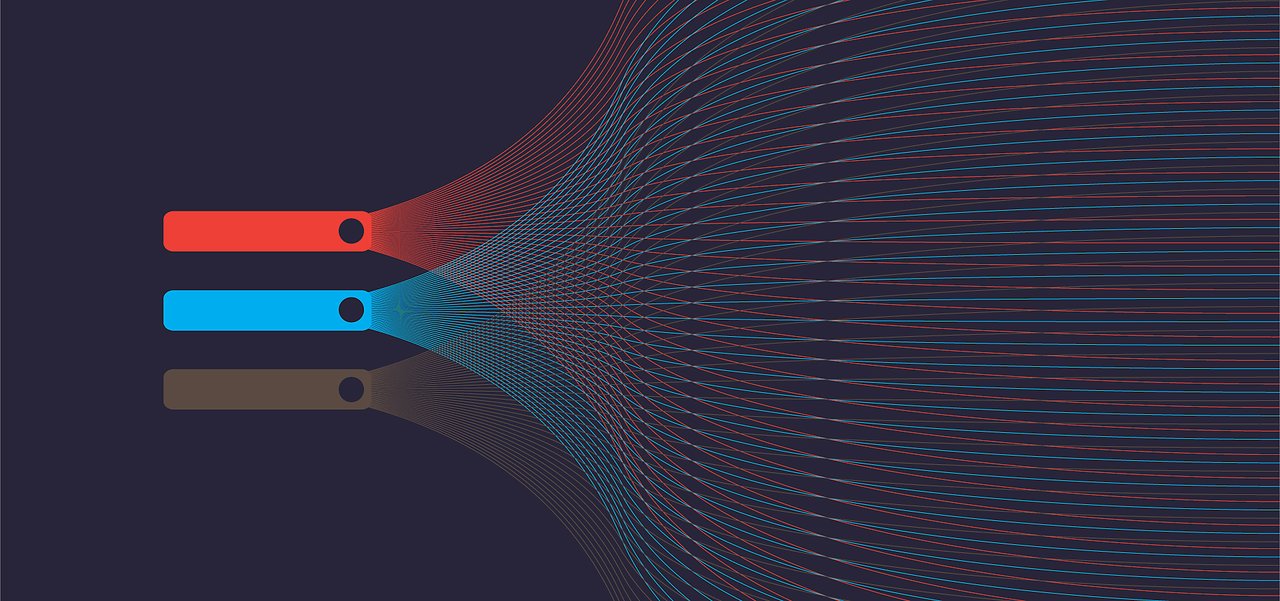
Chrome Headless Browser
Getting Started with Headless Chrome | Web – Google …
Engineer @ Google working on web tooling: Headless Chrome, Puppeteer, Lighthouse
TL;DR
Headless Chrome
is shipping in Chrome 59. It’s a way to run the Chrome browser in a headless environment.
Essentially, running
Chrome without chrome! It brings all modern web platform features provided
by Chromium and the Blink rendering engine to the command line.
Why is that useful?
A headless browser is a great tool for automated testing and server environments where you
don’t need a visible UI shell. For example, you may want to run some tests against
a real web page, create a PDF of it, or just inspect how the browser renders an URL.
Starting Headless (CLI)
The easiest way to get started with headless mode is to open the Chrome binary
from the command line. If you’ve got Chrome 59+ installed, start Chrome with the –headless flag:
chrome \
–headless \ # Runs Chrome in headless mode.
–disable-gpu \ # Temporarily needed if running on Windows.
–remote-debugging-port=9222 \
# URL to open. Defaults to about:blank.
chrome should point to your installation of Chrome. The exact location will
vary from platform to platform. Since I’m on Mac, I created convenient aliases
for each version of Chrome that I have installed.
If you’re on the stable channel of Chrome and cannot get the Beta, I recommend
using chrome-canary:
alias chrome=”/Applications/Google\ \ Chrome”
alias chrome-canary=”/Applications/Google\ Chrome\ \ Chrome\ Canary”
alias chromium=”/Applications/”
Download Chrome Canary here.
Command line features
In some cases, you may not need to programmatically script Headless Chrome.
There are some useful command line flags
to perform common tasks.
