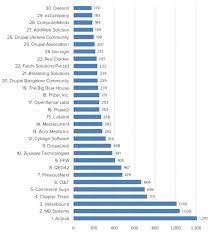
Es Proxy
abutaha/aws-es-proxy – GitHub
aws-es-proxy is a small web server application sitting between your HTTP client (browser, curl, etc… ) and Amazon Elasticsearch service. It will sign your requests using latest AWS Signature Version 4 before sending the request to Amazon Elasticsearch. When response is back from Amazon Elasticsearch, this response will be sent back to your HTTP client.
Kibana requests are also signed automatically.
Installation
Download binary executable
aws-es-proxy has single executable binaries for Linux, Mac and Windows.
Download the latest aws-es-proxy release.
Docker
There is an official docker image available for aws-es-proxy. To run the image:
# v0. 9 and newer (latest always point to the latest release):
docker run –rm -v ~/ -p 9200:9200 abutaha/aws-es-proxy:v1. 0 -endpoint -listen 0. 0. 0:9200
v. 08:
docker run –rm -it abutaha/aws-es-proxy. /aws-es-proxy -endpoint
To expose a port number other than the default 9200, pass an environment variable of PORT_NUM to docker with the port number you wish to expose for your service.
Via homebrew
brew install aws-es-proxy
Build from Source
Dependencies:
go1. 14+
#requires go1. 14
go build
Configuring Credentials
Before using aws-es-proxy, ensure that you’ve configured your AWS IAM user credentials. The best way to configure credentials on a development machine is to use the ~/ file, which might look like:
[default]
aws_access_key_id = AKID1234567890
aws_secret_access_key = MY-SECRET-KEY
Alternatively, you can set the following environment variables:
export AWS_ACCESS_KEY_ID=AKID1234567890
export AWS_SECRET_ACCESS_KEY=MY-SECRET-KEY
aws-es-proxy also supports IAM roles. To use IAM roles, you need to modify your Amazon Elasticsearch access policy to allow access from that role. Below is an Amazon Elasticsearch access policy example allowing access from any EC2 instance with an IAM role called ec2-aws-elasticsearch.
{
“Version”: “2012-10-17”,
“Statement”: [
“Effect”: “Allow”,
“Principal”: {
“AWS”: “arn:aws:iam::012345678910:role/ec2-aws-elasticsearch”},
“Action”: “es:*”,
“Resource”: “arn:aws:es:eu-west-1:012345678910:domain/test-es-domain/*”}]}
Usage example:
You can use either argument -endpoint OR environment variable ENDPOINT to specify AWS ElasticSearch endpoint.. /aws-es-proxy -endpoint Listening on 127. 1:9200
export ENDPOINT=. /aws-es-proxy -listen 10. 1:9200 -verbose
Listening on 10. 1:9200
aws-es-proxy listens on 127. 1:9200 if no additional argument is provided. You can change the IP and Port passing the argument -listen. /aws-es-proxy -listen:8080 -endpoint…. 1:9200 -endpoint…
By default, aws-es-proxy will not display any message in the console. However, it has the ability to print requests being sent to Amazon Elasticsearch, and the duration it takes to receive the request back. This can be enabled using the option -verbose. /aws-es-proxy -verbose…
Listening on 127. 1:9200
2016/10/31 19:48:23 -> GET / 200 1. 054s
2016/10/31 19:48:30 -> GET /_cat/indices? v 200 0. 199s
2016/10/31 19:48:37 -> GET /_cat/shards? v 200 0. 196s
2016/10/31 19:48:49 -> GET /_cat/allocation? v 200 0. 179s
2016/10/31 19:49:10 -> PUT /my-test-index 200 0. 347s
For a full list of available options, use -h:. /aws-es-proxy -h
Usage of. /aws-es-proxy:
-auth
Require HTTP Basic Auth
-debug
Print debug messages
-endpoint string
Amazon ElasticSearch Endpoint (e. g:)
-listen string
Local TCP port to listen on (default “127. 1:9200”)
-log-to-file
Log user requests and ElasticSearch responses to files
-no-sign-reqs
Disable AWS Signature v4
-password string
HTTP Basic Auth Password
-pretty
Prettify verbose and file output
-realm string
Authentication Required
-remote-terminate
Allow HTTP remote termination
-timeout int
Set a request timeout to ES. Specify in seconds, defaults to 15 (default 15)
-username string
HTTP Basic Auth Username
-verbose
Print user requests
-version
Print aws-es-proxy version
Using HTTP Clients
After you run aws-es-proxy, you can now open your Web browser on localhost:9200. Everything should be working as you have your own instance of ElasticSearch running on port 9200.
