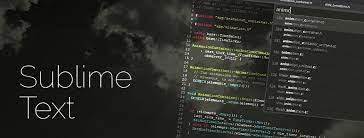
Selenium Javascript Find Element
Find Element and FindElements in Selenium : Differences
Finding elements on the webpage can be a nightmare due to the complexity of web elements. However, web elements play a vital role in developing and testing any application. To make things easier for testers, this article will offer some insight into Selenium findElement and findElements with the help of an ’s what the article will cover:Introduction to Selenium findElementDifference between findElement and findElementsSyntax of Selenium findElementTypes of locatorsUsing locator Strategy to findElementIntroduction to Selenium findElementInteraction with a web page requires the driver to locate a web element and either trigger a JavaScript event like a click, enter, select, etc. or type in the field value. Usually, one starts automated testing of any web-app with finding relevant web elements on the web page. Most often, such automated testing is executed by using frameworks like Selenium lenium defines two methods for identifying web elements:findElement: A command used to uniquely identify a web element within the web ndElements: A command used to identify a list of web elements within the web ’s understand the difference between these two methods in greater detail. Difference between findElement and findElementsfindElementfindElementsReturns the first matching web element if multiple web elements are discovered by the locatorReturns a list of multiple matching web elementsThrows NoSuchElementException if the element is not foundReturns an empty list if no matching element is foundDetects a unique web elementReturns a collection of matching elementsTo understand some fundamental Selenium commands, take a look at Basic Commands of Selenium of Selenium findElementThe findElement command returns an object of type WebElement. It can use various locator strategies such as ID, Name, ClassName, link text, XPath, is the syntax:WebElement elementName = ndElement(By. LocatorStrategy(“LocatorValue”));Locator Strategy comprises the following NameClass NameTag NameLink TextXPathLocator Value is the unique method with which one can easily identify the web ndElement((“//input[@id=’gh-ac’]”)). sendKeys(“Guitar”);Syntax of Selenium findElementsThe findElements command returns an empty list if there are no elements found using the given locator strategy and locator value. Below is the syntax of findElements
driver = (‘. /chromedriver’)
#instance of Chrome | Firefox | IE driver
(
elementID = ndElement((“twotabsearchtextbox”))
# will raise NoSuchElementException if not found2. Find by NameThis is similar to Find By ID except the driver will locate an element by “name” attribute instead of “id”. Example: Consider the same amazon webpage. The search box also has a name locator whose value is “field-keywords” as shown in the snapshot entire code remains similar to that of ID locator, except that the difference will be in the findElement syntax. elementName = ndElement((“field-keywords”))3. Find By LinkTextLinkText is helpful to find links in a webpage. It is the most efficient way of finding web elements containing, locate a Returns link as shown the tester hits that link and inspect, it locates the link text Returns as shown in the snapshot can be located simply by using Selenium findElement with the syntax below:elementLinktext = ndElement(nkText(“Returns”))4. Find By CSS SelectorCSS Selector is used to provide style rules for web pages and also can be used to identify one or more web elements. Example: Consider the same search box of the Amazon page. It has a class attribute whose value is nav-search-input. Using the same value, the Selenium findElement method can locate the element. elementcss= ndElement(By. cssSelector(”))5. Find By XPathXPath is a technique in Selenium to navigate through the HTML structure of a page. XPath enables testers to navigate through the XML structure of any document and can be used on both HTML and XML documents. Example: Let’s try to locate the same search bar in Amazon with the help of XPath. The code below shows that it contains an ID locator. Note that relative XPath starts with ‘//’ the image below, one can see an input tag. Start with //input. Here //input implies a tag name. Make use of the id attribute and pass twotabsearchtextbox in single quotes as its value. This will give the XPath expression shown belowinput[@id=’twotabsearchtextbox’]
Now, using Selenium findElement method, execute the code below:elementxpath = ndElement((“//input[@id=’twotabsearchtextbox’]”))Run Selenium Tests for Free on Real DevicesLocating web elements is at the basis of automated Selenium testing of websites and web-apps. To know more about the fundamentals of Selenium, take a look at Selenium WebDriver Tutorial.