Printing the DOM
The –dump-dom flag prints to stdout:
chrome –headless –disable-gpu –dump-dom
Create a PDF
The –print-to-pdf flag creates a PDF of the page:
chrome –headless –disable-gpu –print-to-pdf
Taking screenshots
To capture a screenshot of a page, use the –screenshot flag:
chrome –headless –disable-gpu –screenshot
# Size of a standard letterhead.
chrome –headless –disable-gpu –screenshot –window-size=1280, 1696
# Nexus 5x
chrome –headless –disable-gpu –screenshot –window-size=412, 732
Running with –screenshot will produce a file named in the
current working directory. If you’re looking for full page screenshots, things
are a tad more involved. There’s a great blog
post from David Schnurr that has you covered. Check out
Using headless Chrome as an automated screenshot tool.
REPL mode (read-eval-print loop)
The –repl flag runs Headless in a mode where you can evaluate JS expressions
in the browser, right from the command line:
$ chrome –headless –disable-gpu –repl –crash-dumps-dir=. /tmp [0608/(278)] Type a Javascript expression to evaluate or “quit” to exit.
>>>
{“result”:{“type”:”string”, “value”:”}}
>>> quit
$
Debugging Chrome without a browser UI?
When you run Chrome with –remote-debugging-port=9222, it starts an instance
with the DevTools protocol enabled. The
protocol is used to communicate with Chrome and drive the headless
browser instance. It’s also what tools like Sublime, VS Code, and Node use for
remote debugging an application. #synergy
Since you don’t have browser UI to see the page, navigate to localhost:9222
in another browser to check that everything is working. You’ll see a list of
inspectable pages where you can click through and see what Headless is rendering:
DevTools remote debugging UI
From here, you can use the familiar DevTools features to inspect, debug, and tweak
the page as you normally would. If you’re using Headless programmatically, this
page is also a powerful debugging tool for seeing all the raw DevTools protocol
commands going across the wire, communicating with the browser.
Using programmatically (Node)
Puppeteer
Puppeteer is a Node library
developed by the Chrome team. It provides a high-level API to control headless
(or full) Chrome. It’s similar to other automated testing libraries like Phantom
and NightmareJS, but it only works with the latest versions of Chrome.
Among other things, Puppeteer can be used to easily take screenshots, create PDFs,
navigate pages, and fetch information about those pages. I recommend the library
if you want to quickly automate browser testing. It hides away the complexities
of the DevTools protocol and takes care of redundant tasks like launching a
debug instance of Chrome.
Install it:
npm i –save puppeteer
Example – print the user agent
const puppeteer = require(‘puppeteer’);
(async() => {
const browser = await ();
(await rsion());
await ();})();
Example – taking a screenshot of the page
const page = await wPage();
await (”, {waitUntil: ‘networkidle2’});
await ({path: ”, format: ‘A4’});
Check out Puppeteer’s documentation
to learn more about the full API.
The CRI library
chrome-remote-interface
is a lower-level library than Puppeteer’s API. I recommend it if you want to be
close to the metal and use the DevTools protocol directly.
Launching Chrome
chrome-remote-interface doesn’t launch Chrome for you, so you’ll have to take
care of that yourself.
In the CLI section, we started Chrome manually using
–headless –remote-debugging-port=9222. However, to fully automate tests, you’ll probably
want to spawn Chrome from your application.
One way is to use child_process:
const execFile = require(‘child_process’). execFile;
function launchHeadlessChrome(url, callback) {
// Assuming MacOSx.
const CHROME = ‘/Applications/Google\ \ Chrome’;
execFile(CHROME, [‘–headless’, ‘–disable-gpu’, ‘–remote-debugging-port=9222’, url], callback);}
launchHeadlessChrome(”, (err, stdout, stderr) => {… });
But things get tricky if you want a portable solution that works across multiple
platforms. Just look at that hard-coded path to Chrome:(
Using ChromeLauncher
Lighthouse is a marvelous
tool for testing the quality of your web apps. A robust module for launching
Chrome was developed within Lighthouse and is now extracted for standalone use.
The chrome-launcher NPM module
will find where
Chrome is installed, set up a debug instance, launch the browser, and kill it
when your program is done. Best part is that it works cross-platform thanks to
Node!