To access Kibana, use localhost:9200/_plugin/kibana/app/kibana
Proxy – JavaScript – MDN Web Docs
The Proxy object enables you to create a proxy for another object, which can intercept and redefine fundamental operations for that scriptionA Proxy is created with two parameters:
target: the original object which you want to proxy
handler: an object that defines which operations will be intercepted and how to redefine intercepted operations.
For example, this code defines a simple target with just two properties, and an even simpler handler with no properties:
const target = {
message1: “hello”,
message2: “everyone”};
const handler1 = {};
const proxy1 = new Proxy(target, handler1);
Because the handler is empty, this proxy behaves just like the original target:
(ssage1); // hello
(ssage2); // everyone
To customise the proxy, we define functions on the handler object:
const handler2 = {
get: function(target, prop, receiver) {
return “world”;}};
const proxy2 = new Proxy(target, handler2);
Here we’ve provided an implementation of the get() handler, which intercepts attempts to access properties in the target.
Handler functions are sometimes called traps, presumably because they trap calls to the target object. The very simple trap in handler2 above redefines all property accessors:
(ssage1); // world
(ssage2); // world
With the help of the Reflect class we can give some accessors the original behavior and redefine others:
const handler3 = {
get: function (target, prop, receiver) {
if (prop === “message2”) {
return “world”;}
return (guments);}, };
const proxy3 = new Proxy(target, handler3);
Constructor
Proxy()
Creates a new Proxy object.
Static methodsExamplesBasic exampleIn this simple example, the number 37 gets returned as the default value when the property name is not in the object. It is using the get() handler.
const handler = {
get: function(obj, prop) {
return prop in obj?
obj[prop]:
37;}};
const p = new Proxy({}, handler);
p. a = 1;
p. b = undefined;
(p. a, p. b);
// 1, undefined
(‘c’ in p, p. c);
// false, 37
No-op forwarding proxyIn this example, we are using a native JavaScript object to which our proxy will forward all operations that are applied to it.
const target = {};
const p = new Proxy(target, {});
p. a = 37;
// operation forwarded to the target
(target. a);
// 37
// (The operation has been properly forwarded! )
Note that while this “no-op” works for JavaScript objects, it does not work for native browser objects like DOM lidationWith a Proxy, you can easily validate the passed value for an object. This example uses the set() handler.
let validator = {
set: function(obj, prop, value) {
if (prop === ‘age’) {
if (! Integer(value)) {
throw new TypeError(‘The age is not an integer’);}
if (value > 200) {
throw new RangeError(‘The age seems invalid’);}}
// The default behavior to store the value
obj[prop] = value;
// Indicate success
return true;}};
const person = new Proxy({}, validator);
= 100;
(); // 100
= ‘young’; // Throws an exception
= 300; // Throws an exception
Extending constructorA function proxy could easily extend a constructor with a new constructor. This example uses the construct() and apply() handlers.
function extend(sup, base) {
var descriptor = tOwnPropertyDescriptor(
ototype, ‘constructor’);
ototype = (ototype);
var handler = {
construct: function(target, args) {
var obj = (ototype);
(target, obj, args);
return obj;},
apply: function(target, that, args) {
(that, args);
(that, args);}};
var proxy = new Proxy(base, handler);
= proxy;
fineProperty(ototype, ‘constructor’, descriptor);
return proxy;}
var Person = function(name) {
= name;};
var Boy = extend(Person, function(name, age) {
= age;});
= ‘M’;
var Peter = new Boy(‘Peter’, 13);
(); // “M”
(); // “Peter”
(); // 13
Manipulating DOM nodesSometimes you want to toggle the attribute or class name of two different elements. Here’s how using the set() handler.