How To Find Elements In A Webpage Using JavaScript – C# …
In this article, will discuss about finding an element using Javascript.
In my last article I have explained about JavascriptExecutor and covered the below points:
What is JavascriptExecutor?
Why do we need JavascriptExecutor?
How to use JavascriptExecutor with an example?
What Is Javascript Executor In Selenium WebDriver?
As I have mentioned in my above article, JavascriptExecutor is used for performing Javascript operations in a web browser from selenium webdriver.
The javascript operations can be performed on two things,
Web Elements
Web Page
So to perform any operations on web elements, first of all, we need to find the elements in the browser.
We all know how to find the elements using selenium web driver, if you don’t know please follow the below article:
Overview Of Selenium Locators
Now we will learn “how to find the elements using javascript”.
Just like in selenium web driver, javascript also provides some methods to find the elements:
getElementById
getElementsByName
getElementsByClass
getElementsByTagName
Here if you observe carefully, we have only one single method(getElementById) which returns the webelement and the rest are returning the list of webelements.
All these methods are available on the document.
In selenium web driver, we try to find the element on driver instance because the driver instance is holding the browser instance but in javascript, we are finding the elements on the document because here we are querying the DOM (Document object model).
Here I’m using below HTML code to demonstrate these Javascript element finding methods,
This method queries the document to get the element based on its id attribute value.
Syntax
tElementById(<
Parameters
id – The id attribute value of an element.
Return value – if the element is found then it returns the element, if the element is not found then it returns null.
In the above HTML code snippet, we have an ID attribute for the first textbox so we use that id value to get the element.
Usage in Selenium code
String javascript = “tElementById(‘username’)”;
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
WebElement element = (WebElement) jsExecutor. executeScript(javascript);
Generally, this is the only method that returns a single element because in a web application the id attribute values are the most unique values.
This method queries the document to get the element(s) based on their name attribute value.
tElementsByName(<
name – The name attribute value of an element(s).
Return value – If the element(s) are found then it returns the NodeList (Collection) of element(s), if the element(s) are not found then it returns an empty NodeList.
In the above HTML code snippet, we have a name attribute for the second textbox so we use that name attribute value to get the element.
Using this method if you want to get only one element just like in selenium web driver(ndElement((<
tElementsByName(<
String javascript = “tElementsByName(‘password’)[0]”;
WebElement element = (WebElement) jsExecutor. executeScript(javascript);
getElementsByClassName
This method queries the document to get the element(s) based on their class attribute value.
tElementsByClassName(<
className – The className attribute value of an element(s).
Return value – if the element(s) are found then it returns the NodeList (Collection) of element(s), if the element(s) are not found then it returns an empty NodeList.
In the above HTML code snippet, we have a class attribute for the third textbox so we use that class attribute value to get the element.
Using this method if you want to get only one element just like in selenium webdriver(ndElement(assName(<
tElementsByClassName(<
String javascript = “tElementsByClassName(‘loginForm’)[0]”;
This method queries the document to get the element(s) based on their tagname.
tElementsByTagName(<
tagName – The tagName of an element(s).
In the above HTML code snippet, we have a button element whose tageName is button. So we use that tagName to get the element.
Using this method if you want to get only one element just like in selenium webdriver(ndElement(By. TagName(<
tElementsByTagName(<
Usage in Selenium code,
String javascript = “tElementsByTagName(‘button’)[0]”;
Here you might be thinking somehting like, what if my target element is not having any of these attributes, in that case, how can I find the element(s)?
In the selenium web driver, we have the other ways to find the element(s) like XPath and CSS selector.
If you want to find the element in javascript also using XPath and CSS selector, we have a way to do that.
But we are not able to find those methods along with the above-mentioned methods right?.
That is because those methods names are quite different here in javascript, those are:
querySelector
evaluate
This method is used for finding an element using a CSS selector.
document. querySelector(<
cssSelector – The cssSelector expression to find the target element.
In the above HTML code snippet, we have a link that doesn’t have any attribute values like id, name and className. so we use that tagName to write the CSS selector.