By default, chrome-launcher will try to launch Chrome Canary (if it’s
installed), but you can change that to manually select which Chrome to use. To
use it, first install from npm:
npm i –save chrome-launcher
Example – using chrome-launcher to launch Headless
const chromeLauncher = require(‘chrome-launcher’);
// Optional: set logging level of launcher to see its output.
// Install it using: npm i –save lighthouse-logger
// const log = require(‘lighthouse-logger’);
// tLevel(‘info’);
/**
* Launches a debugging instance of Chrome.
* @param {boolean=} headless True (default) launches Chrome in headless mode.
* False launches a full version of Chrome.
* @return {Promise
*/
function launchChrome(headless=true) {
return ({
// port: 9222, // Uncomment to force a specific port of your choice.
chromeFlags: [
‘–window-size=412, 732’,
‘–disable-gpu’,
headless? ‘–headless’: ”]});}
launchChrome()(chrome => {
(`Chrome debuggable on port: ${}`);…
// ();});
Running this script doesn’t do much, but you should see an instance of
Chrome fire up in the task manager that loaded about:blank. Remember, there
won’t be any browser UI. We’re headless.
To control the browser, we need the DevTools protocol!
Retrieving information about the page
Let’s install the library:
npm i –save chrome-remote-interface
Examples
const CDP = require(‘chrome-remote-interface’);…
launchChrome()(async chrome => {
const version = await rsion({port:});
(version[‘User-Agent’]);});
Results in something like: HeadlessChrome/60. 0. 3082. 0
Example – check if the site has a web app manifest
(async function() {
const chrome = await launchChrome();
const protocol = await CDP({port:});
// Extract the DevTools protocol domains we need and enable them.
// See API docs: const {Page} = protocol;
await ();
vigate({url: ”});
// Wait for before doing stuff.
Page. loadEventFired(async () => {
const manifest = await tAppManifest();
if () {
(‘Manifest: ‘ +);
();} else {
(‘Site has no app manifest’);}
();
(); // Kill Chrome. });})();
Example – extract the
// See API docs: const {Page, Runtime} = protocol;
await ([(), ()]);
const js = “document. querySelector(‘title’). textContent”;
// Evaluate the JS expression in the page.
const result = await Runtime. evaluate({expression: js});
(‘Title of page: ‘ +);
Using Selenium, WebDriver, and ChromeDriver
Right now, Selenium opens a full instance of Chrome. In other words, it’s an
automated solution but not completely headless. However, Selenium can be
configured to run headless Chrome with a little work. I recommend
Running Selenium with Headless Chrome
if you want the
full instructions on how to set things up yourself, but I’ve dropped in some
examples below to get you started.
Using ChromeDriver
ChromeDriver 2. 32
uses Chrome 61 and works well with headless Chrome.
Install:
npm i –save-dev selenium-webdriver chromedriver
Example:
const fs = require(‘fs’);
const webdriver = require(‘selenium-webdriver’);
const chromedriver = require(‘chromedriver’);
const chromeCapabilities = ();
(‘chromeOptions’, {args: [‘–headless’]});
const driver = new er(). forBrowser(‘chrome’). withCapabilities(chromeCapabilities)
// Navigate to, enter a search.
(”);
ndElement({name: ‘q’}). sendKeys(‘webdriver’);
ndElement({name: ‘btnG’})();
((‘webdriver – Google Search’), 1000);
// Take screenshot of results page. Save to disk.
driver. takeScreenshot()(base64png => {
fs. writeFileSync(”, new Buffer(base64png, ‘base64’));});
Using WebDriverIO
WebDriverIO is a higher level API on top of Selenium WebDriver.
