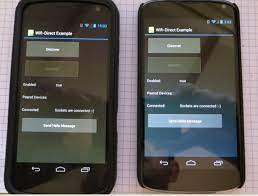
Android Wifi Communication Tutorial
Android – Wi-Fi – Tutorialspoint
Android allows applications to access to view the access the state of the wireless connections at very low level. Application can access almost all the information of a wifi connection.
The information that an application can access includes connected network’s link speed, IP address, negotiation state, other networks information. Applications can also scan, add, save, terminate and initiate Wi-Fi connections.
Android provides WifiManager API to manage all aspects of WIFI connectivity. We can instantiate this class by calling getSystemService method. Its syntax is given below −
WifiManager mainWifiObj;
mainWifiObj = (WifiManager) getSystemService(Context. WIFI_SERVICE);
In order to scan a list of wireless networks, you also need to register your BroadcastReceiver. It can be registered using registerReceiver method with argument of your receiver class object. Its syntax is given below −
class WifiScanReceiver extends BroadcastReceiver {
public void onReceive(Context c, Intent intent) {}}
WifiScanReceiver wifiReciever = new WifiScanReceiver();
registerReceiver(wifiReciever, new IntentFilter(AN_RESULTS_AVAILABLE_ACTION));
The wifi scan can be start by calling the startScan method of the WifiManager class. This method returns a list of ScanResult objects. You can access any object by calling the get method of list. Its syntax is given below −
List
String data = (0). toString();
Apart from just Scanning, you can have more control over your WIFI by using the methods defined in WifiManager class. They are listed as follows −
Method & Description
1
addNetwork(WifiConfiguration config)
This method add a new network description to the set of configured networks.
2
createWifiLock(String tag)
This method creates a new WifiLock.
3
disconnect()
This method disassociate from the currently active access point.
4
enableNetwork(int netId, boolean disableOthers)
This method allow a previously configured network to be associated with.
5
getWifiState()
This method gets the Wi-Fi enabled state
6
isWifiEnabled()
This method return whether Wi-Fi is enabled or disabled.
7
setWifiEnabled(boolean enabled)
This method enable or disable Wi-Fi.
8
updateNetwork(WifiConfiguration config)
This method update the network description of an existing configured network.
Example
Here is an example demonstrating the use of WIFI. It creates a basic application that open your wifi and close your wifi
To experiment with this example, you need to run this on an actual device on which wifi is turned on.
Steps
Description
You will use Android studio to create an Android application under a package application.
Modify src/ file to add WebView code.
Modify the res/layout/activity_main to add respective XML components
Modify the to add the necessary permissions
Run the application and choose a running android device and install the application on it and verify the results.
Following is the content of the modified main activity file src/
package application;
import;
import ntext;
public class MainActivity extends Activity {
Button enableButton, disableButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super. onCreate(savedInstanceState);
setContentView();
enableButton=(Button)findViewById();
disableButton=(Button)findViewById();
tOnClickListener(new OnClickListener(){
public void onClick(View v){
WifiManager wifi = (WifiManager) getSystemService(Context. WIFI_SERVICE);
tWifiEnabled(true);}});
tWifiEnabled(false);}});}}
Following is the modified content of the xml res/layout/
xml version="1. 0" encoding="utf-8"? >
android_id=”@+id/button2″
android_text=”Disable Wifi”
android_layout_marginBottom=”93dp”
android_layout_alignParentBottom=”true”
android_layout_alignStart=”@+id/imageView” />
Following is the content of file.
Let’s try to run your application. I assume you have connected your actual Android Mobile device with your computer. To run the app from Android studio, open one of your project’s activity files and click Run icon from the toolbar. Before starting your application, Android studio will display following window to select an option where you want to run your Android application.