let view = new Proxy({
selected: null},
{
set: function(obj, prop, newval) {
let oldval = obj[prop];
if (prop === ‘selected’) {
if (oldval) {
tAttribute(‘aria-selected’, ‘false’);}
if (newval) {
tAttribute(‘aria-selected’, ‘true’);}}
obj[prop] = newval;
return true;}});
let i1 = lected = tElementById(‘item-1’); //giving error here, i1 is null
(tAttribute(‘aria-selected’));
// ‘true’
let i2 = lected = tElementById(‘item-2’);
// ‘false’
Note: even if selected:! null, then giving tAttribute is not a function
Value correction and an extra propertyThe products proxy object evaluates the passed value and converts it to an array if needed. The object also supports an extra property called latestBrowser both as a getter and a setter.
let products = new Proxy({
browsers: [‘Internet Explorer’, ‘Netscape’]},
// An extra property
if (prop === ‘latestBrowser’) {
return owsers[ – 1];}
// The default behavior to return the value
return obj[prop];},
(value);
return true;}
// Convert the value if it is not an array
if (typeof value === ‘string’) {
value = [value];}
(owsers);
// [‘Internet Explorer’, ‘Netscape’]
owsers = ‘Firefox’;
// pass a string (by mistake)
// [‘Firefox’] <- no problem, the value is an array
testBrowser = 'Chrome';
// ['Firefox', 'Chrome']
(testBrowser);
// 'Chrome'
Finding an array item object by its propertyThis proxy extends an array with some utility features. As you see, you can flexibly "define" properties without using fineProperties(). This example can be adapted to find a table row by its cell. In that case, the target will be
let products = new Proxy([
{ name: 'Firefox', type: 'browser'},
{ name: 'SeaMonkey', type: 'browser'},
{ name: 'Thunderbird', type: 'mailer'}],
// The default behavior to return the value; prop is usually an integer
if (prop in obj) {
return obj[prop];}
// Get the number of products; an alias of
if (prop === 'number') {
return;}
let result, types = {};
for (let product of obj) {
if ( === prop) {
result = product;}
if (types[]) {
types[](product);} else {
types[] = [product];}}
// Get a product by name
if (result) {
return result;}
// Get products by type
if (prop in types) {
return types[prop];}
// Get product types
if (prop === 'types') {
return (types);}
return undefined;}});
(products[0]); // { name: 'Firefox', type: 'browser'}
(products['Firefox']); // { name: 'Firefox', type: 'browser'}
(products['Chrome']); // undefined
(owser); // [{ name: 'Firefox', type: 'browser'}, { name: 'SeaMonkey', type: 'browser'}]
(); // ['browser', 'mailer']
(); // 3
A complete traps list exampleNow in order to create a complete sample traps list, for didactic purposes, we will try to proxify a non-native object that is particularly suited to this type of operation: the docCookies global object created by a simple cookie framework.