String javascript = “document. querySelector(‘a’)”;
This method is used for finding an element using XPath.
document. evaluate(xpathExpression, contextNode, namespaceResolver, resultType, result). singleNodeValue;
xpathExpression – The XPath expression to find the target element.
contextNode – From which context you want to start the search.
namespaceResolver – This is used when handing with XML documents, so for HTML we can give null.
resultType – It is an integer that corresponds to the type of result XPathResult to return.
result – If you have already defined a variable for storing XPathResult, we can use that here or you can pass null (It will create a new XPathResult)
Return value – If the element is found then it returns the element, if the element is not found then it returns null.
In the above HTML code snippet, we have a link that doesn’t have any attribute values like id, name and className. So we use that link’s text to write the XPath.
String javascript = “document. evaluate(‘
Here in every code, I have written the below line:
WebElement element = (WebElement) jsExecutor. executeScript(javascript);
By default, the executeScript method will return object because in HTML DOM every element is treated as an object only.
To store that object instance into WebElement, I have typecast the object into WebElement.
So this is how we have to find the elements in a webpage using javascript.
In my next articles, we will see how we can perform click operation and enter text into a textbox, etc…
I hope you find this article useful. Please provide your valuable feedback, questions, or comments about this article.
Locating elements | Selenium
Locating one elementOne of the most fundamental techniques to learn when using WebDriver is
how to find elements on the page. WebDriver offers a number of built-in selector
types, amongst them finding an element by its ID attribute:JavaPythonCSharpRubyJavaScriptKotlinWebElement cheese = ndElement((“cheese”));
nd_element(, “cheese”)
IWebElement element = ndElement((“cheese”));
cheese = nd_element(id: ‘cheese’)
const cheese = ndElement((‘cheese’));
val cheese: WebElement = ndElement((“cheese”))
As seen in the example, locating elements in WebDriver is done on the
WebDriver instance object. The findElement(By) method returns
another fundamental object type, the WebElement. WebDriver represents the browserWebElement represents a particular DOM node
(a control, e. g. a link or input field, etc. )Once you have a reference to a web element that’s been “found”,
you can narrow the scope of your search
by using the same call on that object instance:JavaPythonCSharpRubyJavaScriptKotlinWebElement cheese = ndElement((“cheese”));
WebElement cheddar = ndElement((“cheddar”));
cheese = nd_element(, “cheese”)
cheddar = nd_elements_by_id(“cheddar”)
IWebElement cheese = ndElement((“cheese”));
IWebElement cheddar = ndElement((“cheddar”));
cheddar = nd_element(id: ‘cheddar’)
const cheddar = ndElement((‘cheddar’));
val cheese = ndElement((“cheese”))
val cheddar = ndElement((“cheddar”))
You can do this because both the WebDriver and WebElement types
implement the SearchContext
interface. In WebDriver, this is known as a role-based interface.
Role-based interfaces allow you to determine whether a particular
driver implementation supports a given feature. These interfaces are
clearly defined and try to adhere to having only a single role of
responsibility. You can read more about WebDriver’s design and what
roles are supported in which drivers in the Some Other Section Which
Must Be nsequently, the By interface used above also supports a
number of additional locator strategies. A nested lookup might not be
the most effective cheese location strategy since it requires two
separate commands to be issued to the browser; first searching the DOM
for an element with ID “cheese”, then a search for “cheddar” in a
narrowed improve the performance slightly, we should try to use a more
specific locator: WebDriver supports looking up elements
by CSS locators, allowing us to combine the two previous locators into
one ndElement(By. cssSelector(“#cheese #cheddar”));
cheddar = nd_element_by_css_selector(“#cheese #cheddar”)
ndElement(By. CssSelector(“#cheese #cheddar”));
nd_element(css: ‘#cheese #cheddar’)
const cheddar = ndElement((‘#cheese #cheddar’));
ndElement(By. cssSelector(“#cheese #cheddar”))
Locating multiple elementsIt is possible that the document we are working with may turn out to have an
ordered list of the cheese we like the best:
- …
- …
- …
- …
Since more cheese is undisputably better, and it would be cumbersome
to have to retrieve each of the items individually, a superior
technique for retrieving cheese is to make use of the pluralized
version findElements(By). This method returns a collection of web
elements. If only one element is found, it will still return a
collection (of one element). If no element matches the locator, an
empty list will be PythonCSharpRubyJavaScriptKotlinList
mucho_cheese = nd_elements_by_css_selector(“#cheese li”)