Select your mobile device as an option and It will shows the following image−
Now click on disable wifi the sample output should be like this −
Create P2P connections with Wi-Fi Direct | Android Developers
Create P2P connections with Wi-Fi Direct | Android Developers
Platform
Android Studio
Google Play
Jetpack
Kotlin
Docs
Overview
Guides
Reference
Samples
Design & Quality
Games
Wi-Fi Direct (also known as peer-to-peer or P2P) allows your application to quickly find and interact with nearby
devices, at a range beyond the capabilities of Bluetooth.
The Wi-Fi peer-to-peer (P2P) APIs allow applications to connect to nearby devices without
needing to connect to a network or hotspot.
If your app is designed to be a part of a secure, near-range network, Wi-Fi
Direct is a more suitable option than traditional Wi-Fi ad-hoc
networking for the following reasons:
Wi-Fi Direct supports WPA2 encryption. (Some ad-hoc networks support only
WEP encryption. )
Devices can broadcast the services that they provide, which helps other
devices discover suitable peers more easily.
When determining which device should be the group owner for the network,
Wi-Fi Direct examines each device’s power management, UI, and service
capabilities and uses this information to choose the device that can handle
server responsibilities most effectively.
Android doesn’t support Wi-Fi ad-hoc mode.
This lesson shows you how to find and connect to nearby devices using Wi-Fi P2P.
Set up application permissions
In order to use Wi-Fi P2P, add the
ACCESS_FINE_LOCATION,
CHANGE_WIFI_STATE,
ACCESS_WIFI_STATE, and
INTERNET permissions to your manifest. Wi-Fi
P2P doesn’t require an internet connection, but it does use standard Java
sockets, which requires the INTERNET
permission. So you need the following permissions to use Wi-Fi P2P:
android_name=”CESS_WIFI_STATE”/>
android_name=”ANGE_WIFI_STATE”/>
android_name=”TERNET”/>…
Besides the preceding permissions, the following APIs also require Location Mode to be enabled:
discoverPeers
discoverServices
requestPeers
Set up a broadcast receiver and peer-to-peer manager
To use Wi-Fi P2P, you need to listen for broadcast intents that tell your
application when certain events have occurred. In your application, instantiate
an IntentFilter and set it to listen for the following:
WIFI_P2P_STATE_CHANGED_ACTION
Indicates whether Wi-Fi P2P is enabled
WIFI_P2P_PEERS_CHANGED_ACTION
Indicates that the available peer list has changed.
WIFI_P2P_CONNECTION_CHANGED_ACTION
Indicates the state of Wi-Fi P2P connectivity has changed. Starting with
Android 10, this is not sticky. If your app has relied on receiving these
broadcasts at registration because they had been sticky, use the appropriate get
method at initialization to obtain the information instead.
WIFI_P2P_THIS_DEVICE_CHANGED_ACTION
Indicates this device’s configuration details have changed. Starting with
private val intentFilter = IntentFilter()…
override fun onCreate(savedInstanceState: Bundle? ) {
super. onCreate(savedInstanceState)
setContentView()
// Indicates a change in the Wi-Fi P2P status.
dAction(WifiP2pManager. WIFI_P2P_STATE_CHANGED_ACTION)
// Indicates a change in the list of available peers.
dAction(WifiP2pManager. WIFI_P2P_PEERS_CHANGED_ACTION)
// Indicates the state of Wi-Fi P2P connectivity has changed.
dAction(WifiP2pManager. WIFI_P2P_CONNECTION_CHANGED_ACTION)
// Indicates this device’s details have changed.
dAction(WifiP2pManager. WIFI_P2P_THIS_DEVICE_CHANGED_ACTION)… }
Java
private final IntentFilter intentFilter = new IntentFilter();…
@Override
public void onCreate(Bundle savedInstanceState) {
super. onCreate(savedInstanceState);
setContentView();
dAction(WifiP2pManager. WIFI_P2P_STATE_CHANGED_ACTION);
dAction(WifiP2pManager. WIFI_P2P_PEERS_CHANGED_ACTION);
dAction(WifiP2pManager. WIFI_P2P_CONNECTION_CHANGED_ACTION);
dAction(WifiP2pManager. WIFI_P2P_THIS_DEVICE_CHANGED_ACTION);… }
At the end of the onCreate() method, get an instance of the WifiP2pManager, and call its initialize()
method. This method returns a annel object, which you’ll use later to
connect your app to the Wi-Fi P2P framework.