npm i –save-dev webdriverio chromedriver
Example: filter CSS features on
const webdriverio = require(‘webdriverio’);
const PORT = 9515;
([
‘–url-base=wd/hub’,
`–port=${PORT}`,
‘–verbose’]);
(async () => {
const opts = {
port: PORT,
desiredCapabilities: {
browserName: ‘chrome’,
chromeOptions: {args: [‘–headless’]}}};
const browser = (opts)();
await (”);
const title = await tTitle();
(`Title: ${title}`);
await browser. waitForText(”, 3000);
let numFeatures = await tText(”);
(`Chrome has ${numFeatures} total features`);
await tValue(‘input[type=”search”]’, ‘CSS’);
(‘Filtering features… ‘);
await (1000);
numFeatures = await tText(”);
(`Chrome has ${numFeatures} CSS features`);
const buffer = await veScreenshot(”);
(‘Saved screenshot… ‘);
();})();
Further resources
Here are some useful resources to get you started:
Docs
DevTools Protocol Viewer – API reference docs
Tools
chrome-remote-interface – node
module that wraps the DevTools protocol
Lighthouse – automated tool for testing
web app quality; makes heavy use of the protocol
chrome-launcher –
node module for launching Chrome, ready for automation
Demos
“The Headless Web” – Paul Kinlan’s great blog
post on using Headless with
FAQ
Do I need the –disable-gpu flag?
Only on Windows. Other platforms no longer require it. The –disable-gpu flag is a
temporary work around for a few bugs. You won’t need this flag in future versions of
Chrome. See
for more information.
So I still need Xvfb?
No. Headless Chrome doesn’t use a window so a display server like Xvfb is
no longer needed. You can happily run your automated tests without it.
What is Xvfb? Xvfb is an in-memory display server for Unix-like systems that enables you
to run graphical applications (like Chrome) without an attached physical display.
Many people use Xvfb to run earlier versions of Chrome to do “headless” testing.
How do I create a Docker container that runs Headless Chrome?
Check out lighthouse-ci. It has an
example Dockerfile
that uses node:8-slim as a base image, installs +
runs Lighthouse
on App Engine Flex.
Can I use this with Selenium / WebDriver / ChromeDriver?
Yes. See Using Selenium, WebDrive, or ChromeDriver.
How is this related to PhantomJS?
Headless Chrome is similar to tools like PhantomJS. Both
can be used for automated testing in a headless environment. The main difference
between the two is that Phantom uses an older version of WebKit as its rendering
engine while Headless Chrome uses the latest version of Blink.
At the moment, Phantom also provides a higher level API than the DevTools protocol.
Where do I report bugs?
For bugs against Headless Chrome, file them on
For bugs in the DevTools protocol, file them at
Headless Chrome: DevOps Love It, So Do Hackers, Here’s Why
Google Chrome is the most popular web browser and has been so for almost a decade. Each new version of Chrome brings new usability, security and performance features.
This article focuses on the “headless mode” feature that Google released more than a year ago; and, since day one has become very popular not only among software engineers and testers but also with attackers.
Off with their heads!
Headless mode is a functionality that allows the execution of a full version of the latest Chrome browser while controlling it programmatically. It can be used on servers without dedicated graphics or display, meaning that it runs without its “head”, the Graphical User Interface (GUI).
In headless mode, it’s possible to run large scale web application tests, navigate from page to page without human intervention, confirm JavaScript functionality and generate reports.
As with benign cases, the same functionality takes place in malicious scenarios, when an attacker needs to evaluate JavaScript or emulate browser functionality.
The practice of web browser automation isn’t new. It’s used in dedicated headless browsers like PhantomJS and NightmareJS, test frameworks like Capybara and Jasmin, and tools like Selenium that can automate different browsers including Chrome.
How popular is Headless Chrome?
The chart below shows the amount of traffic generated by Headless Chrome and other major headless browsers since its release date in June 2017. In comparison to other headless browsers and automation frameworks, Headless Chrome overtook the previous leader, PhantomJS, within a year of its release.
Automated browser trends over the last year
The data collected from our cloud WAF statistics, reinforced by data from Google Trends, highlight how the popularity of PhantomJS fades, while Headless Chrome’s trajectory keeps climbing.
PhantomJS and Headless Chrome: Google search trends
Automated browsers driving increased traffic
Apart from Headless Chrome’s popularity, and the degradation in the popularity of outdated tools, we observed an increase in total traffic generated by automated browsers compared to non-automated web surfing.
The chart below represents the percentage of automated browsers out of total traffic generated by web browsers:
Traffic ratio between automated and non-automated browsers
So, why is Headless Chrome so popular?
