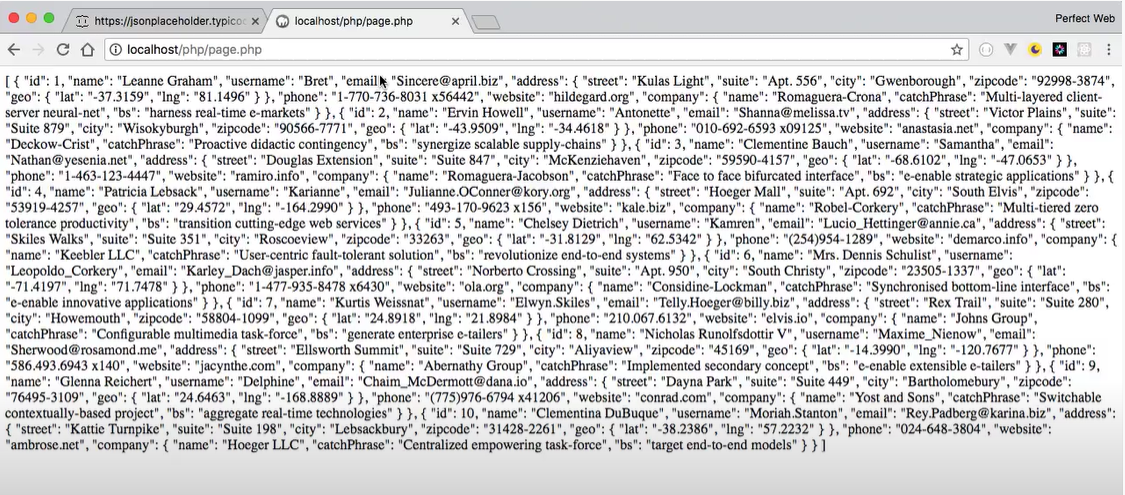
Curl Php Proxy Authentication
Proxy Authentication Required with cURL – Stack Overflow
I would like to use a cURL function, but I’m behind a proxy, so I get an HTTP/1. 1 407 Proxy Authentication Required error…
This is the php code I use:
$proxy_user = ‘Michiel’;
$proxy_pass = ‘mypassword’;
$proxy_url = ‘myproxyurl:port’;
$proxy = true;
$service_url = “;
$service_user = ‘user:password:FO’;
$service_pass = ‘password’;
$ch = curl_init($service_url);
// Set proxy if necessary
if ($proxy) {
curl_setopt($ch, CURLOPT_PROXY, $proxy_url);
curl_setopt($ch, CURLOPT_PROXYUSERPWD, $proxy_user. ‘:’. $proxy_pass);
curl_setopt($ch, CURLOPT_PROXYPORT, 8080);
curl_setopt($ch, CURLOPT_PROXYAUTH, CURLAUTH_NTLM);}
// Set service authentication
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, TRUE);
curl_setopt($ch, CURLOPT_USERPWD, “{$service_user}:{$service_pass}”);
// HTTP headers
$headers[‘Authorization’] = ‘Basic ‘. base64_encode(“$proxy_user:$proxy_pass”);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_VERBOSE, 1);
curl_setopt($ch, CURLOPT_HEADER, TRUE);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($ch, CURLOPT_ENCODING, ”);
curl_setopt($ch, CURLOPT_TIMEOUT, 15);
//WARNING: this would prevent curl from detecting a ‘man in the middle’ attack
curl_setopt ($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt ($ch, CURLOPT_SSL_VERIFYPEER, 0);
$data = curl_exec($ch);
And I don’t know what I do wrong… How can I get around this error?
asked Mar 22 ’12 at 12:23
3
This is what I’m mostly using:
function curlFile($url, $proxy_ip, $proxy_port, $loginpassw)
{
//$loginpassw = ‘username:password’;
//$proxy_ip = ‘192. 168. 1. 1’;
//$proxy_port = ‘12345’;
//$url = ”;
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_PROXYPORT, $proxy_port);
curl_setopt($ch, CURLOPT_PROXYTYPE, ‘HTTP’);
curl_setopt($ch, CURLOPT_PROXY, $proxy_ip);
curl_setopt($ch, CURLOPT_PROXYUSERPWD, $loginpassw);
curl_close($ch);
return $data;}
answered Mar 23 ’12 at 11:51
Dr. KameleonDr. Kameleon21. 7k19 gold badges108 silver badges212 bronze badges
6
Try to implement this in your function
function send_string($data) {
// pr($data);exit;
$equi_input=”Your values”;
// echo $equi_input; exit;
$agent = “”//Agent Setting for Netscape
$url = “”; // URL to POST FORM. (Action of Form)
//xml format
$efx_request=strtoupper($equi_input);
//echo $efx_request;exit;
$post_fields = “site_id=””&service_name=””&efx_request=$efx_request”;
$credentials =;
$headers = array(“HTTP/1. 1”,
“Content-Type: application/x-www-form-urlencoded”,
“Authorization: Basic “. $credentials);
$fh = fopen(‘/tmp/’, ‘w’) or die(“can’t open file”); //File to write the header information of the curl request.