private lateinit var channel: annel
private lateinit var manager: WifiP2pManager
override fun onCreate(savedInstanceState: Bundle? ) {…
manager = getSystemService(Context. WIFI_P2P_SERVICE) as WifiP2pManager
channel = itialize(this, mainLooper, null)}
Channel channel;
WifiP2pManager manager;
public void onCreate(Bundle savedInstanceState) {…
manager = (WifiP2pManager) getSystemService(Context. WIFI_P2P_SERVICE);
channel = itialize(this, getMainLooper(), null);}
Now create a new BroadcastReceiver class that you’ll use to listen for changes
to the System’s Wi-Fi P2P state. In the onReceive()
method, add a condition to handle each P2P state change listed above.
override fun onReceive(context: Context, intent: Intent) {
when() {
WifiP2pManager. WIFI_P2P_STATE_CHANGED_ACTION -> {
// Determine if Wifi P2P mode is enabled or not, alert
// the Activity.
val state = tIntExtra(WifiP2pManager. EXTRA_WIFI_STATE, -1)
WifiP2pEnabled = state == WifiP2pManager. WIFI_P2P_STATE_ENABLED}
WifiP2pManager. WIFI_P2P_PEERS_CHANGED_ACTION -> {
// The peer list has changed! We should probably do something about
// that. }
WifiP2pManager. WIFI_P2P_CONNECTION_CHANGED_ACTION -> {
// Connection state changed! We should probably do something about
WifiP2pManager. WIFI_P2P_THIS_DEVICE_CHANGED_ACTION -> {
(ndFragmentById() as DeviceListFragment)
{
updateThisDevice(
tParcelableExtra(
WifiP2pManager. EXTRA_WIFI_P2P_DEVICE) as WifiP2pDevice)}}}}
public void onReceive(Context context, Intent intent) {
String action = tAction();
if ((action)) {
int state = tIntExtra(WifiP2pManager. EXTRA_WIFI_STATE, -1);
if (state == WifiP2pManager. WIFI_P2P_STATE_ENABLED) {
tIsWifiP2pEnabled(true);} else {
tIsWifiP2pEnabled(false);}} else if ((action)) {
// that. } else if ((action)) {
DeviceListFragment fragment = (DeviceListFragment) tFragmentManager(). findFragmentById();
fragment. updateThisDevice((WifiP2pDevice) tParcelableExtra(
WifiP2pManager. EXTRA_WIFI_P2P_DEVICE));}}
Finally, add code to register the intent filter and broadcast receiver when
your main activity is active, and unregister them when the activity is paused.
The best place to do this is the onResume() and
onPause() methods.
/** register the BroadcastReceiver with the intent values to be matched */
public override fun onResume() {
super. onResume()
receiver = WiFiDirectBroadcastReceiver(manager, channel, this)
registerReceiver(receiver, intentFilter)}
public override fun onPause() {
super. onPause()
unregisterReceiver(receiver)}
public void onResume() {
super. onResume();
receiver = new WiFiDirectBroadcastReceiver(manager, channel, this);
registerReceiver(receiver, intentFilter);}
public void onPause() {
super. onPause();
unregisterReceiver(receiver);}
Initiate peer discovery
To start searching for nearby devices with Wi-Fi P2P, call discoverPeers(). This method takes the
following arguments:
The annel you
received back when you initialized the peer-to-peer mManager
An implementation of tionListener with methods
the system invokes for successful and unsuccessful discovery.
Peers(channel, object: tionListener {
override fun onSuccess() {
// Code for when the discovery initiation is successful goes here.