There are several reasons for Headless Chrome’s popularity; one being the support for Chrome’s new “out of the box” features, which constantly introduce new trends in web development. Another reason is the support for major desktop, server, and mobile operating systems. Headless Chrome also has convenient development tools and many additional useful features for Devs.
The release of Puppeteer a couple of months after the release of the headless functionality was a decisive push in Headless Chrome’s popularity. Puppeteer is a NodeJs library developed by the Chrome team, which provides a high-level API to control headless and full versions of the latest Chrome.
Enter Puppeteer
Puppeteer is a common and natural way to control Chrome. It provides full access to browser features and, most importantly, can run Chrome in fully headless mode on a remote server, which is very useful for both automation teams and attackers.
Without much difficulty, attackers can put in place an infrastructure with a host of nodes running Headless Chrome and orchestrated by one component (Puppeteer).
Apart from Puppeteer, Chrome can be automated using webdriver and automation frameworks like Selenium or by direct access through Command Line Interface (CLI). In this case, some Chrome functionality will be limited, but it offers the flexibility to write automation in any programing language besides NodeJS and JavaScript.
Just how popular is it among attackers?
By analyzing malicious activity generated by automated browsers, I found that PhantomJS was a leader not only in the amount of traffic it produced but also in malicious activity.
However, nowadays, Chrome occupies the top of the “attackers’ podium, ” with half of the malicious traffic divided evenly between execution in headless and non-headless mode.
Taking a closer look at malicious traffic, however, I found that there are no specific trends indicating a preference among attackers for Headless Chrome to exploit vulnerabilities, inject SQL or carry out cross-site scripting attacks (XSS). That said, occasional spikes show attempts at targeting specific sites by using vulnerability scanners, or attempts to exploit newly released vulnerabilities using the “spray and pray” technique.
Using a web browser for vulnerability scanning is crafty, but not a new approach, as it can help to bypass some validation mechanisms based on validation of the legitimacy of the client.
WAF events generated by Headless Chrome
Analyzing traffic from the last year, I didn’t find any DDoS attacks performed from a botnet based on Headless Chrome. Nothing similar to the Headless Android Botnet that was discovered two years ago and since then all but vanished.
Usage of automated browsers in general, and Headless Chrome in particular, for DDoS, is not common practice. The reason for this is the low request rate to the server that browsers can generate. As Chrome receives the response from the server, evaluates it and only then performs the next request, its rate is very low in comparison to a simple script that floods with many requests and doesn’t “care” about the responses.
Having said that, we observe more than 10K unique IP addresses daily performing scraping, sniping, carding, blackhat SEO and other types of malicious activity where JavaScript evaluation is necessary to perform the attack. Distribution among the countries performing these malicious activities is presented in the chart below. While 7% of the traffic is coming from proxies or VPNs to hide the origin of the attack.
Geographical distribution of malicious Headless Chrome traffic
But what about legitimate services?
Headless Chrome isn’t only used by attackers but also by legitimate services. We observe dozens of legitimate well-known web tools that use it to access websites.
Search engines use it to render the page, generate dynamic content and index data from single page web applications.
SEO tools use it to analyze your website and help promote it better.
Monitoring tools use Headless Chrome to measure performance and JavaScript execution time of web applications.
Online testing tools render pages and compare it to previous versions to track regression or distortion in the user interface.
Ok, so how do we make sure we’re protected?
At this point, you’re probably asking yourself whether or not to block Headless Chrome or any other automated browsers.
The answer to this question is “yes… and no. ”
Using Headless Chrome by itself is not malicious, and as stated earlier, there are legitimate scenarios and services that use this functionality to access websites. Whitelisting all legitimate services is tough work, as it requires constant mapping and maintaining the lists of such services and their IPs.
The decision to block Headless Chrome requests or not should be based on the intent and behavior of each IP and session individually.
Unless the payload is malicious (which is high evidence of malicious activity), it is better to pass some requests to the website, analyze the behavior and only then decide whether to block or not.
The reputation of IPs and their correlation, sophisticated heuristics, and machine learning algorithms can be implemented to make a deliberate decision, which will give better long-term results than aggressive blocking, at least in most cases.