/*
var docCookies =... get the "docCookies" object here:
*/
var docCookies = new Proxy(docCookies, {
get: function (oTarget, sKey) {
return oTarget[sKey] || tItem(sKey) || undefined;},
set: function (oTarget, sKey, vValue) {
if (sKey in oTarget) { return false;}
return tItem(sKey, vValue);},
deleteProperty: function (oTarget, sKey) {
if (! sKey in oTarget) { return false;}
return moveItem(sKey);},
enumerate: function (oTarget, sKey) {
return ();},
ownKeys: function (oTarget, sKey) {
has: function (oTarget, sKey) {
return sKey in oTarget || oTarget. hasItem(sKey);},
defineProperty: function (oTarget, sKey, oDesc) {
if (oDesc && 'value' in oDesc) { tItem(sKey, );}
return oTarget;},
getOwnPropertyDescriptor: function (oTarget, sKey) {
var vValue = tItem(sKey);
return vValue? {
value: vValue,
writable: true,
enumerable: true,
configurable: false}: undefined;}, });
/* Cookies test */
(_cookie1 = 'First value');
(tItem('my_cookie1'));
tItem('my_cookie1', 'Changed value');
(_cookie1);
SpecificationsSpecificationECMAScript Language Specification (ECMAScript)# sec-proxy-objectsBrowser compatibilityBCD tables only load in the browserSee also
"Proxies are awesome" Brendan Eich presentation at JSConf (slides)
Tutorial on proxies
ES6 in Action: How to Use Proxies – SitePoint
In computing terms, proxies sit between you and the things you’re communicating with. The term is most often applied to a proxy server — a device between the web browser (Chrome, Firefox, Safari, Edge etc. ) and the web server (Apache, Nginx, IIS etc. ) where a page is located. The proxy server can modify requests and responses. For example, it can increase efficiency by caching regularly accessed assets and serving them to multiple users.
ES6 proxies sit between your code and an object. A proxy allows you to perform meta-programming operations such as intercepting a call to inspect or change an object’s property.
The following terminology is used in relation to ES6 proxies:
target
The original object the proxy will virtualize. This could be a JavaScript object such as the jQuery library or native objects such as arrays or even another proxies.
handler
An object which implements the proxy’s behavior using…
traps
Functions defined in the handler which provide access to the target when specific properties or methods are called.
It’s best explained with a simple example. We’ll create a target object named target which has three properties:
const target = {
a: 1,
b: 2,
c: 3};
We’ll now create a handler object which intercepts all get operations. This returns the target’s property when it’s available or 42 otherwise:
const handler = {
get: function(target, name) {
return (
name in target? target[name]: 42);}};
We now create a new Proxy by passing the target and handler objects. Our code can interact with the proxy rather than accessing the target object directly:
const proxy = new Proxy(target, handler);
(proxy. a); // 1
(proxy. b); // 2
(proxy. c); // 3
(aningOfLife); // 42
Let’s expand the proxy handler further so it only permits single-character properties from a to z to be set:
return (name in target? target[name]: 42);},
set: function(target, prop, value) {
if ( == 1 && prop >= ‘a’ && prop <= 'z') {
target[prop] = value;
return true;}
else {
throw new ReferenceError(prop + ' cannot be set');
return false;}}};
proxy. a = 10;
proxy. b = 20;
= 30;
// Exception: ReferenceError: ABC cannot be set
Proxy Trap Types
We’ve seen the get and set in action which are likely to be the most useful traps. However, there are several other trap types you can use to supplement proxy handler code:
construct(target, argList)
Traps the creation of a new object with the new operator.
get(target, property)
Traps () and must return the property’s value.
set(target, property, value)
Traps () and must set the property value. Return true if successful. In strict mode, returning false will throw a TypeError exception.
deleteProperty(target, property)
Traps a delete operation on an object’s property. Must return either true or false.
apply(target, thisArg, argList)
Traps object function calls.
has(target, property)
Traps in operators and must return either true or false.
ownKeys(target)
Traps tOwnPropertyNames() and must return an enumerable object.
getPrototypeOf(target)
Traps tPrototypeOf() and must return the prototype’s object or null.
setPrototypeOf(target, prototype)
Traps tPrototypeOf() to set the prototype object. No value is returned.
isExtensible(target)
Traps Extensible(), which determines whether an object can have new properties added. Must return either true or false.
preventExtensions(target)
Traps eventExtensions(), which prevents new properties from being added to an object. Must return either true or false.
getOwnPropertyDescriptor(target, property)
Traps tOwnPropertyDescriptor(), which returns undefined or a property descriptor object with attributes for value, writable, get, set, configurable and enumerable.
defineProperty(target, property, descriptor)
Traps fineProperty() which defines or modifies an object property. Must return true if the target property was successfully defined or false if not.