// No services have actually been discovered yet, so this method
// can often be left blank. Code for peer discovery goes in the
// onReceive method, detailed below. }
override fun onFailure(reasonCode: Int) {
// Code for when the discovery initiation fails goes here.
// Alert the user that something went wrong. }})
Peers(channel, new tionListener() {
public void onSuccess() {
public void onFailure(int reasonCode) {
// Alert the user that something went wrong. }});
Keep in mind that this only initiates peer discovery. The
discoverPeers() method starts the discovery process and then
immediately returns. The system notifies you if the peer discovery process is
successfully initiated by calling methods in the provided action listener.
Also, discovery remains active until a connection is initiated or a P2P group is
formed.
Fetch the list of peers
Now write the code that fetches and processes the list of peers. First
implement the erListListener
interface, which provides information about the peers that Wi-Fi P2P has
detected. This information also allows your app to determine when peers join or
leave the network. The following code snippet illustrates these operations
related to peers:
private val peers = mutableListOf
private val peerListListener = erListListener { peerList ->
val refreshedPeers = viceList
if (refreshedPeers! = peers) {
()
(refreshedPeers)
// If an AdapterView is backed by this data, notify it
// of the change. For instance, if you have a ListView of
// available peers, trigger an update.
(listAdapter as WiFiPeerListAdapter). notifyDataSetChanged()
// Perform any other updates needed based on the new list of
// peers connected to the Wi-Fi P2P network. }
if (Empty()) {
Log. d(TAG, “No devices found”)
return@PeerListListener}}
private List
private PeerListListener peerListListener = new PeerListListener() {
public void onPeersAvailable(WifiP2pDeviceList peerList) {
List
if (! (peers)) {
();
(refreshedPeers);
((WiFiPeerListAdapter) getListAdapter()). notifyDataSetChanged();
if (() == 0) {
Log. d(, “No devices found”);
return;}}}
Now modify your broadcast receiver’s onReceive()
method to call requestPeers() when an intent with the action WIFI_P2P_PEERS_CHANGED_ACTION is received. You
need to pass this listener into the receiver somehow. One way is to send it
as an argument to the broadcast receiver’s constructor.
fun onReceive(context: Context, intent: Intent) {
when () {…
// Request available peers from the wifi p2p manager. This is an
// asynchronous call and the calling activity is notified with a
// callback on PeerListListener. onPeersAvailable()
mManager?. requestPeers(channel, peerListListener)
Log. d(TAG, “P2P peers changed”)}… }}
public void onReceive(Context context, Intent intent) {…
else if ((action)) {
if (mManager! = null) {
questPeers(channel, peerListListener);}
Log. d(, “P2P peers changed”);}… }
Now, an intent with the action WIFI_P2P_PEERS_CHANGED_ACTION intent
triggers a request for an updated peer list.
Connect to a peer
In order to connect to a peer, create a new WifiP2pConfig object, and copy data into it from the
WifiP2pDevice representing the device you want to
connect to. Then call the connect()
method.
override fun connect() {
// Picking the first device found on the network.
val device = peers[0]
val config = WifiP2pConfig() {
deviceAddress = viceAddress
=}
nnect(channel, config, object: tionListener {
// WiFiDirectBroadcastReceiver notifies us. Ignore for now. }
override fun onFailure(reason: Int) {
keText(
this@WiFiDirectActivity,
“Connect failed. Retry. “,
Toast. LENGTH_SHORT)()}})}
public void connect() {
WifiP2pDevice device = (0);
WifiP2pConfig config = new WifiP2pConfig();
viceAddress = viceAddress;
=;
nnect(channel, config, new ActionListener() {
public void onFailure(int reason) {
keText(, “Connect failed. LENGTH_SHORT)();}});}
If each of the devices in your group supports Wi-Fi direct, you don’t need
to explicitly ask for the group’s password when connecting. To allow a device
that doesn’t support Wi-Fi Direct to join a group, however, you need to
retrieve this password by calling
requestGroupInfo(), as
shown in the following code snippet:
questGroupInfo(channel) { group ->
val groupPassword = ssphrase}
questGroupInfo(channel, new GroupInfoListener() {
public void onGroupInfoAvailable(WifiP2pGroup group) {
String groupPassword = tPassphrase();}});
Note that the tionListener implemented in
the connect() method only notifies you when the initiation
succeeds or fails. To listen for changes in connection state, implement the
nnectionInfoListener interface.