For Imperva Incapsula (now Imperva Cloud Application Security) users, a set of IncapRules can be implemented to block Headless Chrome from accessing your website. Starting from a simple rule based on client classification up to sophisticated rules including rates, tags, and reputation.
Try Imperva for Free
Protect your business for 30 days on Imperva.
Start Now
What is Google Chrome Headless Mode – DebugBar
All you Need to Know about Headless ChromeThe Headless mode is a feature which allows the execution of a full version of the Chrome Browser. It provides the ability to control Chrome via external programs. The headless mode can run on servers without the need for dedicated display or graphics. It gets the name headless because it runs without the Graphical User Interface (GUI). The GUI of a program is referred to as the headless mode of the Google Chrome browser provides the ability to implement and run massive-scale web app tests, navigate from one page to another without human interference, validate JavaScript functions, and provide detailed reporting. In short, it is a step towards article will discuss the headless mode that Google added to Chrome in 2017 and has subsequently become extremely popular among software engineers, testers, and even attackers and how it bypassed Selenium and other popular Headless Chrome is not the first entry into the automation paradigm. Automation has long been used in other headless browsers, including the PhantomJS and Nightmare antomJS is a discontinued headless browser used for automating web page interaction whereas the Nightmare JS is a browser automation library for frameworks are also automated, such as the Capybara and Jasmin. Both of these softwares simulate scenarios for user stories and automate the web application testing based upon behavior-driven lenium is a top-rated app that automates many browsers, including Google Chrome web browser. Selenium provides the option for a browser add on that can easily replicate the user interactions and popularity of Headless ChromeGoogle Headless Chrome has been extremely popular since its launch in 2017. Within a year, Google Chrome Browser took over the previous automation leader PhantomJS. Cloud WAF statistics following the Google trends clearly show the popularity of Chrome Headless browser increasing while the PhantomJS loses its earlier following chart confirms this Automated Browsers have increased the online traffic? An automated web browser generates much more Traffic compared to non-automated web surfing. This is because Google Headless Chrome is continuously increasing in popularity and taking over the manual paradigm while the PhantomJS, Selenium, etc. are slowly becoming diagram below verifies our claim of the increased popularity of automated makes Google Headless Chrome Browser Popular? Several factors contribute to the success of Google Headless Chrome Browser. The biggest one is Chrome’s support for innovative features that continuously provide out-of-the-box innovation and web development trends. Headless Chrome offers user-friendly tools for web development and additional helpful features for the developers. Another feature is the availability of this Headless Chrome on all operating systems including Windows, Mac, and released Puppeteer a few months after the Chrome headless functionality was unveiled. This tactic boosted the popularity of Headless Chrome amongst developers and software engineers since they now had more options. The popularity of Selenium took a great hit once it was is the Puppeteer API? Puppeteer is a node library feature which was launched a few months after the headless feature was introduced. The Puppeteer node library is user-friendly and provides flexibility to manipulate many Chrome functions. You get full browser functionality with Puppeteer in addition to running Chrome in headless mode on a server. Team managing the automated paradigm greatly benefits from the remote server Puppeteer Node API will not provide you with all the standard functions of Google Chrome browser. However, you will get the flexibility to write the automation code in any programming language you are comfortable with, excluding NodeJS and latest version of the Puppeteer Node API can fortify the functionality of Headless more about Puppeteer API from the GitHub URL. You can find a step by step process and learn how to use it and set it code along with the set up is available on GitHub. Puppeteer API is not the only solution to automate Chrome. Other options are also available. Webdriver or third-party frameworks such as Selenium or direct Command Line Interface (CLI) can also be used for this purpose. How to Setup the Chrome Headless BrowserTo use Headless Chrome, you will need to open the binary Chrome from the command line. For an average user, the commands and the interface might be a little overwhelming, but we will provide a detailed step-by-step by opening the command line on your Operating System. It is already present in operating systems. No need to install an external driver or import any library. It also comes integrated with Users: Applications -> Utilities -> TerminalWINDOWS USERS: Open Start and enter cmd in the search