IReadOnlyList
mucho_cheese = nd_elements(css: ‘#cheese li’)
const muchoCheese = ndElements((‘#cheese li’));
val muchoCheese: List
Element selection strategiesThere are eight different built-in element location strategies in WebDriver:LocatorDescriptionclass nameLocates elements whose class name contains the search value (compound class names are not permitted)css selectorLocates elements matching a CSS selectoridLocates elements whose ID attribute matches the search valuenameLocates elements whose NAME attribute matches the search valuelink textLocates anchor elements whose visible text matches the search valuepartial link textLocates anchor elements whose visible text contains the search value. If multiple elements are matching, only the first one will be nameLocates elements whose tag name matches the search valuexpathLocates elements matching an XPath expressionTips on using selectorsIn general, if HTML IDs are available, unique, and consistently
predictable, they are the preferred method for locating an element on
a page. They tend to work very quickly, and forego much processing
that comes with complicated DOM unique IDs are unavailable, a well-written CSS selector is the
preferred method of locating an element. XPath works as well as CSS
selectors, but the syntax is complicated and frequently difficult to
debug. Though XPath selectors are very flexible, they are typically
not performance tested by browser vendors and tend to be quite lection strategies based on linkText and partialLinkText have
drawbacks in that they only work on link elements. Additionally, they
call down to XPath selectors internally in name can be a dangerous way to locate elements. There are
frequently multiple elements of the same tag present on the page.
This is mostly useful when calling the findElements(By) method which
returns a collection of recommendation is to keep your locators as compact and
readable as possible. Asking WebDriver to traverse the DOM structure
is an expensive operation, and the more you can narrow the scope of
your search, the lative LocatorsSelenium 4 brings Relative Locators which are previously
called as Friendly Locators. This functionality was
added to help you locate elements that are nearby other elements.
The Available Relative Locators are:abovebelowtoLeftOftoRightOfnearThe findElement method accepts a new method with(By) which returns
a RelativeLocator. Users can pick a locator of their choice like, By. cssSelector, does it workSelenium uses the JavaScript function
getBoundingClientRect()
to find the relative elements. This function returns
properties of an element such as
right, left, bottom, and us consider the below example for understanding the relative ()Returns the WebElement, which appears
above to the specified elementJavaPythonCSharpRubyJavaScriptKotlinimport static;
WebElement passwordField = ndElement((“password”));
WebElement emailAddressField = ndElement(with(By. tagName(“input”))
(passwordField));from import By
from lative_locator import locate_with
passwordField = nd_element(, “password”)
emailAddressField = nd_element(locate_with(By. TAG_NAME, “input”)(passwordField))using static lativeBy;
IWebElement passwordField = ndElement((“password”));
IWebElement emailAddressField = ndElement(RelativeBy(By. TagName(“input”))(passwordField));password_field= nd_element(:id, “password”)
email_address_field = nd_element(relative: {tag_name: ‘input’, above:password_field})let passwordField = ndElement((‘password’));
let emailAddressField = await ndElement(locateWith(By. tagName(‘input’))(passwordField));val passwordField = ndElement((“password”))
val emailAddressField = ndElement(with(By. tagName(“input”))(passwordField))below()Returns the WebElement, which appears
below to the specified elementJavaPythonCSharpRubyJavaScriptKotlinimport static;
WebElement emailAddressField = ndElement((“email”));
WebElement passwordField = ndElement(with(By. tagName(“input”))
(emailAddressField));from import By
emailAddressField = nd_element(, “email”)
passwordField = nd_element(locate_with(By. TAG_NAME, “input”)(emailAddressField))using static lativeBy;
IWebElement emailAddressField = ndElement((“email”));
IWebElement passwordField = ndElement(RelativeBy(By. TagName(“input”))(emailAddressField));email_address_field = nd_element(:id, “email”)
password_field = nd_element(relative: {tag_name: ‘input’, below: email_address_field})let emailAddressField = ndElement((’email’));
let passwordField = await ndElement(locateWith(By. tagName(‘input’))(emailAddressField));val emailAddressField = ndElement((“email”))
val passwordField = ndElement(with(By. tagName(“input”))(emailAddressField))toLeftOf()Returns the WebElement, which appears
to left of specified elementJavaPythonCSharpRubyJavaScriptKotlinimport static;
WebElement submitButton = ndElement((“submit”));
WebElement cancelButton = ndElement(with(By. tagName(“button”)). toLeftOf(submitButton));from import By
submitButton = nd_element(, “submit”)
cancelButton = nd_element(locate_with(By. TAG_NAME, “button”).