Proxy Example 1: Profiling
Proxies allow you to create generic wrappers for any object without having to change the code within the target objects themselves.
In this example, we’ll create a profiling proxy which counts the number of times a property is accessed. First, we require a makeProfiler factory function which returns the Proxy object and retains the count state:
// create a profiling Proxy
function makeProfiler(target) {
const
count = {},
handler = {
if (name in target) {
count[name] = (count[name] || 0) + 1;
return target[name];}}};
return {
proxy: new Proxy(target, handler),
count: count}};
We can now apply this proxy wrapper to any object or another proxy. For example:
const myObject = {
h: 'Hello',
w: 'World'};
// create a myObject proxy
const pObj = makeProfiler(myObject);
// access properties
(); // Hello
(); // World
(); // 2
(); // 1
While this is a trivial example, imagine the effort involved if you had to perform property access counts in several different objects without using proxies.
Proxy Example 2: Two-way Data Binding
Data binding synchronizes objects. It’s typically used in JavaScript MVC libraries to update an internal object when the DOM changes and vice versa.
Presume we have an input field with an ID of inputname:
We also have a JavaScript object named myUser with an id property which references this input:
// internal state for #inputname field
const myUser = {
id: ‘inputname’,
name: ”};
Our first objective is to update when a user changes the input value. This can be achieved with an onchange event handler on the field:
inputChange(myUser);
// bind input to object
function inputChange(myObject) {
if (! myObject ||! ) return;
const input = tElementById();
dEventListener(‘onchange’, function(e) {
=;});}
Our next objective is to update the input field when we modify within JavaScript code. This is not as simple, but proxies offer a solution:
// proxy handler
const inputHandler = {
set: function(target, prop, newValue) {
if (prop == ‘name’ &&) {
// update object property
target[prop] = newValue;
// update input field value
tElementById() = newValue;
else return false;}}
// create proxy
const myUserProxy = new Proxy(myUser, inputHandler);
// set a new name
= ‘Craig’;
(); // Craig
(tElementById(‘inputname’)); // Craig
This may not be the most efficient data-binding option, but proxies allow you to alter the behavior of many existing objects without changing their code.
Further Examples
’s article Negative Array Index in JavaScript suggests using proxies to implement negative array indexes. For example, arr[-1] returns the last element, arr[-2] returns the second-to-last element, and so on.
Nicholas C. Zakas’ article Creating type-safe properties with ECMAScript 6 proxies illustrates how proxies can be used to implement type safety by validating new values. In the example above, we could verify was always set to a string and throw an error otherwise.
Proxy Support
The power of proxies may not be immediately obvious, but they offer powerful meta-programming opportunities. Brendan Eich, the creator of JavaScript, thinks Proxies are Awesome!
Currently, proxy support is implemented in Node and all current browsers, with the exception of Internet Explorer 11. However, please note that not all browsers support all traps. You can get a better idea of what’s supported by consulting this browser compatibility table on the MDN Proxy page.
Unfortunately, it’s not possible to polyfill or transpile ES6 proxy code using tools such as Babel, because proxies are powerful and have no ES5 equivalent.
Craig is a freelance UK web consultant who built his first page for IE2. 0 in 1995. Since that time he’s been advocating standards, accessibility, and best-practice HTML5 techniques. He’s created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He’s written more than 1, 000 articles for SitePoint and you can find him @craigbuckler.
Frequently Asked Questions about es proxy
What is proxy in ES6?
ES6 proxies sit between your code and an object. A proxy allows you to perform meta-programming operations such as intercepting a call to inspect or change an object’s property.Apr 12, 2018
What is a proxy function?
Proxy servers act as a firewall and web filter, provide shared network connections, and cache data to speed up common requests. A good proxy server keeps users and the internal network protected from the bad stuff that lives out in the wild internet.May 7, 2021
What are proxy objects?
A proxy object acts as an intermediary between the client and an accessible object. The purpose of the proxy object is to monitor the life span of the accessible object and to forward calls to the accessible object only if it is not destroyed.Aug 19, 2020