bar. Click the icon to open the Command-Line. Once the command line is open, we will open Chrome using it. You need to know where Chrome is stored on your machine. The location should not be confused for an icon. Depending upon your system copy and paste the following driver commands:MAC USERS: /Applications/Google ChromeWINDOWS USERS: C:Program FilesGoogleChromeApplicationYou will require a separate version of Chrome that runs as an independent application. You will need Google Chrome Canary. Users can download the latest version of the driver from this URL. Once Google Chrome Canary is downloaded, we will open it in the command line. The set up is quite simple and takes a few minutes. Mac users can copy and paste the following driver command:MAC USERS: /Applications/Google Chrome Chrome CanaryCanary will open up in a new window. Windows users will have to amend their file path to reach Canary place inside the C make Google Chrome Browser Headless, we will use the same command as we used before, but we will add this time -headless with it. So the driver command becomes:/Applications/Google Chrome Chrome Canary –headlessThe Windows Users will do the same thing with the dows USERS: tProperty(“”, “/path/to/driver”);ChromeOptions options = new ChromeOptions();dArguments(“–headless”);WebDriver driver = new ChromeDriver (options);The Yellow Chrome Canary symbol will appear in the document for a split second and then disappear. It ensures that Chrome Canary runs in Headless tivities Performed Using the Headless Chrome BrowserThe advantages offered by the Headless Chrome software are tremendous. Let us list down a few options that you can perform using Google headless Chrome. Some of these cool features are unique to this software and were not available on previous tools such as Selenium, etc. Dynamic RenderingThe search engines utilize Headless Chrome to render the page, display runtime contents, and index the material for single-page web RankingThe search engine optimization tools offered by Headless Chrome provide a robust analysis for your web application and identify the weak areas. You can improve the shortcomings to set up higher ntime CalculationHeadless Chrome provides tools that help evaluate the performance of JavaScript runtime execution. It can be useful to optimize the code to ensure faster speeds. It is faster than other available proved TestingSeveral online tools help us test the page rendering and compare it with the antecedent version to look for any changes or difficulties that might affect the user experience. An automated test can ensure the correct functioning of the latest list does not just end here. The capabilities are Options to Play Around With Headless Chrome BrowserSince we have learned how to launch and manipulate headless chrome using the command line and Canary, let us dig deeper into the capabilities with this software. All these features can be replicated on a Linux as unching a Web PageLearning how to launch the browser in headless mode will not be of much value. You will be required to open a web page to utilise all the features. Visiting a web page is relatively easy. Add the desired URL at the end of the headless flag headers in the command line interface. Following is an example for Mac users. Use the following driver command:MAC USERS: /Applications/Google Chrome Chrome Canary –headless You can add any URL at the discussed before. The Canary icon will show up and instantly disappear in the Doc. You will not see more than this. To delve deeper into the specifics, take a screenshot of the page in the command a ScreenshotYou can take a screenshot with the help of headers. Use the following example driver command:/Applications/Google Chrome Chrome Canary –headless –disable-GPU –screenshot You can add any URL at the will get a notification on the command line with the destination info. By default, it will be named Mac Users, it will be stored in the Home folder but will overwrite the previous file. Make sure to save will only get the image of one page. To capture the complete web page, you will need a PDF of the web the print to pdf header at the end of the command line. Use the following example driver command:/Applications/Google Chrome Chrome Canary –headless –disable-gpu –print-to-pdf result will be saved as an file in the home folder of Mac. Make sure to rename this file. Otherwise, it will be overwritten every time you make a new nipulating DevTools from the Command LineHeadless Chrome can be used to create a remote instance of the Chrome DevTools. This feature is convenient when it comes to controlling the Headless sure to find out your port number before trying this the previous commands, we will achieve this feat if we add headers at the end. –remote-debugging-port=8080Any port can be set, but it is a good practice to add the default one if you lack the technical experience. Use the following example driver command:/Applications/Google Chrome Chrome Canary –headless –remote-debugging-port=8080 Note: You can add any URL at the and reopen Canary when you use this will get a notification from the chrome devtools when they are the URL that you get and paste it in a new tab on the traditional Google sure