Its onConnectionInfoAvailable() callback notifies you when the state of the
connection changes. In cases where multiple devices are going to be connected to
a single device (like a game with three or more players, or a chat app), one device
is designated the “group owner”. You can designate a particular device as
the network’s group owner by following the steps in the
Create a Group section.
private val connectionListener = nnectionInfoListener { info ->
// String from WifiP2pInfo struct
val groupOwnerAddress: String = Address
// After the group negotiation, we can determine the group owner
// (server).
if (oupFormed && GroupOwner) {
// Do whatever tasks are specific to the group owner.
// One common case is creating a group owner thread and accepting
// incoming connections. } else if (oupFormed) {
// The other device acts as the peer (client). In this case,
// you’ll want to create a peer thread that connects
// to the group owner. }}
public void onConnectionInfoAvailable(final WifiP2pInfo info) {
String groupOwnerAddress = tHostAddress();
Now go back to the onReceive() method of the broadcast receiver, and modify the section
that listens for a WIFI_P2P_CONNECTION_CHANGED_ACTION intent.
When this intent is received, call requestConnectionInfo(). This is an asynchronous call,
so results are received by the connection info listener you provide as a
parameter.
// that.
mManager? { manager ->
val networkInfo: NetworkInfo? = intent. getParcelableExtra(WifiP2pManager. EXTRA_NETWORK_INFO) as NetworkInfo
if (networkInfo?. isConnected == true) {
// We are connected with the other device, request connection
// info to find group owner IP
questConnectionInfo(channel, connectionListener)}}}… }
Java… } else if ((action)) {
if (manager == null) {
return;}
NetworkInfo networkInfo = (NetworkInfo) intent. EXTRA_NETWORK_INFO);
if (Connected()) {
questConnectionInfo(channel, connectionListener);}…
Create a group
If you want the device running your app to serve as the group owner for a
network that includes legacy devices—that is, devices that don’t support
Wi-Fi Direct—you follow the same sequence of steps as in the
Connect to a Peer section, except you create a new
tionListener
using createGroup() instead of connect(). The callback handling within the
is the same, as shown in the following code snippet:
eateGroup(channel, object: tionListener {
// Device is ready to accept incoming connections from peers. }
“P2P group creation failed. LENGTH_SHORT)()}})
eateGroup(channel, new tionListener() {
keText(, “P2P group creation failed. LENGTH_SHORT)();}});
Note: If all the devices in a network support Wi-Fi
Direct, you can use the connect() method on each device because the
method then creates the group and selects a group owner automatically.
After you create a group, you can call
requestGroupInfo() to retrieve details about the peers on
the network, including device names and connection statuses.
Content and code samples on this page are subject to the licenses described in the Content License. Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2021-10-04 UTC.
[{
“type”: “thumb-down”,
“id”: “missingTheInformationINeed”,
“label”:”Missing the information I need”}, {
“id”: “tooComplicatedTooManySteps”,
“label”:”Too complicated / too many steps”}, {
“id”: “outOfDate”,
“label”:”Out of date”}, {
“id”: “samplesCodeIssue”,
“label”:”Samples / code issue”}, {
“id”: “otherDown”,
“label”:”Other”}]
“type”: “thumb-up”,
“id”: “easyToUnderstand”,
“label”:”Easy to understand”}, {
“id”: “solvedMyProblem”,
“label”:”Solved my problem”}, {
“id”: “otherUp”,
“label”:”Other”}]
Android WiFi with Examples – Tutlane
In android, Wi-Fi is a wireless network protocol that allows devices to connect to the internet or connect wirelessly with other devices to exchange the data.