$ch = curl_init(); // Initialize a CURL session.
curl_setopt($ch, CURLOPT_USERAGENT, $agent);
curl_setopt($ch, CURLOPT_URL, $url); // Pass URL as parameter.
curl_setopt($ch, CURLOPT_STDERR, $fh);
curl_setopt($ch, CURLOPT_POST, 1); // use this option to Post a form
curl_setopt($ch, CURLOPT_POSTFIELDS, $post_fields); // Pass form Fields.
curl_setopt($ch, CURLOPT_VERBOSE, ‘1’);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, ‘1’);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
$result = curl_exec($ch); // grab URL and pass it to the variable.
curl_close($ch); // close curl resource, and free system resources.
fclose($fh);
//echo ‘
';print_r($array);echo '
‘;exit;
if (! empty($result)) {
return $result;} else {
echo “Curl Error”. curl_error($ch);}}
check request send 200ok…
And check your secure certificate if you are using….
Thanks.
davehale234, 3742 gold badges25 silver badges39 bronze badges
answered Mar 22 ’12 at 12:30
Javascript CoderJavascript Coder5, 3697 gold badges39 silver badges85 bronze badges
4
This is what I use and it works perfectly:
$proxy = “1. 1:12121″;
$useragent=”Mozilla/5. 0 (Windows; U; Windows NT 5. 1; en-US; rv:1. 8. 1) Gecko/20061204 Firefox/2. 0. 1”;
$url = “;
$credentials = “user:pass”
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 15);
curl_setopt($ch, CURLOPT_HTTP_VERSION, ‘CURL_HTTP_VERSION_1_1’);
curl_setopt($ch, CURLOPT_HTTPPROXYTUNNEL, 1);
curl_setopt($ch, CURLOPT_PROXY, $proxy);
curl_setopt($ch, CURLOPT_PROXYUSERPWD, $credentials);
curl_setopt($ch, CURLOPT_USERAGENT, $useragent);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
$result=curl_exec ($ch);
curl_close ($ch);
answered Mar 25 ’15 at 19:42
Pedro LobitoPedro Lobito80. 1k27 gold badges219 silver badges238 bronze badges
Assuming the $proxy_user:$proxy_pass are the correct values, if you are behind an office firewall the authentication credentials to be used would be that of a network administrator and not your own… I’ve encountered something very similar in my work environment and that was the case for me as my normal credentials didn’t have the necessary privileges. The best way to ensure that this is the case however would be to ensure the code works in an open network with your proxy settings commented. Hope this helps!
answered Mar 23 ’12 at 11:46
optimusprime619optimusprime6197462 gold badges16 silver badges37 bronze badges
Not the answer you’re looking for? Browse other questions tagged php curl proxy or ask your own question.
PHP: Using cURL with a proxy. – This Interests Me
This is a guide on how to use a proxy with PHP’s cURL functions. In this tutorial, we will send our HTTP request via a specific proxy IP and port.
Why use a proxy?
There are various reasons why you might want to use a proxy with cURL:
To get around regional filters and country blocks.
Using a proxy IP allows you to mask your own IP address.
To debug network connection issues.
Using a proxy with PHP’s cURL functions.
Take a look at the following PHP code, which you can use to authenticate with a proxy via cURL and send a HTTP GET request.
//The URL you want to send a cURL proxy request to.
$url = ”;
//The IP address of the proxy you want to send
//your request through.
$proxyIP = ‘1. 2. 3. 4’;
//The port that the proxy is listening on.
$proxyPort = ‘1129’;
//The username for authenticating with the proxy.
$proxyUsername = ‘myusername’;
//The password for authenticating with the proxy.