to keep the port numbers wnside to Headless ChromeLooking at the previous data, it is easy to decipher that the earlier leader of automated browsing, i. e PhantomJS was a top-notch traffic magnet. However, it was a leader in malicious activity as since Chrome browser has taken over the automated paradigm, it also occupies the first spot in the attacker’s podium. The vitriolic Traffic is pretty evenly divided between the execution in headless as well as the non-headless ever, the trends reveal that there is no such preference amongst the hackers to choose Headless Chrome for carrying out a malevolent activity such as exploiting loopholes, database corruption using SQL injection, or a cross-site scripting attack (XSS) using a browsers are popularly used for this purpose because they can easily bypass the web validation techniques. This technique requires skill but is not new. Even Selenium based tools can accomplish this is a Headless Browser not used for DDoS Attacks? If we analyze the activity trends from 2017, we can see no DDoS attack performed using a Headless Chrome or the Headless Android automated browsers such as Headless Chrome for undertaking a DDoS attack is not one of the widespread options. It is because the browsers generate a low request rate to the server. Upon receiving the server’s response, Chrome first evaluates the contents and then moves on to the next request. The sequential checking of packets makes the entire process useless. Instead, hackers resort to a single piece of the script having the potential to flood the server with more requests than it can handle. There is no wait for the response, potentially choking the server. A disaster awaits the website once the server goes does not rule out that headless Chrome is not utilized to carry out other sorts of malicious activity. More than ten thousand IP addresses perform scraping, sniping, blackhat Search Engine Optimization, etc., and different kinds of activity using JavaScript evaluation on the headless Chrome. Many attackers use a Virtual Private Network or utilize other encapsulating options to conceal their identity. It can prove to be more fatal than Selenium if misused. Difference Between Chrome and ChromiumChromeChromiumFeaturesBetter update mechanism, native support for many technologies, rights to play copyrighted content Lacks all of these featuresStabilityExtremely stableCan crash quite often even in comparison with primitive versions of ChromePrivacyCollects detailed information including usage patterns, statistics, crash reports, etc. and sends it to for privacy. Can not collect and send curitySecurity is similar to Chromium since it is powered by it. Chrome automatically updates and applies all the security security as Chrome but lack of automated update cense SupportAll media formats are supported free and basic codecs are command starts the google chrome web browser in headless mode? As we have already seen, you just have to add the flag –headless when you launch the browser to be in headless CLI (Command Line Interface), just write:chrome \ – headless \ # Runs Chrome in headless mode. – disable-gpu \ # Temporarily needed if running on Windows. – remote-debugging-port=9222 \ # URL to open. Defaults to you want to print the DOM (Document Object Model), just write the flag –dump-dom. It will write the to stdout:chrome – headless – disable-gpu – dump-dom information here: Headless Chrome browser in regular usage is not malicious. It is much more powerful than previous tools including Selenium. We learnt how the combination of Puppeteer Node API and Headless Chrome can prove to be a game changer along with the GitHub URL for a detailed overview. We have discussed many scenarios when this feature can add actual value to your workflows. Initially, it might become a little challenging to play around with this software, but once you get the hang of it, the options are endless. We also discussed various commands and their driver to understand how to manipulate options. The Chrome Headless Browser is surely a better option for an average user compared to Selenium with the ability to run on Linux
Frequently Asked Questions about chrome headless browser
What is a headless Chrome browser?
Headless mode is a functionality that allows the execution of a full version of the latest Chrome browser while controlling it programmatically. It can be used on servers without dedicated graphics or display, meaning that it runs without its “head”, the Graphical User Interface (GUI).Nov 28, 2018
How do I open Chrome in headless mode?
Which command starts the google chrome web browser in headless mode? As we have already seen, you just have to add the flag –headless when you launch the browser to be in headless mode. – headless \ # Runs Chrome in headless mode. – disable-gpu \ # Temporarily needed if running on Windows.Feb 5, 2021
How do I install headless browser?
A step-by-step guide to install Headless Chromium on Ubuntu and CentOS.What is Headless Chrome? … Step 1: Update Ubuntu. … Step 2: Install Dependencies. … Step 3: Download Chrome. … Step 4: Install Chrome. … Step 5: Check Chrome Version. … Optional: Run Chrome Headless. … Step 1: Update CentOS.More items…•Oct 4, 2021