Generally, in android applications by using Wi-Fi API’s we can manage all aspects of WI-FI connectivity, such as a scan or search for available networks, add/save/delete Wi-Fi connections and managing the data transfer between devices within the range.
By using android Wi-Fi API’s in android applications, we can perform following functionalities.
Scan for the available Wi-Fi networks within the range
Allow devices to connect to the internet
Connect to other devices through service discovery
Manage list of configured networks.
Manage multiple connections
Android Set Wi-Fi Permissions
To use Wi-Fi features in our android applications, we must need to add multiple permissions, such as CHANGE_WIFI_STATE, ACCESS_WIFI_STATE and INTERNET in our manifest file.
Following is the example of defining the Bluetooth permissions in android manifest file.
Android WifiManager Class
In android, we can perform Wi-Fi related activities by using WifiManager class in our applications. This class will provide required API’s to manage all aspects of Wi-Fi connectivity.
By using WifiManager class, we can perform the operations that are related to network connectivity. We can instantiate this class by using tSystemService(Class) with the argument or tSystemService(String) with the argument Context. WIFI_SERVICE.
Following is the code snippet to initialize WifiManager class using tSystemService(String) with the argument Context. WIFI_SERVICE.
WifiManager wmgr = (WifiManager)tSystemService(Context. WIFI_SERVICE);
If you observe above code snippet, we used getSystemService() method to instantiate a WifiManager class.
In case if getSystemService() method returns NULL, then the device does not support Wi-Fi and we can disable all Wi-Fi features.
Android Enable or Disable Wi-Fi
If Wi-Fi is supported but disabled, then isWifiEnabled() method will return false and we can request user to enable wifi without leaving our application by using setWifiEnabled method.
Following is the code snippet to enable a Wi-Fi in android application by using setWifiEnabled() method.
WifiManager wmgr = (WifiManager)tSystemService(Context. WIFI_SERVICE); tWifiEnabled(true);
If you observe above code snippet, we used setWifiEnabled(true) method to turn ON or Enable a Wi-Fi in our android application.
By using setWifiEnabled(false) method we can Disable or turn OFF a Wi-Fi in android applications.
Android List Wireless Networks
By using WifiManager method getScanResults(), we can get the list of available Wi-Fi network details.
Following is the code snippet to get the available Wi-Fi network details in android applications.
WifiManager wmgr = (WifiManager)getApplicationContext(). getSystemService(Context. WIFI_SERVICE); // Get List of Available Wifi Networks List
Now open your main activity file from \java\com. tutlane. wifiexample path and write the code like as shown below
package com. wifiexample; import ntext; import; import; import; import; import;public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super. onCreate(savedInstanceState); setContentView(); Button btntOn = (Button)findViewById(); Button btntOff = (Button)findViewById(); tOnClickListener(new View. OnClickListener() { @Override public void onClick(View v) { WifiManager wmgr = (WifiManager)getApplicationContext(). WIFI_SERVICE); tWifiEnabled(true);}}); tOnClickListener(new View. WIFI_SERVICE); tWifiEnabled(false);}});}}
If you observe above code, we used setWifiEnabled() method of WifiManager class to enable or disable a Wi-Fi in our application.
As discussed, we need to set Wi-Fi permissions in android manifest file () to access Wi-Fi features in android applications. Now open android manifest file () and write the code like as shown below
xml version="1. 0" encoding="utf-8"? >
If you observe above code, we added required Wi-Fi permissions in manifest file to access Wi-Fi features in android applications.
Output of Android Wi-Fi Turn ON / OFF Example
When we run the above program in the android studio we will get the result as shown below.
When we click on Turn ON button, the device Wi-Fi gets switched ON and when we click on Turn OFF button, the device Wi-Fi get switched OFF.
This is how we can turn ON or OFF Wi-Fi in android applications based on our requirements.