$proxyPassword = ‘mypassword’;
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($ch, CURLOPT_HTTPPROXYTUNNEL, 1);
//Set the proxy IP.
curl_setopt($ch, CURLOPT_PROXY, $proxyIP);
//Set the port.
curl_setopt($ch, CURLOPT_PROXYPORT, $proxyPort);
//Specify the username and password.
curl_setopt($ch, CURLOPT_PROXYUSERPWD, “$proxyUsername:$proxyPassword”);
//Execute the request.
$output = curl_exec($ch);
//Check for errors.
if(curl_errno($ch)){
throw new Exception(curl_error($ch));}
//Print the output.
echo $output;
In the code snippet above, we connected to a proxy that requires authentication before sending a simple GET request.
If the proxy in question does not require authentication, then you can omit the CURLOPT_PROXYUSERPWD line from your code.
“Failed to connect to 1. 4 port 1129: Timed out”
This means that cURL could not connect to the proxy on that IP and port. Make sure that both the IP and port are correct and that the proxy is operating correctly.
“Failed to connect to 1. 4 port 1129: Connection refused”
This error usually occurs when you have specified an incorrect port number. i. e. The IP address of the proxy was correct, but it is not listening for requests on that port. There is also the possibility that the server is up, but the software that runs the proxy is not running.
“Received HTTP code 407 from proxy after CONNECT”
The username and password combination that you are using with CURLOPT_PROXYUSERPWD is incorrect. Make sure that you are separating the username and password by a colon: character.
Using proxy servers with cURL in PHP | Beamtic
Tutorial on how to use proxy servers with cURL and PHP
34141 views
By. Jacob
Edited: 2021-04-12 08:45
Setting a proxy server to be used with cURL and PHP is relatively simple, it mostly depends on the server that you are using, and authentication method (if any). The HTTP authentication method is controlled with the CURLOPT_PROXYAUTH option, the default method is CURLAUTH_BASIC – if the proxy requires authentication, a username and password can be set in the [username]:[password] format, using the CURLOPT_PROXYUSERPWD option.
For now we’ll just focus on using a proxy that doesn’t require any authentication. Setting a proxy server and a port number in PHP for cURL can be done using the CURLOPT_PROXY option, like shown in the below example:
curl_setopt($ch, CURLOPT_PROXY, ‘128. 0. 3:8080’);
As shown in the above example, you can set the a proxy with the IP:PORT syntax in PHP using cURL. But if you prefer to keep the ip seperated from the port, you can also use the CURLOPT_PROXYPORT option, which would result in the below PHP code:
curl_setopt($ch, CURLOPT_PROXY, ‘128. 3’);
curl_setopt($ch, CURLOPT_PROXYPORT, ‘8080’);
After setting a proxy server, you will be able to perform the request using the curl_exec function. I. e.
$ch = curl_init($url);
$url = “;
// Perform the request, and save content to $result
$result = curl_exec($ch);
echo $result;
Setting cURL Proxy Type
cURL supports two proxy types, the default is HTTP, and the other option is SOCKS5. You can set the proxy type using the CURLOPT_PROXYTYPE option. e.
curl_setopt($ch, CURLOPT_PROXYTYPE, CURLPROXY_SOCKS5);
You really only need to set the type of the proxy, if you are not using a HTTP proxy.
Setting Authentication Method
As mentioned in the beginning of the tutorial, setting the authentication method of a proxy server can be done using the CURLOPT_HTTPAUTH option. To make this work properly, we will also need to provide a username and password for the proxy server, this is all accomplished in the below script, in which we are just using a BASIC authentication method.
curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC);
// The username and password
curl_setopt($ch, CURLOPT_PROXYUSERPWD, ‘USERNAME:PASSWORD’);
Other authentication methods include the following:
CURLAUTH_BASIC
CURLAUTH_DIGEST
CURLAUTH_GSSNEGOTIATE
CURLAUTH_NTLM
CURLAUTH_ANY
CURLAUTH_ANYSAFE
vertical bar | (or) operator can be used to combine methods. If this is done, cURL will poll the server to see what methods it supports and pick the best.
Tools:
Your User Agent
Your Request Headers
You can use the following API endpoints for testing purposes:
How to use the AVIF image format in PHP; A1 or AVIF is a new image format that offers better compression than WebP, JPEG and PNG, and that already works in Google Chrome. How to create a router in PHP to handle different request types, paths, and request parameters. How much faster is C++ than PHP to increment and display a counter in a loop? Detecting the request method used to fetch a web page with PHP, routing, HTTP responses, and more. How to create a custom error handler for PHP that handles non-fetal in: PHP Tutorials