to_left_of(submitButton))using static lativeBy;
IWebElement submitButton = ndElement((“submit”));
IWebElement cancelButton = ndElement(RelativeBy(By. TagName(“button”))(submitButton));submit_button= nd_element(:id, “submit”)
cancel_button = nd_element(relative: {tag_name: ‘button’, left:submit_button})let submitButton = ndElement((‘submit’));
let cancelButton = await ndElement(locateWith(By. tagName(‘button’)). toLeftOf(submitButton));val submitButton = ndElement((“submit”))
val cancelButton = ndElement(with(By. toLeftOf(submitButton))toRightOf()Returns the WebElement, which appears
to right of the specified elementJavaPythonCSharpRubyJavaScriptKotlinimport static;
WebElement cancelButton = ndElement((“cancel”));
WebElement submitButton = ndElement(with(By. toRightOf(cancelButton));from import By
cancelButton = nd_element(, “cancel”)
submitButton = nd_element(locate_with(By. TAG_NAME, “button”).
to_right_of(cancelButton))using static lativeBy;
IWebElement cancelButton = ndElement((“cancel”));
IWebElement submitButton = ndElement(RelativeBy(By. TagName(“button”)). RightOf(cancelButton));cancel_button = nd_element(:id, “cancel”)
submit_button = nd_element(relative: {tag_name: ‘button’, right:cancel_button})let cancelButton = ndElement((‘cancel’));
let submitButton = await ndElement(locateWith(By. toRightOf(cancelButton));val cancelButton = ndElement((“cancel”))
val submitButton = ndElement(with(By. toRightOf(cancelButton))near()Returns the WebElement, which is
at most 50px away from the specified PythonCSharpRubyJavaScriptKotlinimport static;
WebElement emailAddressLabel = ndElement((“lbl-email”));
WebElement emailAddressField = ndElement(with(By. tagName(“input”))(emailAddressLabel));from import By
emailAddressLabel = nd_element(, “lbl-email”)
emailAddressField = nd_element(locate_with(By. TAG_NAME, “input”).
near(emailAddressLabel))using static lativeBy;
IWebElement emailAddressLabel = ndElement((“lbl-email”));
IWebElement emailAddressField = ndElement(RelativeBy(By. TagName(“input”))(emailAddressLabel));email_address_label = nd_element(:id, “lbl-email”)
email_address_field = nd_element(relative: {tag_name: ‘input’, near: email_address_label})let emailAddressLabel = ndElement((“lbl-email”));
let emailAddressField = await ndElement(locateWith(By. tagName(“input”))(emailAddressLabel));val emailAddressLabel = ndElement((“lbl-email”))
val emailAddressField = ndElement(with(By. tagName(“input”))(emailAddressLabel))
Frequently Asked Questions about selenium javascript find element
How do you find an element in selenium?
The different locators in Selenium are as follows:By CSS ID: find_element_by_id.By CSS class name: find_element_by_class_name.By name attribute: find_element_by_name.By DOM structure or xpath: find_element_by_xpath.By link text: find_element_by_link_text.By partial link text: find_element_by_partial_link_text.More items…•Aug 7, 2019
How do you find an element?
Explanation: There are two properties that can be used to identify an element: the atomic number or the number of protons in an atom. The number of neutrons and number of electrons are frequently equal to the number of protons, but can vary depending on the atom in question.
Is there a way to get element by XPath using Javascript in selenium WebDriver?
To identify a WebElement using xpath and javascript you have to use the evaluate() method which evaluates an xpath expression and returns a result.Dec 12, 2013