network to generate accurate Nintendo music, we won’t want to train it on user-created remixes.
When you’re writing code to parse through a web page, it’s usually helpful to use the developer tools available to you in most modern browsers. If you right-click on the element you’re interested in, you can inspect the HTML behind that element to get more insight.
With Cheerio, you can write filter functions to fine-tune which data you want from your selectors. These functions loop through all elements for a given selector and return true or false based on whether they should be included in the set or not.
If you looked through the data that was logged in the previous step, you might have noticed that there are quite a few links on the page that have no href attribute, and therefore lead nowhere. We can be sure those are not the MIDIs we are looking for, so let’s write a short function to filter those out as well as making sure that elements which do contain a href element lead to a file:
const isMidi = (i, link) => {
// Return false if there is no href attribute.
if(typeof === ‘undefined’) { return false}
return (”);};
Now we have the problem of not wanting to download duplicates or user generated remixes. For this we can use regular expressions to make sure we are only getting links whose text has no parentheses, as only the duplicates and remixes contain parentheses:
const noParens = (i, link) => {
// Regular expression to determine if the text has parentheses.
const parensRegex = /^((?! \(). )*$/;
return (ildren[0]);};
Try adding these to your code in
$(‘a’)(isMidi)(noParens)((i, link) => {
(href);});});
Run this code again and it should only be printing files.
Downloading the MIDI files we want from the webpage
Now that we have working code to iterate through every MIDI file that we want, we have to write code to download all of them.
In the callback function for looping through all of the MIDI links, add this code to stream the MIDI download into a local file, complete with error checking:
const fileName =;
(`${vgmUrl}/${fileName}`)
(‘error’, err => { (err); (`Error on ${vgmUrl}/${fileName}`)})
(eateWriteStream(`MIDIs/${fileName}`))
(‘finish’, () => (`Finished ${fileName}`));});
Run this code from a directory where you want to save all of the MIDI files, and watch your terminal screen display all 2230 MIDI files that you downloaded (at the time of writing this). With that, we should be finished scraping all of the MIDI files we need.
Go through and listen to them and enjoy some Nintendo music!
The vast expanse of the World Wide Web
Now that you can programmatically grab things from web pages, you have access to a huge source of data for whatever your projects need. One thing to keep in mind is that changes to a web page’s HTML might break your code, so make sure to keep everything up to date if you’re building applications on top of this. You might want to also try comparing the functionality of the jsdom library with other solutions by following tutorials for web scraping using jsdom and headless browser scripting using Puppeteer or a similar library called Playwright.
If you’re looking for something to do with the data you just grabbed from the Video Game Music Archive, you can try using Python libraries like Magenta to train a neural network with it.
I’m looking forward to seeing what you build. Feel free to reach out and share your experiences or ask any questions.
Email:
Twitter: @Sagnewshreds
Github: Sagnew
Twitch (streaming live code): Sagnewshreds

Parsing HTML in Node.js with Cheerio – LogRocket Blog
Introduction
Traditionally, does not let you parse and manipulate markups because it executes code outside of the browser. In this article, we will be exploring Cheerio, an open source JavaScript library designed specifically for this purpose.
Cheerio provides a flexible and lean implementation of jQuery, but it’s designed for the server. Manipulating and rendering markup with Cheerio is incredibly fast because it works with a concise and simple markup (similar to jQuery). And apart from parsing HTML, Cheerio works excellently well with XML documents, too.
Goals
This tutorial assumes no prior knowledge of Cheerio, and will cover the following areas:
Installing Cheerio in a project
Understanding Cheerio (loading, selectors, DOM manipulation, and rendering)
Building a sample application (FeatRocket) that scrapes LogRocket featured articles and logs them to the console
Prerequisites
To complete this tutorial, you will need:
Basic familiarity with HTML, CSS, and the DOM
Familiarity with npm and
Familiarity working with the command line and text editors
Setting up Cheerio
Cheerio can be used on any ES6+, TypeScript, and project, but for this article, we will focus on
To get started, we need to run the npm init -y command, which will generate a new file with its contents like below:
{
“name”: “cheerio-sample”,
“version”: “1. 0. 0”,
“description”: “”,
“main”: “”,
“scripts”: {
“test”: “echo \”Error: no test specified\”” && exit 1″”}